Most people think that Node.js is the ultimate solution for backend development.
However, they forget how quickly the complexity can spiral out of control when building a sizable application.
After crafting dozens of successful products over the past five years, I’ve found that Nest.js provides a much more organized structure to keep code clean and manageable.
Let’s dive in and take a closer look at Nest.js and Node.js.
What is Nest.js?
Nest.js is a progressive Node.js framework for building efficient, scalable, and reliable server-side applications. It is built on top of the powerful and robust Express.js library, while also providing its own features and characteristics.
Nest.js allows developers to use modern JavaScript and TypeScript to build server-side applications. It utilizes decorators, dependency injection, and modules to provide a modular, flexible development experience. The following code example demonstrates the use of decorators in Nest.js:
@Controller('cats')
export class CatsController {
@Get()
findAll(): string {
return 'This action returns all cats';
}
}
The @Controller decorator is used to define a controller class for handling HTTP requests. The @Get decorator defines an HTTP GET endpoint that returns a string. This code demonstrates how Nest.js simplifies the creation of routes and request handling by using decorators and clear routing syntax.
Nest.js also enables developers to create reusable modules that can be easily imported and shared across multiple applications. The following code example showcases the use of modules in Nest.js:
@Module({
imports: [CatsModule],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
This code defines a module that imports a CatsModule and declares an AppController and an AppService. This enables easy separation of concerns and allows for better code organization in large applications.
What is Node.js?
Node.js is a popular backend framework that uses JavaScript as its primary language for server-side development. It is built on Chrome’s JavaScript runtime, V8, and is known for its scalability and performance. Node.js allows developers to create lightweight, high-performance applications that can handle a large number of concurrent connections.
One of the key advantages of Node.js is its event-driven, non-blocking I/O model. This means that Node.js applications can handle multiple requests simultaneously without blocking other requests. This is achieved through the use of callbacks, promises, and async/await functions.
Here is an example of how Node.js uses callbacks:
// Asynchronous function that logs a message after a delay
function logMessage(message, callback) {
setTimeout(() => {
console.log(message);
callback();
}, 1000);
}
// Call the asynchronous function with a callback
logMessage('Hello, Node.js!', () => {
console.log('Callback executed.');
});
In this example, the logMessage function takes a message and a callback function as arguments. The function logs the message after a delay of one second and then executes the callback function. The callback function logs a message that indicates that it has been executed.
Node.js also provides a rich set of modules and libraries that make it easy to develop web applications. It includes built-in modules for handling HTTP requests, working with file systems, and implementing security features. Additionally, there are countless third-party modules available on the npm registry that can be easily integrated into Node.js applications.
Overall, Node.js is a powerful backend framework that enables developers to build scalable, high-performance web applications using JavaScript.
Comparing Similarities
Although Nest.js and Node.js are different frameworks, they share some similarities. They both use JavaScript for server-side development, which allows developers to easily switch between client-side and server-side tasks. Both frameworks use the Express.js library to handle routing and request handling. Furthermore, developers can build middleware in both frameworks to modify requests and responses.
“Nest.js and Node.js are two popular backend frameworks, each with its own set of advantages and use cases.
Key Differences
While Nest.js and Node.js share similarities, they differ in significant ways.
Architecture: Nest.js offers a modular architecture that simplifies development by allowing you to break your application into smaller, reusable components. In contrast, Node.js provides developers with complete control over the architecture of their application.
Ease of Use: While both frameworks have a relatively low learning curve, Nest.js abstracts many of the complexities associated with raw Node.js, making it easier and faster to build web applications.
Level of Abstraction: Nest.js provides a higher level of abstraction compared to Node.js. It uses decorators and dependency injections to simplify development and ensure consistency. In contrast, Node.js requires developers to handle all aspects of application development and deployment manually.
Here’s an example of how Nest.js simplifies the development process:
// Without Nest.js
const server = http.createServer((req, res) => {
if (req.url === '/') {
res.write('Hello World!');
}
});
server.listen(3000);
// With Nest.js
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
getHello(): string {
return this.appService.getHello();
}
}
As you can see, Nest.js abstracts much of the underlying complexity, allowing developers to focus on writing code and building their application.
Scalability and Performance
When it comes to building a high-performance application that can handle a large number of concurrent requests, both Nest.js and Node.js are suitable options. However, there are some differences in the way they handle scalability and performance.
Node.js is known for its excellent scalability due to its non-blocking I/O model. This means that the server can handle multiple requests simultaneously, without blocking any other request. Node.js also has a small memory footprint, making it ideal for building lightweight applications that can handle high traffic.
On the other hand, Nest.js provides a higher level of abstraction and makes it easier to build scalable and maintainable applications. Nest.js uses the underlying principles of Node.js and integrates them with modules, controllers, and services that make the development process more structured and easy to manage.
One example of a highly scalable and performant application built with Nest.js is the microservice architecture used by Netflix. Netflix uses Nest.js to build and maintain their API gateway, which handles millions of requests per day.
Node.js | Nest.js |
---|---|
const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader(‘Content-Type’, ‘text/plain’); res.end(‘Hello, World!’); }); | @Controller() export class AppController { constructor(private readonly appService: AppService) {}@Get() getHello(): string { return this.appService.getHello(); } } |
Raw Node.js handling of requests in an event-driven architecture | Nest.js controller handling of requests with dependency injection |
As demonstrated in the code examples above, Nest.js provides a higher level of abstraction and easier-to-understand code that can make it simpler to scale and maintain complex applications over time.
Nest.js vs Node.js: Community and Ecosystem
When it comes to choosing a backend framework, it’s critical to evaluate the community and ecosystem surrounding it. In this section, we’ll explore the community support and available ecosystem for both Nest.js and Node.js.
Nest.js Community and Ecosystem
Nest.js is steadily growing in popularity, backed by a supportive and rapidly expanding community. It has a strong presence on GitHub, with over 30,000 stars and numerous contributors. The framework also has a very active Discord channel where developers can share their experiences, ask for help, and keep up-to-date with the latest news and updates.
There are various libraries and tools available that complement Nest.js, such as TypeORM for object-relational mapping, Passport.js for authentication, and Swagger for API documentation. These libraries integrate seamlessly with Nest.js, making it easy to build complex, production-ready applications with minimal setup.
Node.js Community and Ecosystem
Node.js has been around for over a decade and has gained widespread popularity within the web development community.
It has a massive user base and a well-established ecosystem of libraries and tools.
Similar to Nest.js, Node.js is also hosted on GitHub, with over 60,000 stars and thousands of contributors. The Node.js community is known for its constant innovation and cutting-edge technologies, such as the recently introduced Node.js 16.0 with V8 9.0 and enhanced diagnostics.
Node.js also has a wide range of libraries and tools to choose from, such as Express.js for building web applications, Mongoose for MongoDB object modeling, and Socket.io for real-time communication.
These libraries have been extensively tested and are widely used in the industry, making them reliable choices for building robust applications.
Which One Should You Choose?
Choosing between Nest.js and Node.js can be challenging, especially when it comes to community and ecosystem. Both frameworks have active communities and various libraries to choose from, making them excellent choices for backend development.
If you’re looking for a new and growing framework with a supportive community and easy-to-use libraries and tools, Nest.js might be the right choice. On the other hand, if you prefer a well-established and widely used framework with a massive ecosystem and a wide range of libraries and tools, Node.js might be the better option.
In the end, the choice will depend on your specific needs and preferences. We recommend trying out both frameworks to get a better understanding of their strengths and weaknesses and making your decision accordingly.
Learning Curve and Documentation
When it comes to learning curve and documentation, both Nest.js and Node.js have their strengths and weaknesses. Node.js has been around longer, and as a result, there is a vast amount of documentation available. Additionally, as a runtime environment, Node.js is relatively straightforward, so developers with experience in JavaScript can pick it up quickly.
On the other hand, Nest.js is a more complex framework, with its focus on modularity, and convention over configuration. While the official Nest.js documentation is comprehensive, it can be overwhelming for beginners. However, the framework offers plenty of resources and examples on their website, making it easy to get started.
Overall, Node.js is more accessible, and its documentation covers many use cases and scenarios. However, for developers looking to build complex applications, Nest.js provides a more structured approach to development. By following the framework’s conventions, developers can save time and avoid common pitfalls in large-scale projects.
Final Thoughts: Choosing the Right Backend Framework
After diving deep into the world of backend frameworks, it’s clear that both Nest.js and Node.js have their own unique strengths and weaknesses. Depending on the specific needs of your project, one may be a better fit than the other.
If you’re already familiar with JavaScript and want a more structured approach to server-side development, Nest.js could be the way to go. Its use of decorators and dependency injection can simplify the development process and make code more maintainable. Additionally, the Nest.js ecosystem offers a variety of plugins and modules to help optimize performance and streamline development.
On the other hand, if you’re looking for a more lightweight, barebones solution, Node.js may be the better choice. Its event-driven, non-blocking I/O architecture allows for high scalability and performance in high traffic scenarios. Node.js also benefits from a large and active community, with plenty of libraries and frameworks available for use.
Ultimately, the decision between Nest.js and Node.js comes down to your own preferences and project requirements. We encourage you to try out both frameworks and see which one feels like the best fit. Keep in mind the importance of a strong community and ecosystem, as well as comprehensive documentation. With a little bit of research and experimentation, you’ll be well on your way to choosing the right backend framework for your project.
External Resources
FAQ
Q: Can I use Nest.js and Node.js together?
A: Yes, Nest.js is built on top of Node.js, so you can use both frameworks together in your project.
Q: Is Nest.js suitable for large-scale applications?
A: Yes, Nest.js is designed to be scalable and can handle large-scale applications effectively.
Q: Do I need to learn JavaScript to use Node.js?
A: Yes, Node.js uses JavaScript as its primary language for server-side development.
Q: How does Nest.js handle routing and request handling?
A: Nest.js uses decorators and modules to handle routing and request handling in a structured manner.
Q: What is the main difference between Nest.js and Node.js?
A: The main difference is that Nest.js provides a higher level of abstraction and simplifies the development process compared to raw Node.js.
Q: Are both Nest.js and Node.js scalable and performant?
A: Yes, both frameworks are scalable and performant, but the specific performance can depend on the implementation and architecture of your application.
Q: What kind of community support is available for Nest.js and Node.js?
A: Both frameworks have active communities and a wide range of libraries, frameworks, and tools that complement their functionalities.
Q: How steep is the learning curve for Nest.js and Node.js?
A: The learning curve can vary depending on your familiarity with JavaScript and server-side development. However, Nest.js provides comprehensive documentation to help developers get started.
Q: What is the recommended framework for my project?
A: The recommended framework depends on your specific project needs. Consider the level of abstraction, ease of use, and community support when making a decision.
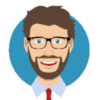
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.