JavaScript is the darling of web development — but that doesn’t mean it’s without its flaws.
Often, developers get so charmed by its universal acceptance and ability to provide interactive web content that, they overlook its weaknesses, such as unpredictable behavior across different browsers.
Countless hours spent in the trenches of web development have led me to a balanced perspective on JavaScript. Here’s my take:
Advantages of JavaScript Web Development
JavaScript is a versatile and widely-used programming language, particularly for web development. Here are some of its key advantages, presented in a concise table format, followed by a few code samples to illustrate these points.
Advantages of JavaScript Web Development
Advantage | Description |
---|---|
Interactivity | Enhances user interaction on web pages through dynamic content updates without reloading the page. |
Versatility | Can be used for both client-side and server-side development (thanks to Node.js). |
Rich Libraries & Frameworks | Extensive libraries and frameworks (like React, Angular, Vue) simplify complex tasks and improve UI/UX. |
High Performance | Fast execution speed, especially for client-side scripting, leading to a better user experience. |
Community Support | Large developer community providing extensive resources, support, and continuous updates. |
Cross-Platform & Cross-Browser Compatibility | Works across all major browsers and can be integrated with other languages for full-stack development. |
Easy to Learn | Relatively straightforward syntax, making it accessible for beginners. |
JavaScript Code Samples
Adding Interactive Elements:
For instance, changing the text of a button when it's clicked.
document.getElementById("myButton").addEventListener("click", function() {
this.innerHTML = "Clicked!";
});
AJAX for Dynamic Content Update:
Using AJAX to load new content without reloading the page.
function loadNewContent() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("content").innerHTML = this.responseText;
}
};
xhttp.open("GET", "newcontent.html", true);
xhttp.send();
}
Using a Framework (React Example):
A simple React component displaying a message.
class WelcomeMessage extends React.Component {
render() {
return <h1>Hello, World!</h1>;
}
}
These examples showcase how JavaScript can be used to add interactivity, perform asynchronous operations, and create web applications with modern frameworks.
Disadvantages of JavaScript Web Development
While JavaScript is widely used in web development, it has some drawbacks that developers should be aware of. These include:
- Browser Compatibility: One of the biggest challenges of using JavaScript in web development is browser compatibility. Different browsers can interpret JavaScript code differently, causing inconsistencies and errors. For example, Internet Explorer may not support certain JavaScript functions or may require specific workarounds for them to work as expected.
- Security Vulnerabilities: JavaScript can be vulnerable to security threats such as cross-site scripting (XSS) attacks, where attackers inject malicious code into web pages to steal sensitive information or execute unauthorized actions. Developers need to take extra care to write secure code and follow best practices to prevent such attacks.
- Potential for Code Complexity: As web applications grow more complex, so does the JavaScript code required to build them. This can make it more difficult to maintain and debug the codebase, reducing overall productivity. Developers need to adopt good coding practices and use tools like linters to avoid writing overly complex code.
- Performance: Heavy use of JavaScript can negatively impact website performance, especially on mobile devices with limited processing power and memory. Developers need to optimize their code to reduce load times and improve overall performance.
However, despite these challenges, JavaScript remains a popular choice among web developers due to its versatility, wide range of frameworks and libraries, and compatibility with different browsers.
By understanding the drawbacks of using JavaScript in web development, developers can take steps to mitigate these issues and build more secure and efficient web applications.
Comparison of JavaScript Frameworks
JavaScript frameworks have become an essential part of web development, offering a range of tools and libraries that make it easier for developers to create complex applications. In this section, we’ll compare popular JavaScript frameworks and highlight the pros and cons of each one.
React
React is one of the most widely used JavaScript frameworks, developed by Facebook. It uses a component-based approach for building user interfaces, allowing developers to create reusable UI elements. React has a large and active community, offering a wide range of plugins and extensions.
One of the benefits of React is its performance; it uses a virtual DOM that updates efficiently, which results in faster rendering times. React’s component structure also makes it easier to debug and maintain code.
Here’s an example of how to create a simple React component:
// Define a component
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
// Use the component
<Greeting name="World" />
Angular
Angular is a framework that emphasizes a declarative approach to building applications, allowing developers to focus on the user interface and the logic that drives it. It is used by many large-scale enterprise applications, including Google’s own products.
One of the benefits of Angular is its use of two-way data binding, which allows changes made to the model to be immediately reflected in the view. Angular also provides a comprehensive suite of tools for building complex applications, including form validation, dependency injection, and routing.
Here’s an example of how to create a simple Angular component:
// Define a component
@Component({
selector: 'hello-world',
template: '<h1>Hello, {{name}}!</h1>'
})
export class HelloWorldComponent {
name = 'World';
}
// Use the component
<hello-world />
Vue.js
Vue.js is a relatively new framework that has gained popularity for its simplicity and flexibility. It focuses on the view layer of an application, making it easy to integrate with existing projects. Vue.js is also known for its small size and fast performance.
One of the benefits of Vue.js is its intuitive syntax, which makes it easy for developers to learn and use. Vue.js also has a growing community, with many plugins and tools available to extend its functionality.
Here’s an example of how to create a simple Vue.js component:
// Define a component
Vue.component('hello-world', {
template: '<h1>Hello, {{name}}!</h1>',
data: function() {
return { name: 'World' }
}
})
// Use the component
<hello-world />
When choosing a JavaScript framework, it’s important to consider factors such as performance, community support, and ease of use. By comparing the pros and cons of each framework, developers can make informed decisions based on their project requirements.
Performance Considerations in JavaScript Web Development
When building a web application, it’s essential to consider its performance. A poorly performing web app frustrates users and can even hurt your business. Here are some key performance considerations to keep in mind when working with JavaScript:
- Optimizing Code Execution: JavaScript is a single-threaded language, which means that if one task takes a long time to execute, it can block other tasks from executing. Developers need to be careful when writing JavaScript code to avoid long-running tasks that can hinder app performance. One solution is to use asynchronous code, which allows multiple tasks to run concurrently without blocking each other.
- Reducing Load Times: A web app’s load time has a significant impact on user experience. JavaScript files can be quite large, which can increase load times. Developers can mitigate this issue by minifying and compressing their JavaScript code to reduce its file size. Additionally, loading scripts asynchronously can help improve load times by allowing the rest of the page to load while the JavaScript is still loading in the background.
- Improving Overall Website Performance: In addition to optimizing JavaScript code and reducing load times, there are other steps developers can take to improve overall website performance. Caching frequently accessed data, such as images or JavaScript libraries, can help reduce load times. Additionally, developers can use techniques such as lazy loading and code splitting to ensure that only the necessary code is loaded when a user navigates to a page.
By keeping these performance considerations in mind, developers can build fast and responsive web applications that provide a great user experience.
Accessibility and JavaScript Web Development
Accessibility is a critical consideration for web developers, as it ensures that everyone, including individuals with disabilities, can access and use a website. However, JavaScript-based interactions can pose challenges for accessibility, and developers must be aware of these challenges to create inclusive websites.
Best Practices for Accessibility
To ensure accessibility in JavaScript web development, developers must follow established best practices. These include:
- Providing text alternatives for non-text content, such as images and videos
- Ensuring that keyboard navigation is available and works seamlessly with JavaScript-based interactions
- Using semantic HTML to ensure that screen readers can accurately interpret the content
- Avoiding flashing or blinking elements that can trigger seizures or other physical reactions
Challenges with JavaScript-Based Interactions
While JavaScript can enhance the user experience, it can also create barriers for individuals with disabilities. Some of the challenges associated with JavaScript-based interactions include:
- Incompatibility with screen readers and other assistive technologies
- Complexity that can make it difficult for users to navigate or understand the content
- Slow-loading pages that can be frustrating for users and exacerbate accessibility issues
However, there are solutions that developers can implement to address these challenges. For example, developers can use ARIA (Accessible Rich Internet Applications) attributes to provide additional information about the content and its context to assistive technologies. They can also optimize code and ensure that pages load quickly to minimize frustration for users.
“Accessibility is not a feature, it is a requirement.”
As web development becomes increasingly complex, it is essential for developers to prioritize accessibility and consider how JavaScript can impact it. By following best practices and addressing the challenges associated with JavaScript-based interactions, developers can create websites that are inclusive and accessible to all users.
Javascript Web Development and SEO
Search engine optimization (SEO) is a critical consideration for web developers who want their websites to rank highly in search engine results pages (SERPs). The unique architecture of JavaScript web applications, however, can make it challenging for search engines to crawl and index content, resulting in lower search rankings.
One of the main SEO challenges of JavaScript web development is that search engines typically rely on the HTML code of a website to understand its content. JavaScript, however, often loads content dynamically, after the page has loaded, which can result in content that is not visible to search engines.
To overcome this challenge, web developers can use techniques such as server-side rendering (SSR) or client-side rendering (CSR) to ensure that content is visible to search engines. SSR involves rendering HTML on the server, while CSR relies on JavaScript to render the content on the client side. Both approaches have their advantages and disadvantages and can have an impact on website performance.
Another important consideration for JavaScript web development and SEO is website speed. Since website loading speed is one of the factors that search engines use to rank websites, it is essential to optimize JavaScript code for fast performance. This can include techniques such as code minification, lazy loading, and compression.
Despite these challenges, JavaScript web development can have significant benefits for SEO. For example, JavaScript frameworks such as React and Angular can improve website speed and user experience, resulting in lower bounce rates and higher engagement metrics, which can positively impact search rankings.
Overall, web developers should carefully consider the SEO implications of using JavaScript in web development projects. By using best practices and optimizing code for speed and performance, developers can mitigate the challenges associated with JavaScript and improve their website’s search engine visibility.
Cross-Platform Development with JavaScript
Cross-platform development enables developers to create applications that can run on multiple platforms. JavaScript has become a popular choice for cross-platform development due to its versatility and wide range of frameworks and libraries. Additionally, JavaScript allows developers to seamlessly integrate with other web technologies, such as HTML and CSS.
Several frameworks and tools are available to facilitate cross-platform development with JavaScript. React Native, for example, allows developers to create native mobile applications for both iOS and Android platforms with a single codebase. Ionic is another popular framework for developing hybrid mobile applications with JavaScript, HTML, and CSS.
Desktop applications can also be built with JavaScript using tools such as Electron and NW.js. Electron is used for creating cross-platform desktop applications with web technologies such as HTML, CSS, and JavaScript. NW.js, on the other hand, enables developers to create desktop applications using web technologies and Node.js modules.
Both frameworks offer similar functionality and structure for creating a login screen in a cross-platform mobile application. React Native uses a combination of JavaScript and JSX to define the screen layout, while Ionic uses Angular and HTML templates.
Ultimately, the choice of framework for cross-platform development with JavaScript will depend on various factors such as project requirements, development team expertise, and available resources.
Final Thoughts
Overall, JavaScript web development has its pros and cons. As we’ve discussed in this article, there are many advantages to using JavaScript, such as its versatility, compatibility with different browsers, and a wide range of frameworks and libraries.
However, there are also potential drawbacks, including challenges with browser compatibility, security vulnerabilities, and the potential for code complexity. Aspiring JavaScript web developers should carefully consider these pros and cons before making career decisions.
While it’s important to be aware of the drawbacks, we must also acknowledge the benefits of using JavaScript for web development. With the right skills and knowledge, developers can create powerful and efficient applications that perform well across different platforms and devices.
External Resources
FAQ
What are the main advantages of using JavaScript for web development?
- Answer: JavaScript’s main advantages include its ability to create interactive web pages, its support for various libraries and frameworks, and its compatibility across different browsers and platforms. It’s also versatile, as it can be used for both client-side and server-side development.
Code Sample: Adding a simple interactive click event.
document.getElementById("clickMeButton").addEventListener("click", function() {
alert("Button Clicked!");
});
Are there any performance concerns with JavaScript?
- Answer: JavaScript can sometimes lead to performance issues, especially in complex web applications. This is due to its single-threaded nature, which can cause delays if scripts are not optimized.
Code Sample: Demonstrating a potential performance bottleneck.
for (let i = 0; i < 1000000; i++) {
// Complex calculations or DOM manipulations here
}
How does JavaScript enhance user experience on websites?
- Answer: JavaScript enhances user experience by allowing for the creation of dynamic, responsive interfaces. This includes things like form validations, animations, and asynchronous content updates.
Code Sample: Asynchronous content update with Fetch API.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
document.getElementById("dataContainer").innerHTML = JSON.stringify(data);
});
Can JavaScript be used for backend development?
- Answer: Yes, JavaScript can be used for backend development, particularly with Node.js, which allows for JavaScript to be run on the server side.
Code Sample: A simple Node.js server.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
What are the limitations of JavaScript in web development?
- Answer: JavaScript’s limitations include security concerns, as client-side scripts can be viewed and altered. It’s also dependent on the user’s browser and settings; for instance, users can disable JavaScript.
Code Sample: Checking if JavaScript is enabled.
<noscript>
JavaScript is not enabled in your browser. Please enable it for the
best user experience.
</noscript>
These questions and answers provide a balanced view of JavaScript’s capabilities and limitations in web development, along with relevant code samples to illustrate these points.
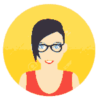
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.