BDD frameworks have gained increasing traction in recent years as one of the most effective ways of developing robust, reliable, and high-quality software. In the world of JavaScript development, using a BDD framework can help to ensure that code is both functional and optimized for performance.
In this article, we will provide an in-depth overview of BDD frameworks in JavaScript, exploring what they are, how they work, and the key benefits they offer.
We will also showcase some of the most popular BDD frameworks for JavaScript and provide some practical tips on how to integrate BDD into your JavaScript development workflow.
Key Takeaways
- BDD frameworks are a critical component of modern web development, ensuring that code is both functional and optimized for performance.
- JavaScript development can greatly benefit from the use of BDD frameworks, which provide improved collaboration, enhanced test coverage, and more reliable code.
- Real-world examples demonstrate the practical applications and benefits of BDD frameworks in JavaScript development.
What is BDD?
First and foremost, BDD, or Behavior-Driven Development, is a software development methodology that emphasizes collaboration between developers, testers, and business stakeholders to ensure that the software being developed meets the desired specifications and fulfills customer needs.
Contrastingly, unlike traditional development approaches, BDD focuses on the behavior of the software rather than solely on its technical details. This methodology involves defining and describing the desired behavior in plain language, creating automated tests to verify that the behavior is implemented correctly, and continuously refining and improving the tests and code based on feedback.
Benefits of BDD Frameworks
Using BDD frameworks in your JavaScript development workflow can bring about a range of benefits, including:
Improved Collaboration | By enabling developers and stakeholders to communicate and clarify requirements through the use of Gherkin syntax, BDD frameworks can foster better collaboration and understanding within a team. |
---|---|
Enhanced Test Coverage | BDD tests focus on the behavior and functionality of specific features, ensuring that all edge cases are tested and leading to higher test coverage and more reliable code. |
More Reliable Code | BDD frameworks encourage developers to write tests before writing the code, leading to more reliable code that meets the desired specifications and is less prone to bugs. |
Additionally, BDD frameworks can help to identify defects earlier in the development cycle, reducing the cost of fixing them and increasing the overall efficiency of the development process.
Popular BDD Frameworks in JavaScript
There are several BDD frameworks available for JavaScript, each with its own unique features and capabilities. Here are some of the most popular ones:
Framework | Description |
---|---|
Jasmine | Jasmine is a popular BDD framework that is easy to get started with. It includes assertion libraries and spies for testing asynchronous code, making it a great choice for JavaScript developers. |
Cucumber.js | Cucumber.js is a BDD framework that enables developers and non-technical stakeholders to work together on testing scenarios. It uses the Gherkin syntax to define scenarios, making it easy to understand and maintain. |
Mocha | Mocha is a flexible BDD framework that allows developers to use any assertion library and any mocking library. It can be used with a variety of browsers and has a rich plugin ecosystem. |
Cypress | Cypress is a newer BDD framework that focuses on end-to-end testing in the browser. It includes a dashboard for monitoring test results and can be used for both manual and automated testing. |
Other popular BDD frameworks include Jest, Protractor, and Nightwatch.js. When selecting a BDD framework for your JavaScript project, it’s important to consider factors like ease of use, flexibility, and the testing capabilities you require.
Getting Started with BDD Frameworks
Integrating a BDD framework into your JavaScript development workflow can seem daunting at first, but with the right guidance, it can be a smooth process. Here is a step-by-step guide to get started:
Step 1: Choose a BDD Framework
The first step is to choose a BDD framework that fits your needs. Jasmine, Cucumber.js, and Mocha are popular choices among JavaScript developers. Consider factors such as ease of use, community support, and integration with other tools.
Step 2: Install the Framework
Once you have chosen a framework, you need to install it in your development environment. You can use a package manager such as npm to install the necessary dependencies and get started.
Step 3: Configure the Framework
Once the framework is installed, you need to configure it to work with your project. This involves setting up a configuration file and defining the test directory and other options.
Step 4: Write the Tests
Now comes the fun part – writing the tests! BDD tests are written in a natural language format, using scenarios and assertions to describe the expected behavior of your code. You can use tools such as Gherkin syntax to write your scenarios in a readable format.
Step 5: Run the Tests
After writing your tests, it’s time to run them! You can use the test runner provided by your framework to execute your tests and get feedback on their success or failure. This is where you can see the benefits of using BDD, as it allows for more comprehensive and reliable testing.
By following these steps, you can quickly and easily integrate a BDD framework into your JavaScript development workflow and start reaping the benefits of improved collaboration, enhanced test coverage, and more reliable code.
Writing BDD Tests in JavaScript
Writing BDD tests in JavaScript involves defining the scenarios and how the system should behave under different conditions. It is essential to ensure that all the acceptance criteria are met by the application. Below are some key steps on how to write BDD tests in JavaScript.
Defining scenarios
Start by defining the different scenarios that need to be tested. This helps to identify the different paths that a user can take while interacting with the system. The scenarios should be defined in simple language that is easy to understand and should cover all possible use cases.
Using assertions
Assertions are used to check that the expected behavior of the system matches the actual behavior. Assertions can be used to check that certain conditions are true or false, that a certain value is returned, or that certain events have occurred. Common assertion libraries used for JavaScript BDD frameworks include Chai and Expect.js.
Organizing test suites
Tests should be organized into logical groups to make it easier to manage and maintain them. Tests can be organized according to their functional areas, use cases, or scenarios. The most commonly used structure for organizing tests is the “describe” block, which groups related tests together.
"describe('User Management', function() { describe('Login', function() { it('should log in a valid user', function() { // test code here }); it('should not log in an invalid user', function() { // test code here }); }); describe('Registration', function() { it('should register a valid user', function() { // test code here }); it('should not register an invalid user', function() { // test code here }); }); });"
The above example shows how tests can be organized into different categories using the “describe” block.
Writing BDD tests in JavaScript can be a complex process, but it is essential for ensuring the quality and reliability of your code. By following best practices and using the right tools, you can develop effective BDD tests that enhance collaboration, improve test coverage, and lead to more reliable code.
Best Practices for BDD in JavaScript
When implementing BDD in JavaScript, it’s important to follow certain best practices to ensure that your tests are effective and maintainable. Here are some tips to keep in mind:
Firstly, keep your tests atomic: Atomic tests are small and focused, making them easier to understand and maintain. It’s essential to avoid creating monolithic tests that try to cover too much functionality at once.
Secondly, write clear and descriptive scenarios: Scenarios should describe a specific behavior in plain language, making it easy for others to understand what the test is covering.
Furthermore, organize your tests effectively: Group tests logically by using tags or folders, making them easier to find and run.
Moreover, avoid redundant tests: Only write tests for specific behaviors that are not already covered by other tests. It’s crucial to avoid duplicating tests unnecessarily.
Additionally, maintain your test suite: Update your tests regularly, ensuring they reflect any changes that have been made to the codebase. Consistency is key; keep your tests clean and well-organized to make them easier to maintain in the long term.
Next, use code reusability: Write code that is reusable across multiple tests, reducing duplication and making your tests easier to maintain.
Lastly, use mocks and stubs: Utilize mocks and stubs to isolate your tests from external dependencies, making them more reliable and easier to run in a variety of environments.
By following these best practices, you can ensure that your BDD tests are effective, maintainable, and scalable, helping you to build high-quality JavaScript applications that meet the needs of your end users.
Integrating BDD with Continuous Integration
Integrating BDD frameworks with continuous integration (CI) tools like Jenkins or CircleCI can significantly enhance the efficiency and accuracy of your testing process. By automating the testing process, you can detect and address potential issues much faster, reducing the risk of errors or delays.
Here are some key steps to follow when integrating BDD with CI:
To begin with, write effective tests: Ensure that your tests are well-designed, accurately reflecting the behavior of your application and providing comprehensive coverage of relevant functionality.
Subsequently, configure CI tools: Choose a CI tool that supports BDD frameworks and configure it to run your tests automatically on each code push or pull request.
Following that, set up reporting: Use a reporting tool like Allure or Cucumber to generate detailed reports on your test results, including test failure analysis and performance metrics.
In conclusion, monitor test results: Regularly monitor your test results to identify any issues or trends, and fine-tune your testing approach accordingly.
By following these best practices, you can ensure that BDD frameworks and CI work together seamlessly, enabling you to quickly and accurately test your JavaScript applications and maintain high code quality.
Examples of BDD Frameworks in JavaScript
Implementing BDD frameworks in JavaScript is not only a best practice but a necessity in today’s web development landscape. Here are some real-world examples of projects that have successfully incorporated BDD frameworks:
Project | Framework Used | Description |
---|---|---|
Netflix | Jasmine | The world’s leading streaming service uses Jasmine to ensure the reliability and scalability of their platform. Their automated tests check everything from login functionality to video playback. |
PayPal | Cucumber.js | PayPal uses Cucumber.js to create executable specifications that capture the requirements of their application in readable and maintainable scenarios. This has helped streamline their development process and improve collaboration between teams. |
Jest | Jest is Facebook’s open-source testing framework used to test all their JavaScript tools and libraries. It comes with built-in mocking capabilities and parallel test execution, making it a top choice for developers looking to optimize their testing procedures. |
These are just a few examples of the many successful implementations of BDD frameworks in JavaScript. By following best practices and leveraging the right tools, developers can ensure the quality and reliability of their code while maximizing efficiency and collaboration.
Conclusion – BDD Frameworks in JavaScript
BDD frameworks have emerged as a crucial component of modern web development, particularly in the JavaScript ecosystem. By enabling developers to write more reliable code, collaborate effectively, and achieve better test coverage, BDD frameworks can help streamline the development process and improve the quality of web applications.
Moreover, throughout this article, we have explored the key concepts and principles of BDD, the benefits and popular frameworks available, and best practices for implementing BDD in JavaScript. Additionally, we have provided real-world examples of successful BDD adoption, highlighting the practical applications of this approach.
In conclusion, we strongly encourage readers to explore and implement BDD frameworks in their own JavaScript development workflows, leveraging the guidance and best practices provided in this article. By embracing BDD, developers can improve their code quality, streamline their processes, and deliver more effective web applications.
FAQs – BDD Frameworks in JavaScript
Understanding BDD Frameworks in JavaScript
What are BDD frameworks in JavaScript?
BDD frameworks in JavaScript empower developers to apply the behavior-driven development methodology in their projects. They structure the way tests are written and boost collaboration among developers, testers, and stakeholders.
Distinguishing BDD from Other Methodologies
How does BDD stand out from other development methodologies?
BDD uniquely centers on defining and verifying a system’s behavior from the user’s viewpoint. It champions collaboration, readability, and thorough documentation, streamlining the understanding and maintenance of tests.
Advantages of BDD Frameworks in JavaScript
Why should developers use BDD frameworks in JavaScript?
Employing BDD frameworks in JavaScript brings forth perks like heightened team collaboration, superior test coverage, fortified code reliability, and a closer alignment to business needs. These tools guarantee that software aligns with the intended behavior, optimizing the development process.
Popular BDD Frameworks in the JavaScript Ecosystem
Which BDD frameworks are prevalent in JavaScript?
Notable BDD frameworks in JavaScript encompass Jasmine, Cucumber.js, and Mocha. Each offers distinct features that aid in crafting and running behavior-driven tests in JavaScript projects.
Kickstarting Your BDD Journey in JavaScript
How can one initiate their journey with BDD frameworks in JavaScript?
To embark on using BDD frameworks in JavaScript, select an apt framework for your endeavor. Subsequently, adhere to a comprehensive guide to weave the framework into your development routine. This encompasses framework installation, configuration, and test writing using BDD principles.
Crafting BDD Tests in JavaScript
What steps are involved in penning BDD tests in JavaScript?
The art of scripting BDD tests in JavaScript entails outlining scenarios or user narratives, constructing test cases with BDD semantics, employing assertions for behavior verification, and clustering tests into suites. This guarantees tests mirror the system’s intended behavior and run efficiently.
Best Practices in BDD for JavaScript
What guidelines should one follow for BDD in JavaScript?
For a fruitful BDD execution in JavaScript, it’s pivotal to adhere to best practices. These include segmenting tests into coherent scenarios, routinely updating test suites, capitalizing on code reusability, and fostering transparent communication among developers, testers, and stakeholders.
Integrating BDD with Continuous Integration
How do BDD frameworks complement continuous integration?
BDD frameworks effortlessly merge with continuous integration instruments like Jenkins or CircleCI. By embedding BDD tests into the CI/CD pipeline, developers can automate testing, garner swift feedback on code alterations, and ascertain consistent system behavior.
Real-World Applications of BDD Frameworks in JavaScript
Can you cite practical instances of BDD frameworks in JavaScript?
Tangible applications of BDD frameworks in JavaScript span projects like e-commerce platforms, digital banking systems, and mobile apps. These ventures have adeptly utilized BDD frameworks to ensure software aligns with the stipulated behavior and enhances user experience.
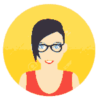
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.