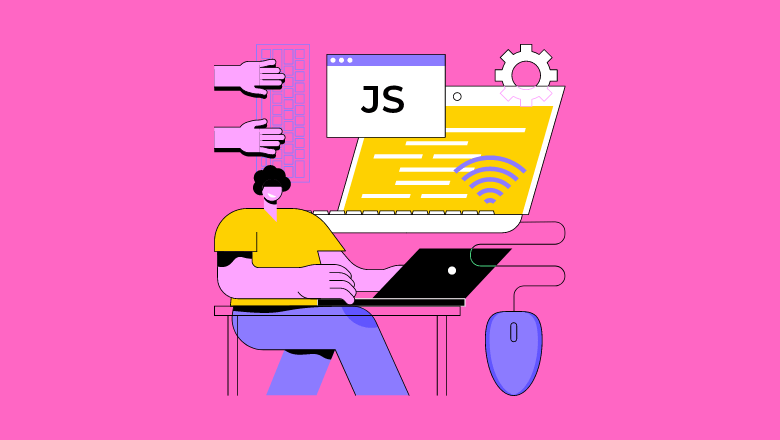
Programming languages such as JavaScript are among the most popular today. It is an integral part of modern web development, and many developers rely on it to build complex and interactive web applications. However, JavaScript has its challenges. In this article, we will explore 11 uncommon JavaScript issues developers face and provide tips and solutions to help you overcome them.
Issue 1: Scope and Hoisting
One of the most common JavaScript issues developers face is related to the concepts of scope and hoisting.
You can declare and access JavaScript variables using these two concepts throughout your code.
Scope refers to the visibility of a variable within a particular part of your code. In JavaScript, there are two main types of scope: global scope and local scope. A variable declared outside of a function has a global scope, enabling access to it from any part of the program.
Function-declared variables are local to that function and can only be accessed within it.
JavaScript behavior referred to as hoisting, allows the use of variables before their declaration. This can cause unexpected results and bugs in your code if you must be aware of how it works.
To exemplify this issue, let’s say you have the following code:
function myFunction() {
console.log(x);
var x = 1;
}
myFunction();
In this code, we have a function called myFunction that logs the value of x to the console and then declares it as the number 1. However, when we call myFunction, we’ll see undefined logs in the console instead of the expected value of 1.
This happens because of hoisting. In JavaScript, variable declarations are moved to the top of their respective scope by the JavaScript engine, which means that our code is interpreted like this:
function myFunction() {
var x;
console.log(x);
x = 1;
}
myFunction();
So, when we log the value of x to the console before it’s declared, we get undefined instead of the expected value of 1.
To avoid this issue, always declare your variables at the top of their respective scope and be aware of the scope chain in your code. This will help you avoid unexpected results and make debugging your code more manageable.
Issue 2: Type Coercion
Type coercion is another JavaScript issue that can cause confusion and unexpected results in your code. Type coercion refers to converting a value from one data type to another.
The data type of a variable can change during the execution of your JavaScript code since JavaScript is dynamically typed. This can lead to type coercion, where JavaScript will try to convert a value to a different data type to complete a particular operation.
To exemplify this issue, let’s say you have the following code:
var x = 5;
var y = "10";
var z = x + y;
console.log(z);
In this code, we have a variable x assigned the number 5, a variable y assigned the string “10”, and a variable z assigned the result of adding x and y together.
When we log the value of z to the console, we expect to see the number 15. However, because JavaScript will try to convert the string “10” to a number to complete the addition, we’ll see the string “510” logged to the console instead.
To avoid this issue, you must know your variables’ data types and use the appropriate type conversion functions when necessary. For example, strings can be converted into integers or floating-point numbers using the parseInt() and parseFloat() functions.
By using these functions, you can ensure that your code behaves as expected and avoid unexpected type coercion issues.
Issue 3: Callback Hell
Callback hell occurs when you have multiple levels of nested callbacks, making your code difficult to read and maintain.
To avoid callback hell, if you want your code to be easier to read and understand, you should use promises, or async/await.
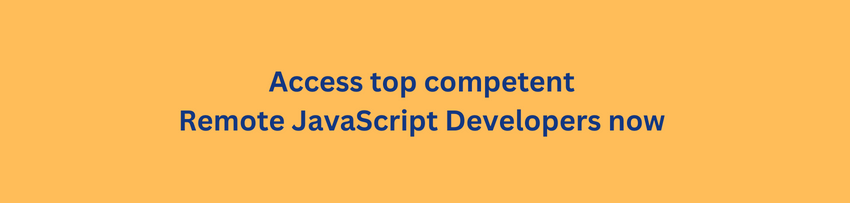
Issue 4: Event Bubbling and Capturing
Event bubbling and capturing are two ways that events propagate through the DOM. Event bubbling occurs when an event is triggered on a child element and then propagates to its parent elements.
On the other hand, event capturing occurs when an event is triggered on a parent element and then propagates to its child elements.
To avoid event bubbling and capturing issues, it’s essential to understand how they work and use event.stopPropagation() and event.preventDefault() methods to control their behavior.
Issue 5: Asynchronous Programming
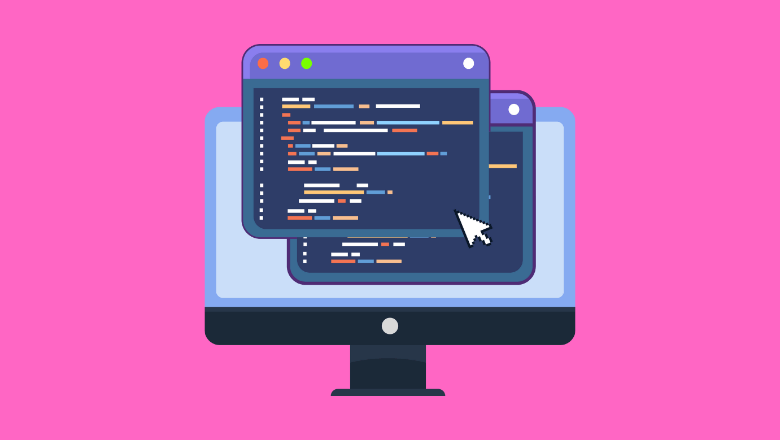
Asynchronous programming is a common technique used in JavaScript to handle long-running tasks without blocking the main thread.
To avoid issues with asynchronous programming, it’s essential to understand how it works, and use techniques such as callbacks, promises, and async/await to handle asynchronous tasks.
Issue 6: Memory Leaks
Your code can lead to performance issues and crashes if it continues to hold references to objects that are no longer needed, which is known as memory leaks.
You can avoid memory leaks by using closures and event listeners carefully since they can create references that are not immediately released. It would help if you also used tools such as the Chrome DevTools to identify and fix memory leaks in your code.
Issue 7: Cross-Site Scripting (XSS)
The cross-site scripting vulnerability occurs when malicious code is injected into a web page that others view.
To avoid XSS issues, it’s essential to use input validation and output encoding to prevent user input from being executed as code. You should also use Content Security Policy (CSP) headers to restrict the sources of executable scripts on your web pages.
Issue 8: Error Handling
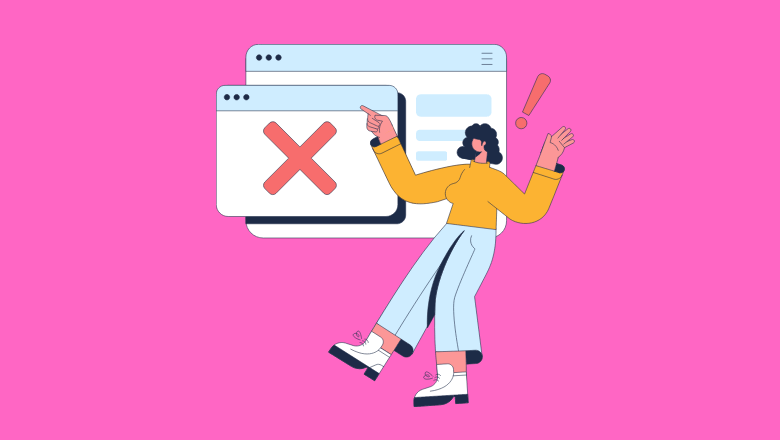
Error handling is essential to any programming language, and JavaScript is no exception.
To avoid issues with error handling, it’s essential to handle errors gracefully and provide clear error messages to users. It would help if you also used tools such as try-catch blocks and error-logging libraries to identify and fix errors in your code.
Issue 9: Security Vulnerabilities
Despite its ability to add interactivity and dynamic features to web pages, improper use of JavaScript can introduce security vulnerabilities.
Unvalidated input fields can be used by attackers to inject malicious code. This could result in potential security breaches or data theft.
To mitigate security vulnerabilities in JavaScript, developers should use appropriate security libraries and frameworks to validate input, sanitize data, and prevent XSS (cross-site scripting) and CSRF (cross-site request forgery) attacks.
Additionally, developers should follow best practices such as using secure authentication methods, encrypting sensitive data, and implementing access control and authorization mechanisms.
It’s also important to remember that security is an ongoing process and requires constant vigilance. Regular code reviews, security audits, and penetration testing can help identify and address potential vulnerabilities in your JavaScript code.
Issue 10: Debugging Complex Code
Debugging complex JavaScript code can be challenging, especially with large and intricate codebases. To simplify the process, developers can use tools and techniques such as breakpoints, console logging, and code analysis tools.
Using breakpoints allows developers to pause the execution of their code at specific points and inspect the values of variables and other data structures. Console logging is another helpful technique for debugging, allowing developers to print out messages and data to the browser console to help pinpoint issues.
Code analysis tools such as ESLint and JSHint can also help catch errors and enforce coding standards. You can integrate these tools into your development workflow and use them to identify potential issues before they cause problems in production.
Issue 11: Keeping Up with Emerging Technologies
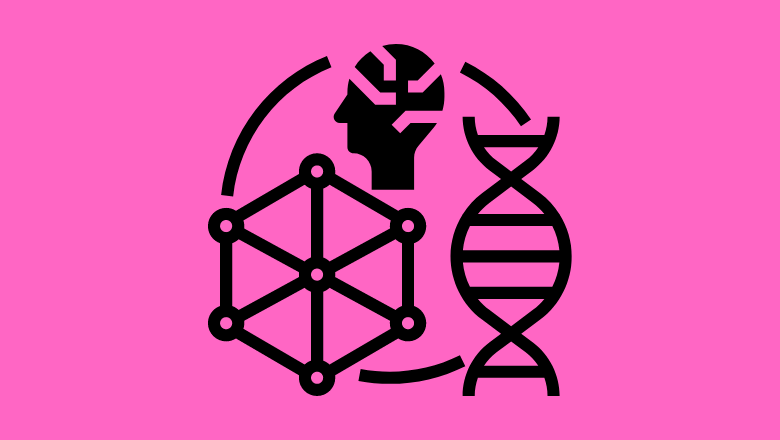
The JavaScript ecosystem is in a constant state of evolution with regular releases of new frameworks, libraries, and tools. These changes can be challenging, but developers must stay current to use the most efficient and effective tools and techniques.
Keeping up with emerging technologies requires developers to read industry publications, attend conferences, and participate in online communities. Take part in hackathons and personal projects to gain hands-on experience and learn new skills.
In order to solve complex problems and deliver high-quality applications, developers should stay current with emerging technologies.
That concludes our discussion on the 11 uncommon JavaScript issues that developers may face. With the right knowledge and tools, developers can overcome these issues and build robust and efficient applications.
Keep learning, experimenting, and improving your skills to stay on top of the JavaScript world.
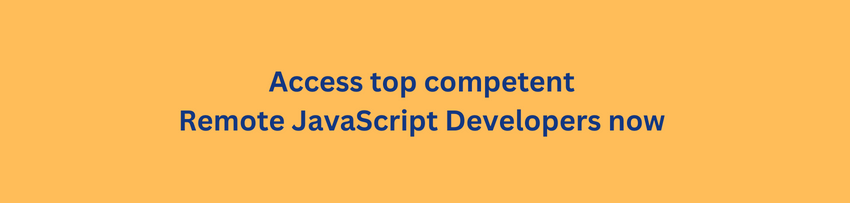
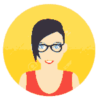
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.