Rapid application development has become a crucial element in the software industry landscape. As businesses strive to keep up with rapidly changing market demands, the ability to create high-quality software rapidly has become essential. JavaScript has emerged as a powerful programming language that empowers developers to build applications quickly and efficiently.
Key Takeaways
- Rapid application development is a critical element in the software development industry.
- JavaScript enables developers to create applications quickly and efficiently.
Benefits of Rapid Application Development
Rapid application development (RAD) methodologies offer numerous benefits to software development projects. By emphasizing quick iteration and collaboration, RAD can increase productivity and efficiency while decreasing development time and costs.
One of the main advantages of RAD is the ability to quickly respond to changing requirements and market demands. With RAD, developers can create functioning prototypes and receive feedback from stakeholders in a matter of days, rather than weeks or months. This promotes better collaboration between developers and stakeholders and results in products that more closely align with user needs.
RAD also tends to produce higher-quality software, as developers are able to identify and fix issues early in the development cycle. By iterating quickly, developers can catch bugs and usability issues before they become ingrained in the code. Additionally, RAD methodologies encourage the use of automated testing tools and continuous integration to ensure that code changes do not introduce new issues.
JavaScript’s Role in Rapid Application Development
JavaScript is a versatile scripting language used for creating web applications and dynamic user interfaces. Its popularity has grown tremendously due to its ability to enhance user experience and streamline application development. JavaScript plays a vital role in rapid application development due to its flexibility, scalability, and extensive community support.
With JavaScript, developers have access to numerous libraries, frameworks, and tools designed specifically for rapid application development. JavaScript libraries like jQuery, Angular, and React offer pre-built components and modules, enabling developers to build complex applications quickly.
JavaScript’s flexibility allows developers to create scalable and adaptable applications that can handle large amounts of data and support multiple users. Additionally, JavaScript frameworks like Node.js and Express enable developers to create server-side applications rapidly.
JavaScript’s vast community support provides developers with access to a wealth of resources, documentation, and sample code. This support network significantly speeds up application development, allowing developers to focus on building innovative features rather than reinventing the wheel.
Overall, JavaScript’s role in rapid application development cannot be overstated. Its flexibility, scalability, and community support make it an essential tool for developers looking to build applications quickly and efficiently.
Key Principles of JavaScript Rapid Application Development
JavaScript rapid application development follows certain basic principles that contribute to the efficiency and scalability of the applications. These principles include:
- Modular coding: Breaking down the code into independent modules makes it easier to maintain, test, and reuse the code, resulting in fewer bugs and faster development times.
- Reusability: Reusing code modules in multiple parts of the application saves a significant amount of time and effort in coding, testing, and debugging.
- Code optimization: Optimizing the code by removing redundancies and using efficient algorithms can speed up the application’s performance and reduce its memory footprint.
Implementing these principles requires adherence to best practices and coding standards. For example, using a consistent naming convention, commenting the code, and following the DRY (Don’t Repeat Yourself) principle can help ensure clarity and maintainability of the code.
Building High-Performing Applications with JavaScript
When building applications, it’s crucial to prioritize performance and efficiency. JavaScript provides several techniques for optimizing code and ensuring that your application runs smoothly. Here are some key strategies to consider:
1. Code Profiling
To improve application performance, it’s essential to identify and diagnose any bottlenecks or performance issues in your code. Profiling tools such as Chrome DevTools and Node.js’ built-in profiler can help you identify areas for improvement and optimize your application’s performance.
2. Minification
Minification is the process of removing unnecessary characters and whitespace from your code to reduce its file size and improve performance. This can significantly improve page load times and user experience.
3. Caching
Caching involves storing frequently accessed data in memory to reduce future database queries and improve application performance. Caching techniques such as memoization and local storage can significantly improve your application’s performance.
4. Asynchronous Programming
Asynchronous programming allows your application to perform multiple tasks concurrently, reducing the time it takes to complete actions and improving performance. JavaScript’s async/await and Promises features allow developers to write asynchronous code that is easy to read and maintain.
By implementing these strategies and techniques, developers can create high-performing JavaScript applications that provide a seamless user experience.
Scalability in JavaScript Rapid Application Development
One of the biggest challenges in rapid application development with JavaScript is ensuring scalability. As the user base grows and the volume of data increases, the application must be able to handle the load and maintain high performance. Here are some strategies for addressing scalability issues:
Handling Large Datasets
For applications that deal with large datasets, it’s important to optimize data retrieval and processing. This can be achieved through techniques such as database indexing, data partitioning, and caching. Additionally, adopting a serverless architecture can help to scale applications dynamically based on demand.
Load Balancing
Load balancing is the practice of distributing incoming network traffic across multiple servers to ensure that no single server is overworked. This can be achieved through various load balancing algorithms and tools, such as NGINX or Amazon Elastic Load Balancing (ELB).
Horizontal Scaling
Horizontal scaling involves adding more servers to manage the increasing load, thus distributing the load across multiple nodes. This can be done through cloud-based services, such as Amazon Web Services (AWS) or Google Cloud Platform (GCP), which provide scalable infrastructure on demand.
By adopting these strategies and tools, developers can ensure that their applications remain scalable and high-performing even as the user base grows.
Tools and Frameworks for JavaScript Rapid Application Development
JavaScript has a vast array of frameworks and libraries that can make rapid application development faster and more efficient. Here are some of the most popular tools and frameworks used by developers for building fast and scalable web applications.
React
React is a popular JavaScript library for building user interfaces and is widely used by developers for building single-page applications. It offers a reusable component-based architecture, making it easy to build complex interfaces quickly. React provides a virtual DOM, which enhances performance by only rendering the components that have changed.
Angular
Angular is a popular JavaScript framework developed by Google for building dynamic web applications. It provides features that allow developers to build scalable and maintainable applications, including two-way data binding, dependency injection, and component-based architecture. Angular offers a powerful CLI tool for generating components and services, making it easy to develop complex applications quickly.
Vue.js
Vue.js is a lightweight JavaScript framework for building user interfaces and single-page applications. It offers a simple and intuitive API and has a small footprint, making it fast and easy to learn. Vue.js provides reactive data binding, component-based architecture, and easy integration with other libraries and frameworks.
Node.js
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to use JavaScript on the server-side, making it possible to build fast and scalable applications. Node.js provides a rich set of APIs for building web servers, and it has a large community of developers creating packages and modules for extending its functionality.
These are just a few examples of the many JavaScript tools and frameworks available for rapid application development. By leveraging the power of these tools, developers can build high-performance, scalable applications quickly and efficiently.
Best Practices for JavaScript Rapid Application Development
JavaScript rapid application development can be a powerful tool for creating efficient and scalable applications. However, without proper planning and implementation, it can also lead to issues in code organization and scalability. Here are some best practices to help ensure successful JavaScript rapid application development:
- Modular Coding: Break your application’s code into smaller, reusable modules to simplify debugging and maintenance. Utilize tools like Webpack or Rollup to bundle these modules together for production use.
- Version Control: Use a version control system like Git to track changes to your codebase and collaborate with others. Utilize branching and tagging features to manage separate development and production branches.
- Code Optimization: Write clear, concise code that is optimized for performance. Utilize tools like Lighthouse or PageSpeed Insights to identify potential bottlenecks and areas for improvement.
- Documentation: Create and maintain clear documentation for your codebase, including API references and technical instructions. Utilize tools like JSDoc or Swagger to generate documentation automatically from source code comments.
- Testing Methodologies: Utilize automated testing tools like Jest or Mocha to verify the functionality and reliability of your codebase. Implement a continuous integration/continuous deployment (CI/CD) pipeline to automate the testing and deployment process.
- Error Handling: Implement robust error handling mechanisms throughout your codebase to ensure graceful degradation of your application in the event of errors or unexpected behavior.
By following these best practices, you can ensure that your JavaScript rapid application development projects are efficient, scalable, and maintainable over the long term.
Conclusion
In today’s fast-paced software development industry, rapid application development has become increasingly important. By leveraging the power of JavaScript, developers can build applications quickly and efficiently, resulting in decreased development time, increased productivity, and improved collaboration with stakeholders.
JavaScript provides essential features and libraries that enable rapid development, including flexibility, scalability, and extensive community support. By following key principles such as modular coding, reusability, and code optimization, developers can ensure their applications are high-performing and scalable.
There are numerous tools and frameworks available for JavaScript rapid application development, including React, Angular, Vue.js, and Node.js. By adopting best practices such as code organization, version control, documentation, and testing methodologies, developers can streamline their development process and create quality applications.
Unlocking the Full Potential of JavaScript Rapid Application Development
As we’ve seen, JavaScript rapid application development can offer significant benefits for developers. By implementing the strategies and practices outlined in this article, developers can unlock the full potential of JavaScript and create powerful, scalable applications that meet the needs of modern businesses and users.
Don’t be afraid to experiment with different tools, frameworks, and methodologies to find what works best for your development team. With the right approach, JavaScript rapid application development can be a game-changer for your software development projects.
FAQ
Q: What is rapid application development?
A: Rapid application development refers to a software development methodology that prioritizes speed and efficiency in creating applications. It emphasizes iterative development, collaboration between stakeholders, and the use of reusable components.
Q: Why is rapid application development important in the software development industry?
A: Rapid application development allows for faster delivery of applications, increased productivity, and improved collaboration between developers and stakeholders. It helps reduce development time, costs, and risks associated with traditional development approaches.
Q: How does JavaScript contribute to rapid application development?
A: JavaScript plays a crucial role in rapid application development due to its flexibility, scalability, and extensive community support. It provides essential features and libraries that enable developers to build applications quickly and efficiently.
Q: What are the key principles of JavaScript rapid application development?
A: Key principles of JavaScript rapid application development include modular coding, reusability, and code optimization. These principles promote maintainability, scalability, and efficiency in application development.
Q: How can I build high-performing applications with JavaScript?
A: To build high-performing applications with JavaScript, you can optimize your code by using techniques such as code profiling, minification, caching, and asynchronous programming. These techniques help improve performance and efficiency.
Q: What are the scalability challenges in JavaScript rapid application development?
A: Scalability challenges in JavaScript rapid application development include handling large datasets, load balancing, and horizontal scaling to accommodate growing user bases. Strategies such as effective data management and distributed architectures can address these challenges.
Q: What are some tools and frameworks for JavaScript rapid application development?
A: Popular tools and frameworks for JavaScript rapid application development include React, Angular, Vue.js, and Node.js. These frameworks offer features and advantages that facilitate rapid development and enhance productivity.
Q: What are the best practices for JavaScript rapid application development?
A: Best practices for JavaScript rapid application development include proper code organization, version control, documentation, and testing methodologies. Following these practices can ensure the quality and maintainability of your applications.
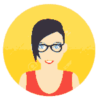
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.