React Query is a popular library for managing data in React applications. However, it’s not limited to React. Angular developers can also benefit from using React Query in their projects to streamline data fetching, caching, and synchronization.
Understanding React Query and Angular
React Query is a popular library used for managing server state in React applications. However, it can also be used in Angular projects to simplify the process of fetching data from a server and managing it in the client-side cache.
By using React Query in Angular, developers can take advantage of its powerful features such as automatic caching, background updates, and error handling, which can significantly improve the performance and user experience of web applications.
One of the main features of React Query is its ability to fetch and cache data from a server, automatically updating the cache as needed. This can reduce the number of server requests required and improve the speed and responsiveness of the application.
In addition, React Query provides a simple and intuitive API for working with server data, including query parameters, pagination, and optimistic updates. This can simplify the process of working with complex data models and APIs, making it easier for developers to build robust and scalable Angular applications.
Overall, using React Query in Angular can help developers streamline their development workflow and build high-performance, user-friendly web applications. In the next section, we will explore how to integrate React Query in an Angular project.
Integration Steps: Adding React Query to an Angular Project
Integrating React Query with an Angular project is an easy process that can be completed in a few steps.
Here’s how:
Step 1: Install React Query
The first step is to install React Query in your Angular project using your preferred package manager (npm or yarn).
Using npm:
npm install react-query
Using yarn:
yarn add react-query
Step 2: Create a Query Client
Next, create a query client that will be used to fetch data from your server. The query client is the main object you will use to communicate with React Query.
// Import the QueryClient and create a new instance import
{ QueryClient, QueryClientProvider } from 'react-query'; const queryClient =
new QueryClient();
// Wrap your app with the QueryClientProvider function App()
{ return ( <QueryClientProvider client={queryClient}> <YourApp />
</QueryClientProvider> ) }
Step 3: Use React Query Hooks to Fetch Data
Now, you’re ready to start using React Query hooks to fetch data in your Angular components. React Query provides several hooks that make it easy to fetch data and handle loading and error states.
Here’s an example:
// Import the useQuery hook import { useQuery } from 'react-query';
// Define a query key and a function to fetch data const QUERY_KEY =
'exampleData'; async function fetchExampleData() { const response =
await fetch('/api/example-data'); return response.json(); }
// Use the useQuery hook in your component function ExampleComponent()
{ const { data, isLoading, error } = useQuery(QUERY_KEY, fetchExampleData);
if (isLoading) { return <p>Loading data...; } if (error)
{ return <p>Error fetching data: {error.message}; }
return <p>Data: {JSON.stringify(data)}; }
With these simple steps, you can start integrating React Query in your Angular project and take advantage of its powerful features to simplify data fetching, optimize performance, and improve error handling.
Key Concepts and Features of React Query Angular
React Query offers several features that can significantly simplify data fetching and management for Angular developers. Here are some of the key concepts and benefits of using React Query in Angular applications:
1. Simplified Data Fetching and Caching
React Query simplifies the process of fetching and caching data, making it easy to manage and reuse data across your application. With React Query, you can fetch data from multiple sources, including APIs, databases, and local storage, and easily cache the data for faster performance and improved user experience.
For example, let’s say you have an Angular component that needs to fetch data from an API. Using React Query, you can define a query that fetches the data and caches it for future use. You can also set up automatic data refreshing and caching policies, so that your data is always up-to-date and your application is more responsive.
2. Automatic Data Synchronization
React Query also makes it easy to keep your data in sync with your backend. With built-in support for optimistic updates and real-time data synchronization, you can ensure that your application remains responsive and up-to-date, even when dealing with frequently changing data.
For example, let’s say you have an Angular app that displays real-time data, such as a social media feed. When a user posts a new message, you want the app to automatically refresh and display the new message without the user having to manually refresh the page. With React Query, you can easily set up real-time data synchronization and automatically update your UI when new data becomes available.
3. Error Handling
React Query provides built-in error handling mechanisms, making it easy to handle server errors and network failures. With React Query, you can define error states and display helpful error messages to users, improving the overall user experience of your application.
For example, let’s say you have an Angular component that displays data from an API. If the API returns an error, you can use React Query to display a helpful error message to the user and give them the option to retry the request or take another action.
Overall, React Query offers several benefits to Angular developers, including simplified data fetching and caching, automatic data synchronization, and error handling. By leveraging these features, you can build more responsive and user-friendly applications with less code and fewer headaches.
Performance Optimization with React Query Angular
One of the major benefits of using React Query in Angular development is its ability to optimize performance. React Query offers a caching mechanism that reduces the number of network requests and improves application speed. When data is fetched and cached, subsequent requests are served from the cache, eliminating the need for additional network requests.
Another performance optimization feature of React Query is automatic data refreshing. With traditional data fetching methods, developers have to manually implement code to refresh data on a regular basis. In contrast, React Query offers automatic data refreshing capabilities that help keep data up-to-date without any extra code.
React Query also offers query invalidation, which allows developers to easily update or invalidate stale data. This feature ensures that users always have access to the most current data and prevents them from seeing outdated or irrelevant information.
These performance optimization features are particularly useful for applications that rely heavily on data fetching and use a lot of network requests. They help reduce the load on servers and improve the overall user experience.
Handling Server Errors with React Query Angular
Server errors are a common occurrence in web development, and handling them can be a time-consuming and complex process. Fortunately, React Query offers built-in mechanisms for handling server errors that simplify this task.
One of the key benefits of using React Query in Angular development is the automatic handling of server errors. When a server error occurs, React Query will automatically set the isError property to true for any query that depends on the failed request. This signals to the application that an error has occurred and allows the appropriate error handling to be executed.
React Query also offers other features to facilitate error handling. For example, the retry feature allows failed queries to be automatically retried after a specified time interval. This can help prevent the user from experiencing a broken application due to server errors.
Here’s an example of how to handle server errors in an Angular application using React Query:
// Define a query
const { data, isError, error } = useQuery('users', fetchUsers, { retry: false });
// Render the error message if an error occurs
{isError && <p>{error.message}</p>}
In this example, the useQuery hook is used to define a query that fetches data from the server. The retry option is set to false to prevent automatic retries. The isError and error properties are destructured from the hook’s return value to help determine if an error has occurred.
The code then checks if isError is true and renders an error message with the {error.message} expression if it is. This ensures that the user is informed of the error and can take the appropriate action.
Overall, React Query’s error handling mechanisms help simplify the process of handling server errors in Angular applications, allowing developers to focus on building better user experiences.
Advanced Use Cases: React Query Angular in Practice
React Query offers a versatile set of features that simplify complex tasks in Angular development. Let’s explore some advanced use cases where React Query shines.
Pagination
Pagination is a common requirement in web applications. React Query offers an elegant solution with the usePaginatedQuery hook. This hook automatically handles the page number and page size, and retrieves the appropriate subset of data from the server.
Here’s an example:
// Define the query
const fetchUsers = async (page = 1) => {
const response = await fetch(`/api/users?page=${page}`);
return response.json();
}
// Use the usePaginatedQuery hook
const { resolvedData, latestData, isFetching, isFetchingMore, fetchMore } =
usePaginatedQuery(['users', 1], fetchUsers);
The fetchMore function can be used to retrieve more data as the user scrolls through the list. React Query handles the pagination automatically, making it easy to implement and maintain.
Optimistic Updates
Optimistic updates are a technique that provides instant feedback to the user while the server processes the request in the background. React Query supports optimistic updates out of the box with the useMutation hook.
Here’s an example:
// Define the mutation
const createTodo = async (data) => {
const response = await fetch('/api/todos', {
method: 'POST',
body: JSON.stringify(data),
headers: { 'Content-Type': 'application/json' }
});
return response.json();
}
// Use the useMutation hook
const [mutate, { isLoading }] = useMutation(createTodo, {
onMutate: (data) => {
// Optimistically update the UI
setTodos((todos) => [...todos, data]);
}
})
The onMutate function is called immediately before the mutation is sent to the server. The function receives the mutated data and can be used to update the UI optimistically. React Query manages the state of the mutation automatically and provides feedback to the user when the server responds.
Real-time Data Synchronization
Real-time data synchronization is crucial in many applications. React Query natively supports real-time updates with the useQuery hook. When combined with a real-time data source such as an SSE (Server-Sent Events) endpoint, React Query can automatically synchronize the data with the server in real-time.
Here’s an example:
// Define the query
const fetchTodos = async () => {
const response = await fetch('/api/todos');
return response.json();
}
// Use the useQuery hook with the staleTime option
const { data } = useQuery('todos', fetchTodos, { staleTime: Infinity });
// Set up the SSE connection and update the cache
const eventSource = new EventSource('/api/todos/stream');
eventSource.onmessage = (event) => {
const updatedTodo = JSON.parse(event.data);
// Update the cache
queryClient.setQueryData('todos', (todos) => {
const index = todos.findIndex((todo) => todo.id === updatedTodo.id);
if (index !== -1) {
todos[index] = updatedTodo;
}
return todos;
});
}
The staleTime option is set to Infinity to prevent the query from automatically refetching data. The real-time updates are handled through the SSE connection, which sends updates to the client as they occur. React Query updates the cache automatically, ensuring that the UI stays in sync with the server.
These are just a few examples of how React Query can streamline complex tasks in Angular development. With its versatile set of features, React Query offers numerous benefits to developers, including simplified data fetching and caching, automatic data synchronization, and error handling.
Tips and Best Practices for Using React Query in Angular
When using React Query in Angular, there are certain tips and best practices that can help developers make the most of this powerful tool. Here are some key insights to keep in mind:
1. Structure Queries Effectively
When structuring queries in React Query, it’s important to keep them organized and clearly labeled. This can be achieved by using descriptive names for queries and grouping related queries together.
It’s also helpful to create a centralized file or module for managing queries, which can simplify maintenance and updates.
2. Manage Cache Strategically
Managing cache is a critical part of using React Query effectively in Angular. By default, React Query stores cache in-memory, which can be limiting for larger applications.
One solution is to use an external caching library, such as Redux or Apollo Client, which can improve cache performance and scalability. Additionally, developers can optimize cache by adjusting the cacheTime and refetchInterval options, which control how frequently data is fetched and updated.
3. Handle Mutations Carefully
Mutations, or changes to data, can be complex to manage in React Query. It’s important to handle mutations carefully, especially when dealing with complex data structures or large databases.
One approach is to use optimistic updates, which allow the UI to respond immediately to user actions, while the mutation is still being processed in the background. Another technique is to use transactional updates, which group mutations together into a single transaction to improve efficiency and consistency.
4. Optimize Performance with Resource Limits
Optimizing performance is a key consideration when using React Query in Angular. One way to do this is by setting resource limits, which control how many queries can be executed simultaneously.
By using resource limits, developers can balance performance with scalability and avoid overloading the server with too many requests. Additionally, developers can use caching, as discussed earlier, along with query prefetching and data denormalization, to further optimize performance.
Final Thoughts about the Power of React Query in Angular
React Query is a powerful and versatile tool that can greatly enhance the development workflow for Angular applications.
By simplifying data fetching, optimizing performance, and offering built-in error handling mechanisms, React Query can help developers create more efficient and stable applications in less time.
Whether you’re working on a small project or a complex enterprise application, React Query has the tools you need to streamline your development process and deliver high-quality results.
So don’t hesitate to give React Query a try in your next Angular project.
With its intuitive interface, robust features, and dedicated community support, it’s sure to become an essential tool in your development arsenal.
External Resources
https://www.npmjs.com/package/react-query
FAQ
Q: Can React Query be used with Angular?
A: React Query can be integrated into an Angular project to enhance data fetching, caching, and synchronization capabilities.
Q: What are the benefits of using React Query in Angular?
A: React Query offers simplified data fetching, automatic cache management, and error handling, which can improve the performance and development experience in Angular projects.
Q: How can I integrate React Query into my Angular project?
A: To integrate React Query into an Angular project, you need to install the necessary dependencies, set up a QueryClientProvider, and use React Query hooks to fetch and manage data.
Q: Does React Query support pagination and real-time data synchronization in Angular?
A: React Query provides features like pagination and real-time data synchronization, making it suitable for handling complex use cases in Angular applications.
Q: Can React Query help optimize the performance of my Angular application?
A: React Query offers features like caching, automatic data refreshing, and query invalidation, which can enhance the performance of Angular applications by reducing network requests.
Q: How does React Query handle server errors in Angular?
A: React Query simplifies error handling in Angular applications by providing built-in mechanisms. It offers error handling hooks and customizable error handling strategies.
Q: Are there any best practices for using React Query in Angular projects?
A: Some best practices include structuring queries, managing cache effectively, handling mutations, and optimizing performance through proper configuration.
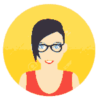
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.