Have wondered about proven JavaScript Techniques? Web application development has become an important skill in today’s technology-driven world. Businesses need dynamic and interactive applications to engage their customers and increase their reach.
Learning web app development is now more accessible than ever, and with the right techniques, it can be a straightforward process.
In this article, we introduce proven JavaScript techniques that will help you learn web app development and build applications quickly. We cover the fundamentals of web app development, getting started with JavaScript, building user interfaces with frameworks, managing data with libraries, enhancing user experience with animation and effects, implementing backend functionality with Node.js, ensuring web app security with JavaScript best practices, and optimizing web app performance with JavaScript techniques.
Key Takeaways
- Learning web app development is crucial for creating dynamic and interactive applications.
- Proven JavaScript techniques can help you build web applications quickly and efficiently.
- This article covers various aspects of web app development, including frontend and backend technologies, as well as important concepts like security and performance optimization.
Fundamentals of Web App Development
Web app development is the process of creating web applications that can be accessed through the internet by users. Before diving into the specifics of web app development, it is essential to understand the fundamentals of the technology.
Client-side vs. Server-side
At a high level, web app development involves two main components – client-side and server-side development. Client-side development involves designing and building the user interface, which users interact with. This involves writing code in HTML, CSS, and JavaScript. Server-side development involves building the backend of the application, where the data processing and storage occur. This typically involves writing code in languages such as PHP, Python, or Node.js.
Key Languages
Additionally, HTML is the foundation of web development, providing the structure and content for web pages. CSS is used to design and style web pages, adding visual elements such as colors, fonts, and layouts. JavaScript is a powerful programming language that adds interactivity and functionality to web applications, allowing developers to create dynamic web pages that can respond to user actions in real-time.
Understanding the DOM
Furthermore, understanding the Document Object Model (DOM) is crucial for web app development. The DOM is a programming interface for HTML and XML documents, allowing developers to manipulate the content and structure of web pages dynamically. The DOM is essential for creating interactive web pages and is closely linked to JavaScript programming.
Getting Started with JavaScript for Web App Development
If you want to build web applications, it is essential to have a solid foundation in JavaScript. In this section, we will provide a comprehensive guide to getting started with JavaScript specifically for web app development. We will cover the basics of JavaScript syntax, data types, variables, functions, and control structures.
JavaScript Syntax
JavaScript is a programming language used primarily for creating dynamic web applications. It is widely used for client-side scripting, server-side scripting, and cross-platform mobile app development. JavaScript syntax is similar to other programming languages like C++, Java, and Python, but there are some unique features that make it stand out.
JavaScript code is placed inside a script tag within the HTML file. The basic syntax is as follows:
<script> //JavaScript code goes here </script>
Data Types
JavaScript has several built-in data types, including strings, numbers, Booleans, null, undefined, objects, and arrays. Data types are used to store different kinds of information and perform operations on them.
Data Types | Description |
---|---|
String | Stores text values, enclosed in single or double quotes |
Number | Stores numeric values, including integers and floating-point numbers |
Boolean | Stores true or false values |
null | Represents the intentional absence of any object value |
undefined | Represents a variable that has not been assigned a value |
Object | Stores key-value pairs, also known as properties and methods |
Array | Stores a list of values, enclosed in square brackets |
Variables
Variables are used to store data values in JavaScript. They are declared using the var, let, or const keyword, followed by the variable name and optional value. Variables can be reassigned a new value later in the program, making them a powerful programming tool.
var myName = "John"; let myAge = 30; const PI = 3.14;
Functions
Functions are reusable blocks of code that can be called multiple times with different arguments. They are declared using the function keyword, followed by the function name, parameter list, and code block.
function addNumbers(num1, num2) { return num1 + num2; }
Control Structures
Control structures are used to control the flow of a program based on certain conditions. They include if…else statements, switch statements, loops, and try…catch statements.
if (score >= 70) { console.log("Pass"); } else { console.log("Fail"); }
By mastering these fundamental concepts, readers will be well-equipped to start building web applications using JavaScript.
Building User Interfaces with JavaScript Frameworks
Building user interfaces is a critical part of web app development, and JavaScript frameworks can help streamline the process. These frameworks provide developers with pre-built UI components and tools to create responsive and interactive user interfaces.
Popular JavaScript Frameworks for User Interface Development
React, Angular, and Vue.js are some of the most popular JavaScript frameworks used for building user interfaces in web applications today. Each framework has its own strengths and is suited to different types of projects, so it’s important to choose one that best fits your needs.
Framework | Description |
---|---|
React | React is a component-based JavaScript library developed by Facebook. It is widely used for building large-scale and complex web applications. React provides a fast and efficient way to update the user interface in real-time, without requiring a page refresh. |
Angular | Angular is a comprehensive JavaScript framework developed by Google. It is designed for building dynamic, single-page web applications. Angular provides features like dependency injection and two-way data binding, which make it easy to manage complex UI components. |
Vue.js | Vue.js is a lightweight JavaScript framework that emphasizes simplicity and ease-of-use. It is well suited for small to medium-sized web applications. Vue.js provides a fast and reactive user interface, and has a growing community of developers. |
Benefits of Using JavaScript Frameworks
JavaScript frameworks provide several benefits when building user interfaces, including:
- Increased productivity and efficiency by using pre-built UI components
- Consistent design and user experience across the application
- Reduced development time and costs
- Better performance and responsiveness of the user interface
Implementing User Interfaces with JavaScript Frameworks
Implementing UI components with JavaScript frameworks involves creating reusable components and composing them to form the final user interface. This approach ensures consistency and simplifies maintenance of the application.
“JavaScript frameworks provide several benefits when building user interfaces, including increased productivity and efficiency.
Here is an example of a React component:
import React from 'react'; function App() { return
( <div> <h1>Hello, World!</h1> <p>This is my first React app.</p> </div> ); }
export default App;
This React component renders a simple HTML page containing a heading and a paragraph. With React, you can easily create more complex components and connect them to data sources to build dynamic user interfaces.
Managing Data with JavaScript Libraries
In modern web applications, managing data is a critical task. JavaScript libraries like Redux, MobX, and Axios are essential for efficient state management, data fetching, and asynchronous operations.
Redux is a predictable state container that helps manage the state of your application. It provides a central store that holds the state of your application and allows for easy data manipulation and retrieval. Redux has gained popularity due to its ability to handle complex state management in large-scale applications.
Additionally, MobX is another popular library that simplifies state management in a web application. It uses observable data structures to track changes in state and automatically updates the user interface to reflect those changes. MobX is easy to learn and integrates well with React, making it an excellent choice for managing state in React applications.
Furthermore, Axios is a powerful JavaScript library that simplifies sending HTTP requests from a web application. It supports all modern browsers and provides a simple API for sending requests, handling responses, and managing errors. Axios is widely used in web applications for fetching data from APIs and updating the application state accordingly.
Enhancing User Experience with JavaScript Animation and Effects
JavaScript animation and effects can take your web application’s user experience to the next level. With the right tools and techniques, you can create smooth animations, interactive elements, and engaging visual effects that make your application stand out.
There are several libraries and techniques available that can help you achieve these results. Below we discuss some of the most popular options:
CSS Animations
Using CSS animations is a simple way to add movement and transitions to your web application. By defining keyframes and animation properties in your CSS stylesheet, you can create dynamic effects that work across various browsers and devices.
GreenSock Animation Platform (GSAP)
GSAP is a powerful JavaScript animation library that provides a wide range of tools for creating advanced animations and effects. It offers a performant and flexible animation engine, timeline control, and plugins for adding complex effects like physics-based motion, morphing, and 3D transformations.
Smooth Transitions and Interactive Elements
When using JavaScript for animation and effects, it’s essential to ensure that transitions are smooth and feel natural to the user. Applying easing functions and using requestAnimationFrame can help achieve this. It’s also important to make interactive elements like buttons or menus respond quickly and smoothly to user input.
By using these techniques and libraries, you can create web applications that are not only highly functional but also visually appealing and engaging for users. Remember to always test your animations and effects across different devices and browsers to ensure a consistent experience.
Implementing Backend Functionality with Node.js
When venturing into web application creation, it’s undeniable that the backend plays a pivotal role, complementing the frontend. Node.js stands out as a renowned runtime environment, enabling JavaScript execution on the server side. Owing to its speed, scalability, and user-friendly nature, it’s a top pick for backend development.
Laying the Groundwork: Server-side Basics
Before immersing ourselves in Node.js, it’s imperative to grasp the foundational concepts of server-side development. At its core, a server is a computer program that awaits incoming client requests and reciprocates with pertinent data. Remarkably, Node.js empowers us to craft servers using JavaScript—a language many web developers are well-acquainted with.
Kickstarting Your Node.js Journey
To embark on our Node.js adventure, the initial step involves its installation. Whether through a package manager like npm or directly from the Node.js official website, the installation process is straightforward.
Node.js Essentials
Upon successful installation, the next phase is setting up our server. This begins by initiating a new Node.js project and generating a package.json file, which diligently records our project’s dependencies and configurations.
Subsequently, the integration of a web framework is paramount. Serving as a toolkit, a web framework comprises tools and libraries designed to expedite web application development. Renowned frameworks in the Node.js realm include Express, Koa, and Hapi.
With our chosen framework in place, the creation of server routes takes center stage. Defined as URL endpoints, routes enable our server to furnish responses. These routes are effortlessly defined using the intrinsic routing capabilities of our web framework.
Mastering HTTP Requests with Node.js
Within the Node.js ecosystem, the HTTP module stands as the guardian of incoming requests. This module is replete with methods and properties tailored for HTTP server creation.
Database Dynamics in Node.js
Storing and Retrieving Data: Beyond mere request management, data storage and retrieval are paramount. Node.js boasts compatibility with databases like MongoDB, MySQL, and PostgreSQL. Leveraging drivers such as Mongoose and Sequelize, database interactions become a breeze.
The Node.js Advantage
In conclusion, Node.js emerges as a formidable platform for crafting resilient web applications. When harmonized with a web framework and a robust database, it promises dynamic servers that scale seamlessly, even under substantial traffic.
Ensuring Web App Security with JavaScript Best Practices
Prioritizing Web App Security
As a web app developer, securing your application is paramount. Here are key practices to bolster your app’s security:
1. Input Validation
A frequent vulnerability is inadequate input validation. Always sanitize and validate input to guard against SQL injection and cross-site scripting.
2. Authentication and Authorization
Authentication verifies user identity, while authorization controls resource access. Implement robust mechanisms like multi-factor authentication and role-based access control.
3. Embrace HTTPS
HTTPS encrypts data between client and server. Always use it to shield sensitive data from potential interception.
4. Update Dependencies Regularly
Ensure your dependencies remain current to avoid known security flaws.
5. Integrate Security Headers
Security headers like X-XSS-Protection offer added protection. Implement them to defend against vulnerabilities like cross-site scripting.
6. Adopt Content Security Policy
Content Security Policy (CSP) dictates allowable resource loads. Use CSP to further safeguard against vulnerabilities.
Stay informed on security trends and practices to continuously shield your app from cyber threats.
Optimizing Web App Performance with JavaScript Techniques
Web application performance stands as a cornerstone of user satisfaction. Thus, for developers, optimization isn’t just a consideration—it’s a necessity. To ensure our web apps offer a swift and uninterrupted experience, let’s delve into some potent techniques:
1. Code Optimization: Streamlining for Speed Code optimization is all about refining the code to enhance its performance. This involves:
- Minification: Stripping away all redundant whitespace and comments.
- Tree shaking: Discarding any code that remains unused.
- Focusing on refining loops and ensuring correct variable scoping. These steps can significantly boost performance.
2. Asynchronous Programming: Multitasking Mastery Embracing asynchronous programming can elevate the performance of your web app. This approach:
- Enables multiple tasks to operate concurrently, which boosts performance and minimizes the risk of app hang-ups.
- Incorporates asynchronous functions and promises, ensuring that extensive tasks don’t hinder the main thread. The outcome? A more fluid user experience.
3. Lazy Loading: On-Demand Resources Lazy loading is the art of fetching resources only when they’re truly needed, instead of bulk-loading everything upfront. This strategy:
- Drastically cuts down the initial load time of a web application.
- Can be applied to various elements like images, videos, and other media. Additionally, segmenting code into tinier bundles can further optimize loading times.
4. Caching Strategies: Swift and Localized Data Access Caching is the technique of locally storing data that’s accessed frequently. This minimizes the need for constant network requests, thereby enhancing the performance of your web app. Key strategies include:
- Browser caching: This saves data directly on the user’s device.
- Server-side caching: This method caches data on the server, lightening the load on the database.
Incorporating these strategies, developers are well-equipped to ensure their web apps provide a rapid, agile, and uninterrupted experience for all users.
Conclusion – JavaScript Techniques
Learning web app development and building applications quickly using proven JavaScript techniques is essential for anyone looking to succeed in the tech industry. In this article, we have covered a wide range of topics, from the fundamentals of web app development to advanced JavaScript techniques for optimizing performance.
We have explored the role of client-side vs. server-side development, the importance of the DOM, and how to get started with JavaScript. Additionally, we have delved into popular libraries and frameworks for building user interfaces and managing data in web applications.
Furthermore, we have discussed backend functionality with Node.js, ensuring web app security with JavaScript best practices, and optimizing web app performance with various techniques.
Applying Knowledge and Continued Learning
It is now up to you to apply the knowledge gained and continue honing your skills in JavaScript and web app development. Always keep in mind the importance of staying up-to-date with industry trends and exploring new technologies.
Remember, practice makes perfect. The more you build applications using these proven JavaScript techniques, the more proficient and confident you will become. Stay curious and keep learning to succeed in this fast-paced and exciting field.
FAQ – JavaScript Techniques
What are the main components of web app development?
Web app development involves two main components – client-side and server-side development. Client-side involves designing the user interface with HTML, CSS, and JavaScript. Server-side involves building the backend where data processing and storage occurs, using languages like PHP, Python, or Node.js.
What are some benefits of using JavaScript frameworks like React, Angular, and Vue.js?
Benefits of using JavaScript frameworks include:
- Increased productivity and efficiency by using pre-built UI components
- Consistent design and user experience across the application
- Reduced development time and costs
- Better performance and responsiveness of the user interface
How can you manage data efficiently in a web application?
JavaScript libraries like Redux, MobX, and Axios are essential for efficient state management, data fetching, and asynchronous operations in web apps. They simplify managing data from APIs and updating the application state.
What techniques can be used to implement animations and visual effects?
Techniques for animations and effects include:
- CSS Animations – Simple way to add movement using CSS styles
- GreenSock Animation Platform (GSAP) – Advanced library for complex animations and effects
- Smooth Transitions – Using easing functions and requestAnimationFrame
What are some best practices for optimizing web app performance?
Best practices include:
- Code optimization – Minification, tree shaking, optimizing loops
- Asynchronous programming – Using async functions/promises
- Lazy loading – Only loading necessary resources
- Caching strategies – Browser and server-side caching
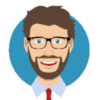
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.