JavaScript is an essential tool for optimizing enterprise operations through efficient, scalable, and customizable coding solutions. Its ability to handle complex business rules makes it a valuable asset for developers and businesses alike. In this article, we will explore the concept of JavaScript business logic and its importance in modern-day enterprise-level projects.
Implementing JavaScript business logic involves understanding how to define the behavior and logic of a business process, using various logic components such as conditionals, loops, and functions.
Understanding JavaScript Business Rules
JavaScript business rules are an essential component of creating efficient and optimized enterprise operations. These rules define the logic and behavior of a business process, providing a blueprint for automated decision-making and data processing.
Business rules in JS can take many forms, from simple conditional statements to complex algorithms involving multiple variables and functions. Here is an example of a basic business rule in JS:
If the user is logged in, display a personalized greeting.
if (loggedIn == true) {
console.log("Welcome back, " + userName + "!");
}
In this example, the business rule checks whether the user is logged in and, if so, displays a personalized greeting using the user’s name.
Business rules can be created using various logic components available in JavaScript, such as conditionals, loops, and functions. These components allow for flexible and scalable coding solutions that can handle complex business requirements.
When creating business rules in JS, it is essential to consider the specific needs and requirements of the enterprise project. This includes identifying the data sources, defining the decision-making criteria, and selecting the appropriate logic components.
Example: Using Framework X to Implement Business Rules in JS
Framework X offers powerful capabilities for implementing business rules in JS. Here is an example of how to use Framework X to create a business rule for calculating a discount based on the total purchase amount:
If the total purchase amount is over $100, apply a 10% discount.
Framework X Code: function calculateDiscount(totalPurchaseAmount) { if (totalPurchaseAmount > 100) { return totalPurchaseAmount * 0.1; } }
This business rule uses a standard function to calculate the discount based on the total purchase amount. The if statement checks whether the total purchase amount is over $100, and if so, returns the discounted amount.
By using Framework X, this business rule can be easily integrated into the enterprise project, providing a scalable and efficient solution for processing data and automating decision-making.
Exploring Logic Components in JavaScript
JavaScript provides a range of logic components that can be used to create efficient, scalable, and customizable business logic solutions. These components include:
- Conditionals
- Loops
- Functions
These components can be combined in various ways to create complex logic solutions that meet the needs of businesses.
Conditionals
Conditionals are used to execute different blocks of code based on certain conditions. The most common type of conditional is the “if statement”, which allows you to execute a block of code if a condition is true. For example:
// If the temperature is above 90 degrees, output "It's really hot!"
if (temperature > 90) {
console.log("It's really hot!");
}
Other types of conditionals include the “else statement”, which allows you to execute a block of code if the condition in the “if statement” is not true, and the “else if statement”, which allows you to check additional conditions if the condition in the “if statement” is not true.
Loops
Loops are used to execute a block of code multiple times. The most common type of loop is the “for loop”, which allows you to execute a block of code a specific number of times. For example:
// Output the numbers from 1 to 10
for (var i = 1; i
console.log(i);
}
Other types of loops include the “while loop”, which allows you to execute a block of code as long as a condition is true, and the “do-while loop”, which is similar to the “while loop”, but executes the block of code at least once, even if the condition is initially false.
Functions
Functions are reusable blocks of code that perform specific tasks. They can be called from other parts of the code, allowing for more efficient and modular code. For example:
// Define a function that adds two numbers together
function addNumbers(number1, number2) {
return number1 + number2;
}
// Call the function and output the result
console.log(addNumbers(5, 10));
Functions can also be nested, allowing for more complex logic solutions. They can be anonymous or named, and can be used as callbacks, which are functions that are passed as arguments to other functions.
By leveraging these logic components, businesses can create robust and efficient business logic solutions in JavaScript.
Comparing JavaScript Frameworks: A Look at Framework X
When it comes to implementing efficient and scalable JavaScript business logic, choosing the right framework can make all the difference. Framework X is a popular choice for developers due to its robust features and ease of use. Let’s take a look at how Framework X compares to other popular JavaScript frameworks in terms of implementing business logic.
Code Example: Business Rule Implementation
One of the key components of business logic is the implementation of business rules. In Framework X, this can be accomplished using a simple, yet powerful syntax:
if (customerAge >= 18) {
allowPurchase();
}
This code block checks the age of a customer and allows them to make a purchase if they are over 18. This is a basic example, but it illustrates the simplicity and ease of implementing business rules in Framework X.
Code Example: Conditionals
Conditionals are an important component of business logic, allowing developers to create branching logic based on specific conditions. Framework X offers a variety of conditional statements, such as:
Statement | Description |
---|---|
if…else | Executes code if a condition is true and another code block if it is false. |
switch | Evaluates an expression and executes code based on the value. |
ternary operator | Provides shorthand syntax for simple conditional statements. |
Here’s an example of a switch statement in Framework X:
switch (dayOfWeek) {
case "Monday":
doSomething();
break;
case "Tuesday":
doSomethingElse();
break;
default:
doNothing();
}
This code block evaluates the value of the dayOfWeek variable and executes different code based on the result. It’s just one example of how Framework X provides powerful conditional statements for implementing business logic.
Code Example: Functions
Functions are the building blocks of business logic, allowing developers to create reusable blocks of code. In Framework X, functions can be easily defined and called:
function calculateTotal(price, quantity) {
return price * quantity;
}
let total = calculateTotal(10, 5);
This code block defines a function called calculateTotal that takes in two parameters, price and quantity. It then multiplies the two values together and returns the result. The function can be called anywhere in the code, allowing for easy reuse and maintainability.
Overall, Framework X offers a wide range of features and tools for implementing efficient and scalable business logic. However, it’s important to consider other popular frameworks and their capabilities before making a final decision.
Comparing JavaScript Frameworks: A Look at Framework Y
Framework Y is another popular JavaScript framework with robust capabilities for implementing business logic. One of the key strengths of Framework Y is its modularity, which allows developers to reuse components and simplify code maintenance.
Here’s an example of how Framework Y can implement a simple business rule:
Framework Y Code |
---|
const age = 25; if(age > 18){ console.log(“You are an adult”); } else { console.log(“You are a minor”); } |
In this example, Framework Y uses a conditional statement to determine whether an individual is an adult or a minor based on their age. Framework Y’s syntax is highly readable and can simplify complex business logic.
Another advantage of Framework Y is its lightweight architecture, which allows for fast and efficient processing of complex logic. Here’s an example of how Framework Y can perform a loop:
Framework Y Code |
---|
const numbers = [1, 2, 3, 4, 5]; for(let i = 0; i < numbers.length; i++){ console.log(numbers[i] * 2); } |
In this example, Framework Y uses a for loop to multiply each element in the numbers array by 2. Framework Y’s concise syntax and efficient processing make it ideal for handling large-scale business logic.
Overall, Framework Y is a versatile framework that excels in modularity and lightweight architecture. While it may not have the same widespread adoption as Framework X, it offers unique advantages for implementing complex business logic.
Best Practices for Implementing JavaScript Business Logic
Implementing JavaScript business logic is a crucial step in optimizing enterprise operations. To ensure that your coding solutions are efficient, maintainable, and scalable, it is essential to follow industry best practices. Here are some tips to keep in mind:
1. Keep Your Code Simple and Readable
When implementing JavaScript business logic, it’s essential to keep your code as simple and readable as possible. This will make it easier to maintain and scale your code over time. Use descriptive variable and function names, comments, and white space to make your code more understandable.
For example:
Bad Code Good Code var x = 3; var age = 3; if(x>2){ /*do something*/ } if(age > 2){ /*do something*/ }
2. Use Object-Oriented Programming
Object-oriented programming is the most effective way to implement JavaScript business logic. It allows you to encapsulate code into reusable objects, making it easier to maintain and scale your code. Use classes, objects, and inheritance to organize your code and create modular solutions.
For example:
class Employee {
constructor(name, id, salary) {
this.name = name;
this.id = id;
this.salary = salary;
}
getSalary() {
return this.salary;
}
}
class Manager extends Employee {
constructor(name, id, salary, bonus) {
super(name, id, salary);
this.bonus = bonus;
}
getSalary() {
return super.getSalary() + this.bonus;
}
}
const john = new Manager('John', 123, 5000, 1000);
console.log(john.getSalary());
// Output: 6000
3. Use Promises and Async/Await
Asynchronous programming is a crucial concept in JavaScript business logic. It allows your code to process multiple tasks simultaneously, leading to faster and more efficient operation. Use promises and async/await to handle asynchronous tasks and improve code readability.
For example:
function fetchData() { return new Promise((resolve, reject) =>
{ //fetch data from API if(data) { resolve(data); } else { reject(new Error
('Failed to fetch data')); } }); } async function displayData()
{ try { const data = await fetchData(); console.log(data); }
catch(error) { console.log(error); } } displayData();
4. Test Your Code Thoroughly
Thorough testing is the key to ensuring the reliability and accuracy of your JavaScript business logic. Use testing frameworks like Jest, Mocha, or Jasmine to test your code and catch errors. Write unit tests for individual functions and integration tests for the entire system.
For example:
function multiply(a, b) { return a * b; } describe('multiply', () => { it('multiplies two numbers', () => { expect(multiply(2, 3)).toBe(6); expect(multiply(1, 0)).toBe(0); expect(multiply(-1, -1)).toBe(1); }); });
By following these best practices, you can ensure that your JavaScript business logic is efficient, maintainable, and scalable- leading to optimized enterprise operations.
Examples of JavaScript Business Logic
JavaScript business logic is not just a theoretical concept; it has proven to be highly effective in optimizing enterprise operations across a wide range of industries. Let’s take a look at some real-world applications of JavaScript business logic.
E-commerce
E-commerce websites rely heavily on JavaScript business logic to provide a seamless shopping experience to their customers. From shopping carts to checkout processes, JavaScript business logic ensures that all operations are efficient and error-free. For example, JavaScript can be used to calculate discounts, validate coupon codes, and update inventory in real-time.
Code example:
// calculate discount
let price = 100;
let discount = 0.25;
let discountedPrice = price - (price * discount);
console.log(discountedPrice); // output: 75
Healthcare
JavaScript business logic plays a crucial role in healthcare applications, from patient care to record-keeping. For example, JavaScript can be used to validate patient information, track medication schedules, and automate billing processes. This not only saves time but also reduces the risk of errors, ensuring that patients receive optimal care.
Code example:
// validate patient age
let age = 25;
if (age 65) {
console.log("Sorry, this patient is not eligible for this treatment.");
} else {
console.log("This patient is eligible for this treatment.");
}
Finance
JavaScript business logic is widely used in the finance industry to analyze data and make informed decisions. It can be used to calculate interest rates, track investments, and generate financial reports. This ensures that financial institutions have accurate and up-to-date information, enabling them to make sound business decisions.
Code example:
// calculate compound interest
let principal = 1000;
let rate = 0.05;
let time = 10;
let compoundInterest = principal * Math.pow(1 + rate, time);
console.log(compoundInterest); // output: 1628.89
As these examples demonstrate, JavaScript business logic is a critical component of modern enterprise operations. By leveraging its power and versatility, businesses can optimize their processes, reduce errors, and enhance customer satisfaction.
Scaling JavaScript Business Logic for Enterprise-Level Projects
Implementing JavaScript business logic in enterprise-level projects can be complex and challenging. As the project grows in size and complexity, so does the need for efficient and scalable logic components.
Here are some strategies for scaling JavaScript business logic to meet the demands of enterprise-level projects:
Break Down Business Rules into Smaller Components
Divide complex business rules into smaller, more manageable components. This approach can simplify the coding process, make it easier to maintain and debug, and improve the scalability of the logic. Use functions to encapsulate these smaller components and ensure that they can be reused throughout the code.
Use Design Patterns
Design patterns are reusable solutions to common programming problems. Using design patterns can improve the scalability of a coding project, as well as its maintainability and readability. Some examples of design patterns that are commonly used for JavaScript business logic include the observer pattern, the factory pattern, and the strategy pattern.
Ensure Optimal Performance
Optimize the performance of JavaScript business logic by minimizing the use of expensive operations and by caching expensive computations. Additionally, avoid using blocking code that can cause the application to become unresponsive. Instead, use asynchronous code that allows the application to remain responsive while performing long-running operations.
Use a Framework or Library
Using a framework or library can simplify the process of scaling JavaScript business logic. Frameworks and libraries can provide pre-built components, which can reduce the amount of code that needs to be written. Additionally, frameworks and libraries can provide performance optimizations and other scalability features.
When choosing a framework or library for enterprise-level projects, consider the specific needs of the project and the requirements of the business. Some popular JavaScript frameworks for enterprise-level projects include Angular, React, and Vue.
By implementing these strategies, businesses can scale their JavaScript business logic to meet the demands of enterprise-level projects. This can result in improved efficiency, reduced costs, and a better user experience.
Final Thoughts
JavaScript business logic is a crucial element in optimizing enterprise operations. By providing efficient, scalable, and customizable coding solutions, businesses can improve their productivity and profitability.
External Resources
https://learn.microsoft.com/en-us/power-apps/developer/model-driven-apps/client-scripting
FAQ
Q: What is JavaScript business logic?
A: JavaScript business logic refers to the implementation of coding solutions using the JavaScript programming language to optimize enterprise operations. It involves defining the logic and behavior of business processes through efficient, scalable, and customizable code.
Q: Why is JavaScript business logic important?
A: JavaScript business logic is important because it allows businesses to streamline processes, automate tasks, and improve overall efficiency. By implementing logic components in JavaScript, businesses can create customized workflows tailored to their specific needs, resulting in improved productivity and profitability.
Q: What are logic components in JavaScript?
A: Logic components in JavaScript refer to language constructs such as conditionals, loops, and functions that enable the implementation of business rules and logic. These components allow for the execution of specific actions based on defined conditions, iterations over data sets, and the organization of code into reusable and modular units.
Q: How do I compare JavaScript frameworks for implementing business logic?
A: When comparing JavaScript frameworks for implementing business logic, it is important to consider factors such as ease of use, performance, community support, and compatibility with existing systems. Additionally, examining code examples and understanding the strengths and weaknesses of each framework can help in making an informed decision.
Q: What are the best practices for implementing JavaScript business logic?
A: Some best practices for implementing JavaScript business logic include writing clean and modular code, using meaningful variable and function names, documenting the code, handling errors effectively, and optimizing performance. It is also beneficial to follow coding standards and conventions and leverage tools and frameworks that promote code quality and maintainability.
Q: How can I scale JavaScript business logic for enterprise-level projects?
A: Scaling JavaScript business logic for enterprise-level projects requires careful planning and consideration of factors such as performance optimization, code modularization, and handling large data sets. Applying architectural patterns, implementing caching mechanisms, and leveraging cloud services can also help in achieving scalability and performance.
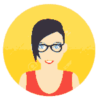
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.