React vs PHP for Web Development: Explore the strengths and weaknesses of React’s interactive front-end capabilities against PHP’s backend proficiency. Make informed decisions for your web development projects.
The argument that PHP is outdated and inferior to newer technologies like React for web development is a prevalent one.
But the truth is that existing legacy systems and a vast amount of online resources still rely heavily on PHP. This familiarity and dependency can make transitioning intimidating for many.
After navigating this transition myself and helping dozens of other developers do the same.
Understanding the Core Differences between React and PHP
Below is a concise understanding of the core differences between React and PHP, presented in a table format, along with code samples. Key terms are highlighted in bold for emphasis.
Aspect | React | PHP |
---|---|---|
Type | A JavaScript library for building user interfaces. | A server-side scripting language primarily used for web development. |
Main Use | Primarily used for building dynamic, interactive UIs in web applications. | Mainly used for server-side operations, such as accessing databases. |
Execution Environment | Runs on the client-side (in the user’s browser). | Executes on the server-side before sending HTML to the client’s browser. |
Data Handling | Handles the view layer (front-end) of web applications. | Used to handle data on the server (back-end), like database interactions. |
Architecture | Based on components, which are reusable UI elements. | Often follows a traditional server-side architecture (e.g., LAMP stack). |
Learning Curve | Requires understanding of JavaScript and modern front-end development. | Generally considered easier to start with, especially for backend development. |
React Code Sample:
import React from 'react';
function HelloComponent() {
return <h1>Hello, React!</h1>;
}
export default HelloComponent;
This sample shows a basic React component displaying “Hello, React!”. React components are used to build the UI.
PHP Code Sample:
<?php
echo "Hello, PHP!";
?>
This PHP code simply outputs “Hello, PHP!” to the browser. PHP scripts are executed on the server.
React is a front-end library used for building dynamic, interactive user interfaces, focusing on the view layer of web applications. In contrast, PHP is a server-side scripting language used primarily for back-end development, handling server operations and database interactions.
The Advantages of Using React for Front-End Development
Below is a table highlighting the advantages of using React for front-end development, along with relevant code samples. Key terms are highlighted in bold for emphasis.
Advantage | Details | Code Sample |
---|---|---|
Component-Based Architecture | React’s structure is based on reusable components, allowing for modular and maintainable code. | function Welcome(props) { return <h1>Hello, {props.name}</h1>; } |
JSX (JavaScript XML) | JSX combines HTML and JavaScript, making the code more readable and easier to write. | const element = <h1>Hello, JSX</h1>; |
Virtual DOM | Improves app performance and user experience by efficiently updating the UI. | Implicit in React’s rendering logic |
Declarative UI | Makes the code more predictable and easier to debug, as you design views for each state in the application. | function App() { return <Welcome name=”React” />; } |
Strong Community Support | Large community and ecosystem, with abundant resources and libraries available. | Not code-specific, but evident in libraries like Redux, React Router, etc. |
Ease of Integration | Can be easily integrated with other frameworks and technologies. | Integration patterns with APIs, libraries, etc. |
Learn Once, Write Anywhere | Learn the React way of building components and use it to develop web, mobile, or desktop apps with frameworks like React Native. | Conceptual, not code-specific |
React Code Sample:
import React, { Component } from 'react';
class Welcome extends Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
export default Welcome;
This code illustrates a basic React class component. It demonstrates the component-based architecture and the use of JSX.
React offers a component-based architecture that promotes reusable and maintainable code, uses JSX for easier and more readable code, employs a virtual DOM for performance efficiency, allows for declarative UIs that simplify debugging, benefits from strong community support, and its principles can be applied across platforms, embodying the philosophy of learn once, write anywhere.
Why Developers Choose PHP for Back-End Development
Below is a table summarizing why developers choose PHP for back-end development, complemented with relevant code samples. Key terms are highlighted in bold for emphasis.
Reason | Details | Code Sample |
---|---|---|
Ease of Learning | PHP is straightforward and easy to learn, especially for beginners in web development. | <?php echo “Hello, PHP!”; ?> |
Wide Range of Applications | Suitable for various web development needs, from small websites to large web applications. | // PHP can be used in various web apps |
Built-in Database Connectivity | PHP has excellent support for database integration, especially with MySQL. | $mysqli = new mysqli(“localhost”, “user”, “password”, “database”); |
Large Community and Resources | PHP has a vast community and a wealth of resources, frameworks, and documentation available. | Not code-specific, but evident in frameworks like Laravel, Symfony, etc. |
Cost-Effective and Accessible | It is open-source and runs on various platforms, making it a cost-effective solution for web development. | Implicit in PHP’s deployment and usage |
Mature and Stable | PHP has been around for a long time, making it a mature and stable choice for back-end development. | Implicit in PHP’s long-term use and evolution |
Built-In Web Development Capabilities | PHP comes with features tailored for web development, like handling sessions, cookies, and form data. | <?php session_start(); $_SESSION[“user”] = “John”; ?> |
PHP Code Sample:
<?php
// Connecting to a database
$mysqli = new mysqli("localhost", "username", "password", "database");
// Checking connection
if ($mysqli->connect_error) {
die("Connection failed: " . $mysqli->connect_error);
}
echo "Connected successfully";
?>
This code sample demonstrates a basic database connection in PHP, showcasing its built-in database connectivity.
Developers choose PHP for back-end development due to its ease of learning, making it accessible for beginners. It’s versatile for a wide range of applications, has robust built-in database connectivity options, and is supported by a large community.
PHP’s cost-effectiveness and accessibility, along with its maturity and stability, make it a reliable choice. Additionally, its intrinsic web development capabilities, like session and cookie management, streamline the development process.
Comparative Analysis: React vs PHP Performance
Here’s a comparative analysis of React vs PHP in terms of performance, using a table and code samples. The descriptions are written in an active tone with bold key terms for emphasis.
Aspect | React | PHP |
---|---|---|
Execution Speed | React’s client-side rendering offers fast UI updates. | PHP’s server-side rendering can be slower for complex operations. |
Resource Handling | Handles dynamic content efficiently with the virtual DOM. | Handles server-side computations, potentially heavier on resources. |
Scalability | Scales well in complex applications, especially single-page applications. | Scales well for diverse back-end needs, but can be limited by server capabilities. |
Load Time | Initial loading can be slower due to JavaScript bundle size. | Generally faster initial load due to server-side HTML rendering. |
Data Handling | Excels in real-time data handling with state management. | Better for large-scale data operations on the server-side. |
SEO Optimization | Requires additional configurations for SEO optimization. | Naturally better for SEO due to server-side rendering. |
React Code Sample:
function Content() {
const [data, setData] = React.useState(null);
React.useEffect(() => {
fetchData().then(setData);
}, []);
return <div>{data}</div>;
}
React efficiently updates only the necessary parts of the UI, enhancing performance.
PHP Code Sample:
<?php
$data = fetchDataFromDatabase();
echo "<div>" . $data . "</div>";
?>
PHP handles server-side data before delivering it to the client, impacting load times.
React shines in providing fast UI updates and handling dynamic content, suitable for complex and interactive web applications. PHP, being server-side, is more resource-intensive but excels in handling large-scale data operations.
While React requires extra steps for SEO optimization, PHP naturally performs better in this aspect due to server-side rendering.
Scalability Showdown: React vs PHP for Growing Applications
Aspect | React | PHP |
---|---|---|
Handling User Load | React excels in managing high user interaction. | PHP can struggle with high user load due to server-side processing. |
Modular Development | Component-based design aids in scaling features easily. | Script-based; modular but may require more architecture for scalability. |
Performance Optimization | Virtual DOM optimizes rendering, beneficial for large apps. | Performance depends on server resources and optimization techniques. |
State Management | Advanced state management options with Redux, Context API. | Manages state on the server, can be complex in large applications. |
Integration Flexibility | Easily integrates with other technologies and microservices. | Integrates with various databases and server-side technologies. |
Real-Time Data Handling | Ideal for apps with real-time data needs like SPAs. | Not optimal for real-time; better for traditional web applications. |
React Code Sample:
const [users, setUsers] = useState([]);
useEffect(() => {
fetchUsers().then(data => setUsers(data));
}, []);
React handles dynamic changes efficiently, crucial for scalable apps.
PHP Code Sample:
<?php
$users = fetchUsersFromDatabase();
foreach ($users as $user) {
echo "<p>" . $user['name'] . "</p>";
}
?>
PHP processes data server-side, which can be less efficient for high loads.
React is excellent for apps requiring high user interaction and dynamic updates, thanks to its component-based design and virtual DOM. PHP, while capable of scaling, may face challenges with high user load and real-time data due to its server-side nature.
React’s state management and integration flexibility make it more adaptable for growing applications, especially single-page applications (SPAs) and those with real-time requirements.
Breaking Down React vs PHP for Building Dynamic Web Applications
Aspect | React | PHP |
---|---|---|
Front-End Interactivity | React excels in creating interactive UIs. | PHP is less focused on front-end, handles server-side logic. |
Data Rendering | Renders data client-side, ideal for dynamic content updates. | Renders data server-side, then sends HTML to the client. |
User Experience | Offers seamless user experiences in single-page applications. | Can be slower for dynamic content due to page reloads. |
Development Approach | Component-based approach for UI building blocks. | Script-based approach, focusing on server-side operations. |
State Management | Efficient state management for responsive interfaces. | Manages state on the server, leading to more HTTP requests. |
SEO Friendliness | Requires additional setup for SEO optimization. | Naturally better for SEO due to server-side rendering. |
React Code Sample:
function DynamicContent() {
const [data, setData] = React.useState(null);
React.useEffect(() => {
fetchData().then(setData);
}, []);
return <div>{data}</div>;
}
React’s client-side rendering is perfect for dynamic and responsive interfaces.
PHP Code Sample:
<?php
$data = fetchData();
echo "<div>" . $data . "</div>";
?>
PHP handles data on the server, sending complete HTML to the client.
React is the go-to for building highly interactive and dynamic user interfaces, leveraging its component-based structure and efficient state management.
PHP, on the other hand, is more suitable for applications where server-side rendering and operations are the focus, although it may not be as responsive as React for dynamic content updates.
React requires extra SEO considerations due to its client-side nature, while PHP is more SEO-friendly out of the box.
Deciding Between React and PHP for Full-Stack Development
Aspect | React (Front-End) | PHP (Back-End) |
---|---|---|
UI Development | Ideal for interactive and dynamic UIs. | Not used for UI; focuses on server-side logic. |
Server-Side Logic | Needs integration with a back-end service or API. | Handles server-side processing, database interactions. |
Data Handling | Manages state and data flow on the client side. | Manages data on the server, handles HTTP requests. |
Performance | Fast rendering with Virtual DOM for responsive interfaces. | Performance varies based on server capacity and optimization. |
Scalability | Scales well in complex, client-heavy applications. | Scales with server-side resources and architecture. |
Ecosystem & Tools | Rich ecosystem with libraries like Redux, Axios. | Wide range of frameworks and tools, like Laravel, Symfony. |
React Code Sample:
function fetchUserData() {
const [userData, setUserData] = React.useState(null);
React.useEffect(() => {
axios.get('/api/user').then(response => setUserData(response.data));
}, []);
return <div>{JSON.stringify(userData)}</div>;
}
React interfaces with a back-end API to manage data.
PHP Code Sample:
<?php
// API endpoint to fetch user data
header("Content-Type: application/json");
echo json_encode(fetchUserData());
?>
PHP handles server-side data fetching and API responses.
When choosing between React and PHP for full-stack development, consider React for building dynamic and responsive user interfaces. PHP, in contrast, is more suited for server-side logic and data management.
React requires back-end integration for full-stack capabilities, while PHP provides robust server-side solutions. Performance in React is client-dependent, focusing on UI speed, whereas PHP’s performance relies on server resources and architecture. Both have rich ecosystems offering various tools and libraries for development.
Examples of Projects Built with React and PHP
Here’s a table presenting some examples of projects that are typically built with React and PHP:
Project Type | React Usage Examples | PHP Usage Examples |
---|---|---|
Single Page Applications (SPAs) | Ideal for responsive, dynamic SPAs. | Used for backend API serving data to the SPA. |
E-commerce Platforms | Front-end development for interactive user interfaces. | Backend management for databases, payment processing, etc. |
Content Management Systems (CMS) | Building user-facing CMS dashboards. | Core development of CMS functionalities. |
Social Media Platforms | Creating real-time, interactive social feeds. | Handling server-side logic, user authentication, etc. |
Data Visualization Tools | Visualizing data in graphs, charts dynamically. | Backend data processing and serving APIs. |
Educational Platforms | Interactive educational tools and user interfaces. | Backend management of user data, course content, etc. |
In this table:
- React is primarily used for creating dynamic and interactive front-end user interfaces, excelling in real-time updates and responsive designs, often seen in SPAs and interactive platforms.
- PHP is utilized more for its server-side capabilities, managing back-end processes like database interactions, user authentication, and data serving, key in systems requiring robust back-end management.
Final Thoughts
In the intersection of React vs PHP for building websites, my analysis comes to a pivotal juncture. Picking the right technology stack hinges on the nuances of each project’s vision, user experience goals, and technical sustainability. React shines with its fast-rendering, dynamic user interfaces suitable for interactive, state-of-the-art web applications.
In contrast, PHP offers a dependable and mature server-side solution, easing the path from development to deployment with its extensive ecosystem. Balancing these insights with practical demands is essential for a future-proof and robust web development strategy.
External Resources
FAQ
How do React and PHP handle data fetching from a database?
React:
// Fetching data using React (requires a back-end API)
function fetchData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('/api/data').then(response => response.json()).then(setData);
}, []);
return <div>{data}</div>;
}
PHP:
// Fetching data in PHP (direct database access)
<?php
$conn = new mysqli("localhost", "username", "password", "database");
$result = $conn->query("SELECT * FROM table");
while($row = $result->fetch_assoc()) {
echo $row["data"];
}
?>
Can React and PHP be used together in a project?
React:
// React component fetching data from a PHP API
function App() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('/api/getData.php').then(response => response.json()).then(setData);
}, []);
return <div>{data}</div>;
}
PHP:
// PHP script providing API endpoint
<?php
header("Content-Type: application/json");
echo json_encode(["data" => "Data from PHP"]);
?>
How do state management and server-side logic compare in React vs PHP?
React:
// State management in React
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
PHP:
// Server-side logic in PHP
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Process form data
}
?>
How do React and PHP handle user authentication?
React:
// React handling front-end authentication (requires back-end verification)
const [loggedIn, setLoggedIn] = useState(false);
// Function to call PHP API for authentication
function authenticate(credentials) {
fetch('/api/authenticate.php', { method: 'POST', body: JSON.stringify(credentials) })
.then(response => response.json())
.then(data => setLoggedIn(data.authenticated));
}
PHP:
// PHP handling authentication on server-side
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$credentials = json_decode(file_get_contents("php://input"), true);
// Authenticate user
}
?>
How are forms handled in React vs PHP?
React:
// Handling forms in React
function Form() {
const [inputValue, setInputValue] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
// Process form data
};
return (
<form onSubmit={handleSubmit}>
<input type="text" value={inputValue} onChange={e => setInputValue(e.target.value)} />
<button type="submit">Submit</button>
</form>
);
}
PHP:
// Handling forms in PHP
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$inputValue = $_POST['inputName'];
// Process form data
}
?>
<form method="post">
<input type="text" name="inputName" />
<button type="submit">Submit</button>
</form>
These FAQs cover a range of common queries regarding the functionalities and integration of React and PHP in web development scenarios.
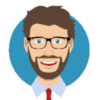
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.