Taking the easy road and sticking with Backbone.js is a surefire way to court obsolescence.
The truth is, many developers are sticking to Backbone because it’s what they know and are comfortable with. But this robs them of the benefits of evolving technologies.
Start embracing React.js’s component-based architecture and world-class ecosystem.
An Introduction to Backbone.js
Backbone.js is a lightweight JavaScript library pivotal for efficient web application development, particularly beneficial for early-stage startups. Here’s why understanding Backbone.js is crucial:
- MVC Framework: Backbone.js implements the Model-View-Controller (MVC) pattern, promoting organized code and clear separation of concerns. This aspect is key for maintaining and scaling applications as your team expands.
- Simplicity and Flexibility: Renowned for its simplicity and adaptability, Backbone.js provides essential tools for building your application’s architecture, offering more flexibility than heavier frameworks.
- Event-Driven Communication: It heavily relies on events for model-view communication, fostering a clean separation that’s beneficial for creating interactive, user-focused applications.
- RESTful JSON Interface: Backbone.js efficiently integrates with RESTful APIs, using a straightforward JSON interface, an essential feature for startups aiming to develop modern, high-performance web applications.
- Large Community and Resources: It boasts a mature community and a wealth of resources and tutorials, an invaluable asset for startups with limited resources.
- Use Cases: Backbone.js is particularly effective for single-page applications where control over structure is needed without the complexity of larger frameworks, aiding startups in developing applications that require quick iterations and adaptable architectures.
Examples of popular web applications built using Backbone.js include Trello, Foursquare, and Hulu.
For a growing startup, mastering Backbone.js can lay the foundation for building scalable and maintainable web applications, accommodating the dynamic demands of an expanding business.
An Introduction to React.js
React.js is a powerful JavaScript library used for building user interfaces, particularly single-page applications. Embracing React.js can be highly beneficial for several reasons:
- Component-Based Architecture: React allows you to build encapsulated components that manage their own state, then compose them to make complex UIs. This modular approach facilitates code reusability and easier maintenance.
- Declarative Views: React’s declarative nature makes your code more predictable and easier to debug. By designing simple views for each state in your application, React efficiently updates and renders the right components when your data changes.
- Virtual DOM: React implements a virtual DOM, which is a lightweight copy of the actual DOM. This feature ensures efficient updates and rendering, leading to improved performance and a smoother user experience.
- Strong Community Support and Ecosystem: With a vast and active community, React provides a wealth of resources, tools, and reusable components. This ecosystem is invaluable for startups looking to accelerate development with reliable, community-tested solutions.
- Integration with Other Libraries and Frameworks: React can be used with a variety of other libraries and frameworks, like Redux for state management or React Router for navigation, providing flexibility in building rich, interactive web applications.
- Suitability for Complex Applications: Its ability to handle complex state management and user interfaces makes React an excellent choice for startups aiming to build sophisticated web applications.
Understanding and utilizing React.js can significantly streamline the development process at your startup, helping you build robust, scalable, and maintainable web applications that can adapt to the evolving needs of your business.
Architectural Differences
Below is a table that outlines the key architectural differences between Backbone.js and React.js, along with code samples to illustrate these differences.
Aspect | Backbone.js | React.js |
---|---|---|
Core Philosophy | Provides minimal structure, more freedom in how you structure your app. | Emphasizes declarative UI, component-based architecture. |
Data Binding | Manual, more control over your bindings. | One-way data flow, with props and state. |
Rendering | Manual, typically uses Underscore.js templates. | Virtual DOM for efficient, declarative rendering. |
State Management | Managed within models, custom code for complex scenarios. | In-component state management, Redux for complex state. |
DOM Manipulation | Direct manipulation through jQuery or similar. | No direct DOM manipulation, uses a virtual DOM instead. |
Learning Curve | Lower, but requires more decisions about architecture. | Higher, due to unique concepts like JSX and virtual DOM. |
Community & Ecosystem | Mature, but smaller than React’s. | Extremely large and continuously growing. |
Code Sample – Backbone.js
var TodoView = Backbone.View.extend({
tagName: 'li',
// Cache the template function for a single item.
template: _.template($('#item-template').html()),
render: function() {
this.$el.html(this.template(this.model.toJSON()));
return this;
}
});
var todoView = new TodoView({model: myTodo});
Code Sample – React.js
class Todo extends React.Component {
render() {
return <li>{this.props.todo.text}</li>;
}
}
const todoElement = <Todo todo={{text: 'Learn React'}} />;
ReactDOM.render(todoElement, document.getElementById('app'));
These code samples demonstrate the fundamental differences in how Backbone.js and React.js approach UI rendering and structure.
Backbone.js provides more direct control over the DOM and data binding, while React.js introduces a more declarative approach with a virtual DOM and component-based architecture.
For a startup, the choice between Backbone.js and React.js can significantly impact development velocity, scalability, and maintainability of web applications.
Performance Comparison of Backbone.js and React.js
A performance comparison between Backbone.js and React.js is essential for making informed architectural decisions.
Here’s a concise comparison presented in a table format:
Aspect | Backbone.js | React.js |
---|---|---|
DOM Manipulation | Direct, manual DOM manipulation. | Uses a virtual DOM for minimal real DOM interaction. |
Data Binding | Manual data binding; can lead to performance issues in complex apps. | Efficient update and re-render mechanism via state and props. |
Rendering Performance | Requires manual optimization for complex UIs. | High performance in rendering complex UIs due to the virtual DOM and reconciliation algorithm. |
Memory Usage | Generally lower, but depends on manual optimization. | Slightly higher due to the virtual DOM, but managed efficiently. |
Startup Time | Faster initial load due to smaller library size. | Can be slower on initial load due to larger library size. |
Updates & Re-rendering | Manual control over rendering logic. | Automated and optimized through component lifecycle and hooks. |
Scalability | Good for small to medium applications; requires careful architecture for large apps. | Excellently suited for large applications with complex UIs. |
Code Sample – Backbone.js (Rendering and Updating)
var TodoView = Backbone.View.extend({
initialize: function() {
this.listenTo(this.model, 'change', this.render);
},
render: function() {
this.$el.html(this.model.get('title'));
return this;
}
});
var todoView = new TodoView({model: myTodo});
todoView.render();
Code Sample – React.js (Rendering and Updating)
class Todo extends React.Component {
render() {
return <div>{this.props.todo.title}</div>;
}
}
const todoElement = <Todo todo={{title: 'Learn React'}} />;
ReactDOM.render(todoElement, document.getElementById('app'));
In these code examples, Backbone.js requires manual setup for listening to model changes and re-rendering, while React.js automates this process with its state and props system, leading to a more efficient update and rendering process.
Overall, React.js generally offers better performance in handling complex UIs and large-scale applications, thanks to its virtual DOM and optimized rendering.
However, Backbone.js can be more performant for simpler applications with its direct and lean approach to DOM manipulation and smaller library size.
An Exploration of Community and Ecosystem Surrounding Backbone.js and React.js
Exploring the community and ecosystem surrounding Backbone.js and React.js is crucial, as it impacts the availability of resources, support, and future-proofing of technology choices. Here’s a concise comparison:
Aspect | Backbone.js | React.js |
---|---|---|
Community Support | Mature, but smaller. Strong early adopters but has plateaued. | Extremely large and continuously growing. |
Resource Availability | Good documentation, fewer new resources. Active in niche circles. | Extensive documentation, tutorials, and learning materials. |
Third-party Libraries | Good range of plugins and extensions, but fewer new developments. | Vast range of libraries and tools for almost every need. |
Talent Pool | Smaller, but experienced developers in Backbone.js. | Large pool of developers, easier to hire new talent. |
Industry Adoption | Used by established companies but less favored in new projects. | Widely adopted across industries, including major tech companies. |
Innovation and Updates | Slower, with fewer updates and innovations. | Rapid development, frequent updates, and cutting-edge features. |
Community Contributions | Stable, with some long-term contributors. | Highly active with contributions from individuals and companies. |
Integration with Other Technologies | Integrates well with older technologies and systems. | Easily integrates with modern technologies and toolchains. |
Future Proofing | More suitable for maintaining existing projects. | Highly suitable for new projects with long-term viability. |
Backbone.js
- Strengths: Stability in existing projects, simpler architecture.
- Considerations: Less suited for rapidly changing or cutting-edge projects.
React.js
- Strengths: Modern, highly scalable, and backed by a strong community.
- Considerations: Requires keeping up with frequent updates and changes.
Understanding these aspects will help you align your technology choices with your startup’s long-term vision and immediate project requirements. React.js, with its vibrant ecosystem and wide adoption, might be more suitable for startups looking to build scalable and future-proof applications.
Backbone.js, while having a stable and mature community, may be more appropriate for projects where a lightweight and straightforward approach is preferred, especially for teams with existing Backbone.js
Learning Curve and Documentation of Backbone.js and React.js
Understanding the learning curve and documentation of Backbone.js and React.js is vital for a CTO, particularly in an early-stage startup in the USA, as it impacts the ease of onboarding new developers and the speed of development. Here’s a concise comparison:
Aspect | Backbone.js | React.js |
---|---|---|
Learning Curve | Relatively gentle. Familiarity with JavaScript and jQuery is often sufficient. | Steeper due to JSX, virtual DOM concepts, and advanced state management techniques. |
Core Concepts | Models, Views, Collections, Events. | Components, JSX, State, Props, Lifecycle Methods, Hooks. |
Documentation | Clear and concise, but less extensive. Suitable for developers who prefer less abstraction. | Extensive and detailed, including guides, tutorials, and community contributions. |
Initial Setup | Minimal setup required. Easily integrates into existing projects. | Requires a build setup (like Create React App) for JSX and advanced features. |
Code Clarity | Straightforward, closer to vanilla JavaScript. More manual wiring needed. | Higher abstraction. Clear separation of concerns but requires understanding of React’s paradigms. |
Example Code | Basic view rendering is simple and easy to grasp. | Component-based approach might take time to understand for beginners. |
Backbone.js Code Sample
var TodoView = Backbone.View.extend({
tagName: 'li',
render: function() {
this.$el.html(this.model.get('title'));
return this;
}
});
var todoView = new TodoView({model: new Backbone.Model({title: 'Learn Backbone'})});
todoView.render();
React.js Code Sample
class Todo extends React.Component {
render() {
return <li>{this.props.title}</li>;
}
}
ReactDOM.render(<Todo title="Learn React" />, document.getElementById('app'));
In these examples, Backbone.js shows a more direct approach to JavaScript, which might be easier for those familiar with jQuery and vanilla JS. React, on the other hand, introduces JSX and a component-based approach, which provides powerful abstractions but requires a grasp of new concepts.
- Backbone.js: Ideal for teams with a strong foundation in traditional JavaScript and looking for a lightweight framework that adds minimal abstraction.
- React.js: Better suited for teams willing to invest time in learning modern JavaScript frameworks, offering a more robust and scalable solution for complex applications.
Your choice will depend on your team’s current skill set, the complexity of the projects, and the need for scalability and future growth.
React.js tends to be the go-to choice for new projects due to its large ecosystem and component-based architecture, while Backbone.js offers simplicity and ease of integration for smaller projects or teams with existing Backbone expertise.
Scalability and Maintainability
Scalability and maintainability are crucial factors for a CTO to consider, especially in a startup environment where resources are limited and future growth is a priority.
Here’s a concise comparison of Backbone.js and React.js regarding these aspects:
Aspect | Backbone.js | React.js |
---|---|---|
Scalability | Good for small to medium applications. Can become challenging in large, complex applications due to manual DOM manipulation and lack of component-based structure. | Excellently suited for large, complex applications. The component-based architecture makes it easier to manage and scale. |
Maintainability | Can be harder to maintain as projects grow due to less structure and potential for spaghetti code. Requires disciplined coding practices. | High maintainability due to component reusability, clear structure, and strong community support for best practices. |
State Management | Manual state management; can become cumbersome in large-scale applications. | Inherent state management within components; additional libraries like Redux offer advanced state management solutions. |
Code Modularity | Supports modular code but lacks the strict component structure of React. | Highly modular due to the component-based architecture, leading to better organization and separation of concerns. |
Update Management | Manual update management; requires careful coding to avoid performance issues. | Automated and efficient update process due to the virtual DOM and React’s diffing algorithm. |
Backbone.js Code Sample (Event Handling)
var TodoView = Backbone.View.extend({
events: {
'click .toggle': 'toggleCompleted'
},
toggleCompleted: function() {
this.model.toggle();
}
});
React.js Code Sample (Event Handling)
class Todo extends React.Component {
toggleCompleted = () => {
this.setState(state => ({
completed: !state.completed
}));
};
render() {
return <div onClick={this.toggleCompleted}>{this.props.title}</div>;
}
}
In these examples, Backbone.js shows a direct approach to event handling but lacks the streamlined state management seen in React.js. React’s use of state and the component lifecycle allows for more maintainable and scalable code in large applications.
- Backbone.js: Better suited for smaller projects or projects where full control over the architecture is required. It demands more discipline from developers to maintain scalability and maintainability.
- React.js: Ideal for large-scale applications due to its efficient handling of the DOM and state, as well as its modular component structure. It offers better tools and patterns out of the box for managing complex applications.
The decision should align with the project’s current and future complexity, team expertise, and the importance of rapid scaling and ease of maintenance. React.js is generally more suited for startups expecting significant growth and complex application development.
Integration and Compatibility
Ensuring that the chosen technology stack integrates well with other tools and remains compatible with various systems is vital. Here’s a concise comparison of Backbone.js and React.js in terms of integration and compatibility:
Aspect | Backbone.js | React.js |
---|---|---|
Integration with Existing Systems | Integrates well with traditional web technologies and server-side rendered pages. | Designed for modern web development; can be integrated into existing systems but may require additional configuration. |
Compatibility with Other Libraries | Easily pairs with other libraries like jQuery or Underscore.js. | Can coexist with other libraries, but its component-based nature may lead to conflicts if not managed properly. |
API Connectivity | Works seamlessly with RESTful APIs, but requires manual handling of updates and data synchronization. | Offers robust tools for connecting with APIs, especially with libraries like Axios or Fetch for data fetching. |
Ecosystem Tools | Limited to traditional web development tools. | Extensive range of tools available for development, testing, state management, etc. |
Mobile Development | Not directly suited for mobile development. | Compatible with mobile app development through React Native. |
Server-Side Rendering (SSR) | Possible, but not inherently designed for SSR. | Supports SSR natively, beneficial for SEO and performance. |
Backbone.js Code Sample (Integration with jQuery)
var $ = require('jquery');
var Backbone = require('backbone');
Backbone.$ = $;
var ListView = Backbone.View.extend({
// Backbone view code
});
React.js Code Sample (Integration with Axios for API Calls)
import React, { Component } from 'react';
import axios from 'axios';
class DataFetcher extends Component {
componentDidMount() {
axios.get('https://api.example.com/data')
.then(response => {
this.setState({ data: response.data });
});
}
render() {
// render data
}
}
In these code samples, Backbone.js easily integrates with jQuery, a familiar library in traditional web development. React.js, on the other hand, integrates efficiently with modern libraries like Axios for API interactions.
- Backbone.js: A good choice for projects that need to integrate with existing traditional web technologies, offering straightforward integration with a range of commonly used libraries.
- React.js: More suited for startups looking to leverage a modern and extensive ecosystem, especially if the project involves complex frontend requirements or mobile app development.
It’s crucial to consider both the current and future needs of the startup, ensuring that the chosen technology not only meets immediate requirements but is also capable of adapting to evolving technological trends and project scopes.
React.js is generally more adaptable for future growth and diverse development needs.
Choosing the Right Framework
Choosing the right framework between Backbone.js and React.js is a critical decision for a CTO, as it influences the development process, scalability, and future-proofing of your product. Here’s a concise comparison:
Factor | Backbone.js | React.js |
---|---|---|
Project Complexity | Better for simpler applications or where fine-grained control is needed. | Ideal for complex, interactive UIs and large-scale applications. |
Development Speed | Faster initial development due to simplicity, but can slow down as complexity increases. | Steeper learning curve, but components and tools speed up development in larger projects. |
Flexibility | High flexibility in architecture and design, with less opinionated structure. | More opinionated, especially about state management and component structure. |
Scalability | Can become challenging to scale due to manual DOM manipulations and lack of component-based architecture. | Excellent scalability due to component-based architecture and efficient DOM handling. |
Ecosystem & Community | Stable but smaller community. Less frequent updates and innovations. | Vibrant, large community with a rich ecosystem of tools and regular updates. |
Talent Availability | Fewer new developers are specializing in Backbone.js, but there’s a pool of experienced developers. | Large pool of developers, making it easier to find and hire qualified personnel. |
Backbone.js Code Sample (Basic Model and View)
var TodoModel = Backbone.Model.extend({
defaults: {
title: '',
completed: false
}
});
var TodoView = Backbone.View.extend({
render: function() {
this.$el.html(this.model.get('title'));
return this;
}
});
var todoModel = new TodoModel({ title: 'Learn Backbone' });
var todoView = new TodoView({ model: todoModel });
todoView.render();
React.js Code Sample (Component State and Rendering)
class Todo extends React.Component {
constructor(props) {
super(props);
this.state = { completed: false };
}
render() {
return (
<div>
{this.props.title} -
{this.state.completed ? 'Completed' : 'Not Completed'}
</div>
);
}
}
ReactDOM.render(<Todo title="Learn React" />, document.getElementById('app'));
In these examples, Backbone.js demonstrates a straightforward approach but requires manual management of model and view. React.js, with its stateful components, offers a more structured approach for managing UI and state.
- Backbone.js: Choose if your project is smaller or if your team is already experienced with Backbone.js and prefers a less opinionated framework.
- React.js: Opt for this if your project requires a robust, scalable solution for complex applications and you have access to or can develop React.js expertise.
Ultimately, the decision should align with the project’s requirements, your team’s skills, and the long-term vision for your product. React.js tends to be a more future-proof choice, especially for startups anticipating rapid growth and technological evolution.
Final Thoughts
The choice between Backbone.js and React.js for a CTO at a startup hinges on several key factors:
- Project Complexity and Scale: Backbone.js is suitable for simpler, smaller applications or projects where detailed control is preferred. React.js excels in handling complex, large-scale applications with its component-based architecture and efficient DOM management.
- Development Process and Speed: Backbone.js offers a quicker start for smaller projects due to its simplicity but may face challenges as complexity grows. React.js, while having a steeper learning curve, provides faster development for larger projects through reusable components and a rich set of tools.
- Community and Ecosystem: Backbone.js has a mature, stable community but with slower innovation and fewer new resources. React.js boasts a vibrant, continually evolving community with a wealth of resources and regular updates.
- Scalability and Maintainability: Backbone.js requires disciplined coding for scalability and maintainability, which can be challenging in larger applications. React.js, with its clear structure and state management, offers superior scalability and maintainability, especially for complex projects.
- Talent and Ecosystem: Finding experienced Backbone.js developers may be more challenging, whereas React.js has a larger pool of developers, making recruitment easier.
- Integration and Future-proofing: React.js is more adaptable to modern web development trends and offers better integration with a wide range of tools and technologies, making it a more future-proof choice.
While Backbone.js offers simplicity and fine-grained control, React.js is generally the more robust choice for startups looking to build scalable, maintainable, and future-proof web applications, especially in the dynamic and fast-paced startup ecosystem.
The decision should align with the specific needs, existing expertise, and future growth plans of the startup.
External Resources
FAQ
Here are five frequently asked questions (FAQs) about Backbone.js and React.js, each accompanied by a relevant code sample. These FAQs are tailored to provide valuable insights for a CTO managing a development team or evaluating these technologies for their startup.
FAQ 1: How do I handle user input and events in Backbone.js and React.js?
Backbone.js
Q: How do I bind a view to a DOM event in Backbone.js? A: Use the events
hash in your Backbone View. Code Sample:
var TodoView = Backbone.View.extend({
events: {
'click .toggle': 'toggleCompleted'
},
toggleCompleted: function() {
this.model.toggle();
}
});
React.js
Q: How do I handle a button click in React.js? A: Use an event handler function and bind it in the component’s render method. Code Sample:
class MyComponent extends React.Component {
handleClick = () => {
console.log('Button clicked');
};
render() {
return <button onClick={this.handleClick}>Click me</button>;
}
}
FAQ 2: How do I make an API call in Backbone.js and React.js?
Backbone.js
Q: How do I fetch data from an API in Backbone.js? A: Use a Backbone Model or Collection’s fetch
method. Code Sample:
var TodoCollection = Backbone.Collection.extend({
url: '/api/todos'
});
var todos = new TodoCollection();
todos.fetch();
React.js
Q: How do I fetch data from an API in React.js? A: Use the fetch
API or a library like Axios in a lifecycle method or useEffect hook. Code Sample:
class MyComponent extends React.Component {
componentDidMount() {
fetch('/api/todos')
.then(response => response.json())
.then(data => this.setState({ todos: data }));
}
render() {
// render todos
}
}
FAQ 3: How is state management handled in Backbone.js and React.js?
Backbone.js
Q: How do I manage state in Backbone.js? A: Manage state in Models and update Views when the model changes. Code Sample:
var TodoModel = Backbone.Model.extend({
defaults: {
title: '',
completed: false
}
});
var todoModel = new TodoModel();
todoModel.on('change', () => {
console.log('State changed');
});
todoModel.set({ title: 'New Todo' });
React.js
Q: How do I manage state in React.js? A: Use this.state
in class components or useState
hook in functional components. Code Sample:
function MyComponent() {
const [count, setCount] = React.useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
FAQ 4: How do I create a new view or component in Backbone.js and React.js?
Backbone.js
Q: How do I create a view for a model in Backbone.js? A: Define a View class extending Backbone.View
. Code Sample:
var TodoView = Backbone.View.extend({
render: function() {
this.$el.html(this.model.get('title'));
return this;
}
});
var todoView = new TodoView({model: new Backbone.Model({title: 'Learn Backbone'})});
todoView.render();
React.js
Q: How do I create a new component in React.js? A: Define a class extending React.Component
or use a function component. Code Sample:
class TodoComponent extends React.Component {
render() {
return <div>{this.props.title}</div>;
}
}
// Or using a function component
function TodoComponent({ title }) {
return <div>{title}</div>;
}
FAQ 5: How do you handle routing in Backbone.js and React.js?
Backbone.js
Q: How do I implement client-side routing in Backbone.js? A: Use Backbone.Router
. Code Sample:
var AppRouter = Backbone.Router.extend({
routes: {
'help': 'helpRoute'
},
helpRoute: function() {
// Handle the help route
}
});
var myRouter = new AppRouter();
Backbone.history.start();
React.js
Q: How do I handle routing in a React.js application? A: Use a third-party library like React Router. Code Sample:
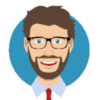
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.