Choosing the right backend framework is crucial for any development project. With so many options available, it can be overwhelming to decide which one fits your needs best. In this article, we will compare two popular frameworks: Nest.js and Node.js.
Node.js is a well-known backend framework that has been around since 2009. It is built on top of the Google Chrome V8 engine and allows developers to use JavaScript for server-side programming. Nest.js, on the other hand, is a relatively new framework that was introduced in 2017.
It is built on top of the Express.js framework and provides a more structured approach to building scalable and maintainable applications.
Understanding Node.js
Node.js is a popular open-source backend runtime environment that allows developers to build server-side applications using JavaScript. Developed by Ryan Dahl in 2009, Node.js has gained significant traction among developers due to its event-driven, non-blocking I/O model. It allows developers to build scalable and high-performance applications that can handle thousands of concurrent connections.
Node.js uses the Google V8 JavaScript engine, which compiles code into machine code for faster runtime execution. This means Node.js can handle a high volume of requests and perform complex computations with ease. Node.js is also known for its vast library of modules, which make it easy to build powerful web applications without having to write everything from scratch.
Key Features of Node.js
Node.js has several key features that make it a popular choice for backend development:
- Non-blocking I/O model for efficient concurrency
- V8 JavaScript engine for fast and efficient code execution
- Event-driven architecture for building scalable applications
- Rich library of modules for rapid application development
- Support for multiple platforms including Linux, Windows, and macOS
Advantages of Node.js
Node.js provides several advantages for backend development:
- Efficient and scalable architecture
- Faster development time due to vast library of modules
- Easy to switch between frontend and backend development as both can be done in JavaScript
- Good for building real-time applications that require quick data updates
- Extensive community support and excellent documentation
Use Cases for Node.js
Node.js is a popular choice for building various types of web applications, including:
- Real-time chat applications
- Streaming services
- Social media applications
- E-commerce platforms
- API-based applications
Node.js is a powerful and versatile backend framework that allows developers to build scalable and high-performance applications. Its event-driven, non-blocking I/O model makes it an excellent choice for building real-time applications. Node.js also has an extensive library of modules, which makes it easy to build complex applications in a fraction of the time.
Getting to Know Nest.js
Nest.js is a progressive Node.js framework for building scalable and efficient server-side applications. It is built on top of Express.js and provides a modular architecture that makes it easy to organize code and maintain a clear separation of concerns.
One of the most unique features of Nest.js is its use of decorators, which allow developers to easily define and inject dependencies, create middleware, and route endpoints. Nest.js also provides a powerful CLI (Command Line Interface) that helps with project scaffolding and automating repetitive tasks.
Dependency Injection
Nest.js makes use of Dependency Injection, which is a design pattern that promotes loose coupling and enhances the testability and reusability of code. With Nest.js, we can define providers and inject them into our controllers or services using decorators. For example, we can define a database connection provider and inject it into our service to access the database inside our controller methods.
Vanilla Node.js | Nest.js |
---|---|
// Vanilla Node.js Database Connection const MongoClient = require('mongodb').MongoClient; const url = 'mongodb://localhost:27017'; const dbName = 'myproject'; MongoClient.connect(url, function(err, client) { console.log("Connected successfully to server"); const db = client.db(dbName); client.close(); }); | // Nest.js Database Connection import { Injectable } from '@nestjs/common'; import { Connection } from 'mongoose'; @Injectable() export class DatabaseService { constructor(private readonly connection: Connection) {} async connect() { console.log('Connected successfully to server'); await this.connection.connect(); } } // Controller or Service import { Controller } from '@nestjs/common'; import { DatabaseService } from './database.service'; @Controller() export class AppController { constructor(private readonly dbService: DatabaseService) {} async getHello(): Promise { await this.dbService.connect(); return 'Hello World!'; } } |
Modularity
Nest.js provides a modular architecture that makes it easy to organize code and maintain a clear separation of concerns. Each module can contain controllers, services, and providers that are related to a specific feature or functionality of the application.
This modularity makes it easy to add or remove features without affecting the rest of the codebase.
Vanilla Node.js | Nest.js |
---|---|
|
|
Overall, Nest.js is a powerful backend framework that provides developers with the tools and structure to build high-quality server-side applications efficiently. Its use of decorators, dependency injection, and modularity makes it a popular choice for large-scale projects and microservices.
Express vs Nest.js Routing
Routing is a crucial aspect of backend development as it determines how the application handles requests and responses. In Node.js, routing is typically handled with the Express framework, while Nest.js provides its own routing system.
Let’s explore the differences between these two frameworks in terms of routing.
Express Routing
Express provides a straightforward routing system using HTTP verbs like GET and POST to handle requests. Here’s an example of an Express route for handling a GET request:
app.get('/users/:userId', function (req, res) {
res.send('User ID: ' + req.params.userId);
})
The above code creates a route for handling GET requests to the ‘/users/:userId’ endpoint, where ‘:userId’ is a parameter that can be accessed with ‘req.params.userId’.
Express also allows for middleware functions to be added to routes, which can perform actions like authentication or data validation before the final response is sent back to the client.
Nest.js Routing
Nest.js provides a more structured approach to routing with decorators that can be used to define routes and their associated HTTP verbs. Here’s an example of a Nest.js route:
@Get('/users/:userId')
getUser(@Param('userId') userId: string): string {
return 'User ID: ' + userId;
}
The ‘@Get’ decorator defines a route for handling GET requests to the ‘/users/:userId’ endpoint, and the ‘@Param’ decorator allows for easy access to the ‘:userId’ parameter.
Nest.js also provides middleware-like constructs called ‘guards’ and ‘interceptors’, which can be added to routes to perform actions before or after the request is handled.
Express vs Nest.js Routing: Which is Better?
Both Express and Nest.js have their advantages and drawbacks when it comes to routing. Express provides a more flexible and customizable system, while Nest.js offers more structure and organization.
Ultimately, the choice between these frameworks depends on the specific needs of the project and the preferences of the development team.
Database Integration: Node.js vs Nest.js
Both Node.js and Nest.js offer multiple approaches to database integration, making it a versatile choice for backend development.
In Node.js, the popular choice for database interaction is the Object-Relational Mapping (ORM) library called Sequelize. It supports multiple databases such as MySQL, PostgreSQL, and SQLite, and allows developers to work with database objects using JavaScript syntax. Here’s an example of a Sequelize model for a blog post:
const Sequelize = require('sequelize');
const sequelize = new Sequelize('database', 'username', 'password', {
host: 'localhost',
dialect: 'mysql'
});
const Post = sequelize.define('post', {
title: {
type: Sequelize.STRING,
allowNull: false
},
body: {
type: Sequelize.TEXT,
allowNull: false
}
});
Post.sync();
Nest.js, on the other hand, provides a built-in Object Document Mapper (ODM) called TypeORM, which supports multiple databases such as MySQL, PostgreSQL, MongoDB, and SQLite. TypeORM allows developers to work with database objects using TypeScript syntax. Here’s an example of a TypeORM entity for a blog post:
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class Post {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
@Column()
body: string;
}
Both Sequelize and TypeORM offer similar features such as query builders, database migrations, and schema validation. However, developers with a background in JavaScript may find Sequelize more familiar, while those who prefer TypeScript may prefer TypeORM.
Overall, both Node.js and Nest.js provide robust database integration options, making it easy for developers to manage data in their applications.
Scalability and Performance: Nest.js vs Node.js
One critical factor to consider when choosing a backend framework is scalability and performance. The ability of a framework to handle high traffic and ensure optimal performance is essential for any web application. In this section, we will compare the scalability and performance capabilities of Nest.js and Node.js.
Scalability
Node.js was designed to be highly scalable, making it the go-to framework for building high-performance web applications. It uses an event-driven architecture that allows it to handle a large number of simultaneous connections efficiently. Node.js also makes it easy to cluster processes across multiple cores, enabling it to scale to meet increasing demands.
Nest.js takes scalability to the next level by building on top of the powerful features of Node.js. It provides additional tools and techniques for building scalable applications, such as the ability to create microservices and use message brokers. Nest.js also includes a built-in module for distributed cache management, making it easier to scale applications across multiple nodes or servers.
Performance
Node.js is known for its excellent performance, thanks to its non-blocking I/O model and event-driven architecture. This enables it to handle a large number of simultaneous connections without blocking the event loop. Node.js also has a small memory footprint, making it ideal for building high-performance applications that require low latency.
Nest.js builds on Node.js’s performance capabilities by providing additional tools and techniques to optimize performance.
For example, Nest.js includes a built-in module for caching, which can significantly improve response times for frequently accessed data. It also provides support for HTTP/2, which can improve the performance of web applications by reducing the latency of requests.
Community Support and Documentation
Choosing the right backend framework can be a daunting task, especially if you’re new to the field. Luckily, both Node.js and Nest.js have a strong and active community of developers who are always ready to help you out.
The Node.js community is vast, with over a million users and more than 60,000 packages available on npm. Developers can easily find online resources, documentation, and support for any question or issue they may encounter. The official Node.js website also offers a documentation library that covers everything from basic syntax to advanced features and modules.
Similarly, the Nest.js community is growing steadily, with over 14,000 stars on GitHub and an active Discord server. The official Nest.js documentation is comprehensive and covers all aspects of the framework, from installation to deployment.
Framework | Community Support | Documentation |
---|---|---|
Node.js | Active and vast | Comprehensive and extensive |
Nest.js | Growing and supportive | Extensive and user-friendly |
Both communities offer great support and documentation resources, but Node.js has the upper hand due to its widespread adoption and longevity, making it easier to find answers to any problem you may encounter. However, Nest.js is catching up quickly and has a growing community that is supportive and helpful.
When it comes to learning resources, both Node.js and Nest.js have a range of tutorials, courses, and books available online. However, since Nest.js is relatively new, the availability of learning resources may be limited.
In terms of community support and documentation, Node.js is the clear winner due to its widespread adoption and longevity. However, Nest.js is not far behind and has a supportive community that is growing rapidly. Regardless of your choice, both frameworks have extensive documentation and a broad range of developers ready to help you tackle any challenge you may encounter.
Choosing the Right Framework: Factors to Consider
Choosing the right backend framework for your development needs is crucial for the success of your project.
Here are some factors to consider when deciding between Nest.js and Node.js:
- Project requirements: Consider the specific requirements of your project, such as scalability, performance, and security. Node.js may be a good fit for projects that require fast and lightweight solutions, while Nest.js may be better suited for larger and more complex projects.
- Team expertise: Evaluate the expertise and experience of your development team. Node.js has been around longer and has a larger developer community, making it a more widely-known and accessible framework. On the other hand, Nest.js may require a steeper learning curve due to its unique architecture and concepts.
- Long-term support: Consider the long-term support and maintenance of the framework. Node.js has a stable and well-established ecosystem, with a large number of libraries and resources. Nest.js is a relatively newer framework but is backed by a strong team of developers and has been gaining popularity in the developer community.
Ultimately, the choice between Nest.js and Node.js depends on your project’s specific needs and the expertise of your development team. Consider the factors above when making your decision.
Nest.js and Node.js in Action: Use Cases
Both Nest.js and Node.js are versatile and widely adopted frameworks for backend development with a wide range of use cases. Here are a few examples:
Node.js
Web Applications: Node.js is a popular choice for developing web applications such as social networking sites, real-time chat applications, and e-commerce platforms.
Its ability to handle multiple connections simultaneously makes it an ideal choice for web applications that require real-time updates and interactions.
Industry | Use Case |
---|---|
Finance | High-frequency trading systems that require real-time processing of large data sets |
Media and Entertainment | Large-scale content distribution systems that can handle high traffic and streaming demands |
Healthcare | Real-time patient monitoring systems that require constant data exchange between devices |
Nest.js
Microservices: Nest.js is ideal for developing microservices, which are independent, small-scale services that work together to form an application. Its modular architecture and dependency injection capabilities make it easy to build and maintain complex microservice systems.
Industry | Use Case |
---|---|
Finance | Payment processing systems that require secure communication between services and fast processing of requests |
E-commerce | Order management and shipping services that require real-time communication and coordination between various modules |
Travel | Booking and reservations systems that require handling multiple requests from different sources simultaneously |
Regardless of the industry or project type, both frameworks offer unique advantages and can be tailored to fit specific development needs.
Final Thoughts
Choosing the right backend framework for your project is crucial for its success. Node.js and Nest.js are two of the most popular frameworks used in backend development today, each with its own strengths and weaknesses.
After exploring the key features, advantages, and use cases of Node.js and Nest.js, it’s clear that both frameworks are capable and powerful tools for developing robust and scalable applications.
However, when it comes to routing capabilities, Nest.js offers a more modern and intuitive syntax, while Express (used in Node.js) provides a more minimalistic and lightweight approach. In terms of database integration, Node.js has a wider range of supported libraries, while Nest.js provides a built-in ORM and TypeORM integration for type-safe and consistent database access.
When it comes to scalability and performance, both frameworks offer tools and techniques to handle high traffic and ensure optimal performance. However, Nest.js has a more modular and structured architecture, making it easier to maintain and scale larger projects in the long run.
Lastly, community support and documentation are essential for any framework, and both Node.js and Nest.js have active and vibrant communities with plenty of documentation and resources available.
Ultimately, the choice between Node.js and Nest.js depends on various factors such as project requirements, team expertise, and long-term support. It’s important to carefully evaluate these factors before making a decision.
Both Node.js and Nest.js offer powerful and flexible options for backend development. Understanding their differences and unique strengths can help developers make a more informed choice when selecting a framework for their projects.
External Resources
https://expressjs.com/en/guide/database-integration.html
FAQ
Q: What is the difference between Nest.js and Node.js?
A: Nest.js is a framework built on top of Node.js that provides additional features and structure for backend development. While Node.js is a runtime environment that allows JavaScript to run on the server-side, Nest.js adds a layer of organization and modularity to the codebase.
Q: What are the advantages of using Node.js?
A: Node.js offers several advantages for backend development. It is based on JavaScript, which makes it easier for frontend developers to transition to backend development. Additionally, Node.js provides non-blocking I/O, allowing for efficient handling of multiple requests and scalability. It also has a vast ecosystem of packages and libraries available for various functionalities.
Q: What are the main features of Nest.js?
A: Nest.js offers features such as dependency injection, modular structure, and robust routing capabilities. It also integrates well with other libraries and frameworks, making it suitable for complex applications. Nest.js leverages decorators and TypeScript, providing developers with a strongly-typed and expressive development experience.
Q: How does routing in Nest.js compare to Express?
A: Express is a minimalist framework for Node.js, while Nest.js provides a more structured and opinionated approach to routing. Nest.js uses decorators to define routes, creating a more organized and intuitive routing system. Express, on the other hand, uses a more traditional syntax for defining routes.
Q: How can Node.js and Nest.js integrate with databases?
A: Both Node.js and Nest.js support various libraries and frameworks for database integration. Node.js offers a wide range of options, including popular libraries like Sequelize and Mongoose. Nest.js leverages the same libraries and provides additional features for easy integration, such as built-in support for dependency injection.
Q: Which framework is more scalable and performant, Nest.js or Node.js?
A: Both Nest.js and Node.js can handle high traffic and ensure optimal performance. However, Nest.js provides additional utilities and architectural patterns for scalability, such as module-based organization and Dependency Injection containers. Depending on the specific needs of your project, either framework can be suitable for achieving scalability and performance.
Q: Is there good community support and documentation for Nest.js and Node.js?
A: Both Nest.js and Node.js have active communities and abundant documentation resources. Node.js, being a widely popular runtime, has a vast community and extensive online resources. Nest.js, being a newer framework, also has a growing community and official documentation that covers its various features and concepts.
Q: What factors should I consider when choosing between Nest.js and Node.js?
A: When choosing between Nest.js and Node.js, consider factors such as your project requirements, team expertise, and long-term support. If you prefer a more structured and opinionated framework with additional features, Nest.js might be a better choice. If you prioritize flexibility and a vast ecosystem, Node.js might be more suitable.
Q: Can you provide some use cases for Nest.js and Node.js?
A: Nest.js is often used in projects that require a modular and organized approach to backend development, such as enterprise applications or large-scale APIs. Node.js, on the other hand, is widely used in various contexts, including real-time applications, microservices, and serverless architectures.
Q: What are the final thoughts on the Nest.js vs Node.js comparison?
A: The choice between Nest.js and Node.js ultimately depends on your specific needs and preferences. Both frameworks offer unique advantages and can be suitable for different types of projects.
Consider the features, community support, and compatibility with your existing tech stack to make an informed decision.
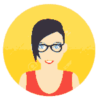
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.