JavaScript is a highly versatile programming language that supports multiple data types for storing and manipulating data. Boolean and integer are two data types often used in JavaScript, but sometimes, you may need to convert boolean values to integers.
If you’re looking to learn about js bool to int or converting boolean values to integers, you’re in the right place. Let’s dive in.
Boolean and Integer Data Types in JavaScript
JavaScript is a dynamically-typed language, which means that variables can dynamically change their type during runtime. The two most common data types in JavaScript are boolean and integer.
A boolean data type has two possible values: true and false. It is often used to represent logical values or conditions in programming. For example, a variable could be assigned the boolean value of true if a condition is met, and false if it is not.
On the other hand, an integer data type represents whole numbers, both positive and negative. It is commonly used in arithmetic operations, counting, and indexing. Unlike other programming languages, JavaScript does not distinguish between integers and floating-point numbers, as all numbers in JavaScript have the same type: Number.
While boolean and integer data types are distinct from each other, there are scenarios where converting between the two may be necessary. For instance, when dealing with user input, it may be necessary to convert boolean values to integers for calculations or comparisons.
Converting Boolean to Integer Using Type Conversion
JavaScript offers multiple methods for converting boolean values to integers. One of the simplest ways is to use type conversion, which allows us to change the data type of a value from boolean to integer.
Type conversion in JavaScript can be either implicit or explicit. Implicit type conversion happens automatically by JavaScript, while explicit type conversion requires us to specify the conversion.
Implicit Type Conversion
When we use a boolean value in a context where a number is expected, JavaScript will automatically convert it to either 0 or 1. For instance, when we add a boolean value to a number, JavaScript will implicitly convert the boolean to 0 or 1 and add it to the number.
Code | Result |
---|---|
1 + true | 2 |
2 + false | 2 |
Explicit Type Conversion
We can use explicit type conversion to convert a boolean value to an integer using the Number()
function, which converts a value to a number.
Code | Result |
---|---|
Number(true) | 1 |
Number(false) | 0 |
We can also use the unary plus (+) operator to convert a boolean value to a number. It is important to note that adding the unary plus before a string will also convert the string to a number.
Code | Result |
---|---|
+true | 1 |
+false | 0 |
The parseInt()
function is another way to convert a boolean value to an integer. This function parses a string and returns an integer. When we pass a boolean value to parseInt()
, the boolean is first converted to a string and then parsed to an integer.
Code | Result |
---|---|
parseInt(true) | NaN |
parseInt(false) | NaN |
parseInt(String(true)) | 1 |
parseInt(String(false)) | 0 |
It is important to note that if the boolean value is not first converted to a string using String()
, parseInt()
will return NaN (Not a Number).
In the next section, we will compare how different JavaScript frameworks handle the conversion of boolean values to integers.
Using the Number() Function for Boolean to Integer Conversion
The Number() function is a built-in JavaScript function that can be used for converting boolean values to integers. This method works by converting true to 1 and false to 0.
Here is an example of how to use the Number() function:
let boolValue = true;
let intValue = Number(boolValue);
console.log(intValue); // Output: 1
In the above code, we create a boolean variable named boolValue and set it to true. We then create a new variable named intValue and set it to Number(boolValue), which converts the boolean value to an integer value of 1. Finally, we log the value of intValue to the console.
When using the Number() function for boolean to integer conversion, it is important to note that any non-boolean value will result in NaN (Not a Number). Additionally, this method may not be suitable for converting large numbers of boolean values due to potential performance issues.
Overall, the Number() function is a simple and effective way to convert boolean values to integers in JavaScript.
Utilizing the + Operator for Boolean to Integer Conversion
The + operator is a versatile tool for converting boolean values to integers in JavaScript. It can be used for both implicit and explicit type conversion, making it a flexible option for developers.
When used explicitly, the + operator converts a boolean value to an integer by assigning a value of 1 to true and 0 to false. For example:
Code | Output |
---|---|
var boolVal = false; | |
var intVal = +boolVal; | 0 |
In the above code, the boolean value of false is explicitly converted to an integer value of 0 by using the + operator.
Implicit type conversion can also be achieved using the + operator. When a boolean value is combined with a number using the + operator, the boolean value is first converted to an integer and then added to the number. For example:
Code | Output |
---|---|
var boolVal = true; | |
var combinedVal = boolVal + 5; | 6 |
In this example, the boolean value of true is implicitly converted to an integer value of 1, and then added to the number 5 to produce a combined value of 6.
While the + operator is a convenient tool for converting boolean values to integers, it is important to use it with caution. It can easily lead to unintended consequences if used improperly, such as when combining strings and boolean values. Therefore, it is crucial to thoroughly test your code and avoid using the + operator for anything other than simple boolean to integer conversions.
Applying the parseInt() Function for Boolean to Integer Conversion
The parseInt() function is another popular method for converting boolean values to integers in JavaScript. It parses a string and returns an integer based on the given radix parameter. When used with a boolean value, it first converts the boolean value to a number using the implicit type conversion and then applies the parseInt() function.
Here is an example of using the parseInt() function for boolean to integer conversion:
const boolValue = true; const intValue =
parseInt(boolValue); console.log(intValue); // Output: 1
In the above example, the variable boolValue is a boolean value of true. The parseInt() function is called with the boolean value as an argument, which is then implicitly converted to a number and passed to the function. The returned value is the integer representation of the boolean value, which is 1.
It’s worth noting that the parseInt() function can also take a second parameter, which specifies the radix or base of the number system to use. For example, parseInt(“11”, 2) returns 3, as “11” is binary for 3.
However, when using the parseInt() function for boolean to integer conversion, it’s important to consider the potential pitfalls. If the boolean value is false, the function will return NaN (Not a Number) instead of 0. In addition, passing non-boolean values to the function can have unexpected results. For example, parseInt(“foo”) returns NaN, while parseInt(“10 foo”) returns 10.
Despite these considerations, the parseInt() function can be a useful tool for converting boolean values to integers in JavaScript.
Comparing Boolean to Integer Conversion in JavaScript Frameworks
When it comes to converting boolean values to integers in JavaScript, different frameworks may have varying approaches and nuances. In this section, we will compare how two popular frameworks, React and Angular, handle this conversion.
React
In React, the most common approach to converting boolean values to integers is to use the ternary operator. The ternary operator allows for concise and readable code that can easily be understood by other developers, making it a popular choice for many React projects.
// Converting boolean to integer using ternary operator in React
const boolValue = true;
const intValue = boolValue ? 1 : 0;
console.log(intValue); // Output: 1
However, it’s important to note that this approach may not be suitable for all cases, especially for larger and more complex projects that require more complex conversions. Additionally, using the ternary operator for every conversion can quickly become cumbersome and cluttered.
Angular
In Angular, a common approach to converting boolean values to integers is to use the Number() function. This method is straightforward and simple to understand, making it a popular choice for many Angular developers.
// Converting boolean to integer using Number() function in Angular
const boolValue = true;
const intValue = Number(boolValue);
console.log(intValue); // Output: 1
While using the Number() function is a common approach, it’s important to note that it may not be the most performant method for large-scale applications. Additionally, this approach may not be as readable or concise as other methods.
Overall, both React and Angular provide reliable and efficient approaches to converting boolean values to integers in JavaScript. The choice between the two may ultimately depend on the specific needs and requirements of the project at hand.
Best Practices for Converting Boolean to Integer in JavaScript
Converting boolean values to integers is a common task in JavaScript development. While it may seem simple, there are certain best practices you can follow to ensure your code is optimized for performance, readability, and maintainability.
Use Explicit Conversion
When converting boolean values to integers, it’s best practice to use explicit conversion methods rather than relying on implicit conversion. This is because implicit conversion can lead to unexpected behavior and bugs in your code.
For example:
const boolValue = true; const intValue =
boolValue * 1; // implicit conversion console.log(intValue); // output: 1
In the above code, the boolean value true is implicitly converted to the integer value 1. While this may work in some cases, it can lead to confusion and errors if the conversion is not intended. Instead, it’s better to use explicit conversion methods like the Number() function or the parseInt() function.
Check for Null or Undefined Values
Before converting boolean values to integers, it’s important to check if the value is null or undefined. This can help prevent errors and improve the overall quality of your code.
For example:
let boolValue; const intValue =
boolValue ? 1 : 0; // error, boolValue is undefined
In the above code, the boolean value is undefined, which can lead to errors when trying to convert it to an integer. To avoid this issue, you can use a nullish coalescing operator or an if statement to check if the value is null or undefined.
let boolValue; const intValue = boolValue ?? 0; // output: 0
Use Ternary Operators for Simple Conversions
For simple boolean to integer conversions, it’s best practice to use ternary operators instead of if statements or switch statements. This can help keep your code concise and easy to read.
For example:
const boolValue = true; const intValue = boolValue ? 1 : 0; // output: 1
Consider Performance Optimization
When converting boolean values to integers in performance-critical code, it’s important to consider the performance implications of each conversion method. Some methods may be faster than others, depending on the size of the data set and the frequency of the conversion.
For example, using bitwise operators like the | operator can be faster than using the Number() function or the parseInt() function for converting boolean values to integers. However, this method may not be as readable or maintainable as other methods.
Use Consistent Naming Conventions
When writing code that converts boolean values to integers, it’s important to use consistent naming conventions to make your code easier to read and maintain. This includes using descriptive variable names and avoiding abbreviations.
For example:
const isUserActive = true;
const userIsActive = isUserActive ? 1 : 0;
// better variable name and consistent naming convention
Test Your Code
It’s important to thoroughly test your code to ensure that your boolean to integer conversions are working as intended. This includes testing edge cases and input validation to catch any errors or unexpected behavior.
By following these best practices, you can ensure that your boolean to integer conversions are optimized for performance, readability, and maintainability in your JavaScript code.
External Resources
https://developer.mozilla.org/en-US/docs/Learn/Tools_and_testing/Client-side_JavaScript_frameworks
FAQ
Q: Can boolean values be converted to integers in JavaScript?
A: Yes, boolean values can be converted to integers in JavaScript using various methods.
Q: Why would I need to convert a boolean to an integer?
A: There may be scenarios where you need to convert a boolean value to an integer for calculations, comparisons, or data manipulation purposes.
Q: What are the different methods for converting boolean to integer in JavaScript?
A: There are multiple methods for converting boolean values to integers in JavaScript, including type conversion, using the Number() function, utilizing the + operator, and applying the parseInt() function.
Q: How does type conversion work for boolean to integer conversion?
A: Type conversion involves changing the data type of a value. In the case of boolean to integer conversion, JavaScript implicitly converts true to 1 and false to 0.
Q: What is the Number() function and how is it used for boolean to integer conversion?
A: The Number() function is a built-in JavaScript function that converts a value to a number. It can be used to convert boolean values to integers by passing the boolean value as an argument to the function.
Q: How does the + operator help with boolean to integer conversion?
A: The + operator can be used to convert boolean values to integers by applying it directly to the boolean value. JavaScript treats true as 1 and false as 0 when using the + operator for numeric calculations.
Q: What is the parseInt() function and how is it used for boolean to integer conversion?
A: The parseInt() function is a JavaScript function that parses a string and returns an integer. It can be used for boolean to integer conversion by passing the boolean value as a string to the function.
Q: Are there any differences in boolean to integer conversion between JavaScript frameworks?
A: Different JavaScript frameworks may handle boolean to integer conversion differently. It’s important to refer to the documentation and guidelines of the specific framework you are using.
Q: What are some best practices for converting boolean to integer in JavaScript?
A: Some best practices include ensuring proper error handling, writing readable code, and considering performance optimization techniques.
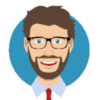
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.