Developers have been taught to follow JavaScript best practices and tips to write efficient and reliable code for years. However, after over 40 years of experience in the field, blindly following these guidelines could limit your potential as a developer.
I will outline my viewpoint in this article and provide supporting evidence and arguments.
Coding Conventions Can Stifle Creativity
As developers, we’re often told to follow coding conventions to ensure our code is clean, readable, and easy to maintain. But in reality, these conventions can often stifle our creativity and prevent us from exploring new approaches to coding.
For example, take the commonly used convention of placing curly braces on their lines in JavaScript. While this may make the code more readable, it can also limit our ability to write concise, expressive code.
By forcing us to use extra lines and indentation, this convention can make it harder to write code that is both elegant and efficient.
Furthermore, strict adherence to coding conventions can also lead to a culture of conformity, where developers are discouraged from thinking outside the box and exploring new approaches to coding.
Our culture of innovation and creativity can be fostered by challenging these conventions and exploring new code-writing methods.
Counterargument: But isn’t following coding conventions essential for maintaining consistency and readability in a team environment?
While it’s true that consistency is essential in a team environment, blindly following conventions without questioning their relevance can lead to a lack of creativity and innovation.
Instead, by encouraging developers to think critically about these conventions and explore new approaches to coding, we can foster a culture of creativity and innovation while maintaining consistency and readability.
Less Code Can Be More Efficient
One of the most common JavaScript best practices is to write code that is as concise and efficient as possible. However, this can often lead to a culture of code golf, where developers prioritize writing the fewest lines of code over the readability and maintainability of that code.
But in reality, less code can often be more efficient than overly complex and convoluted code. By embracing a minimalist approach to coding, we can write code that is not only more efficient but also easier to read, debug, and maintain.
For example, consider using functional programming concepts like higher-order functions and closures.
By using these techniques to write code that is more declarative and expressive, we can often write code that is both more concise and more efficient than code that relies on imperative programming techniques like loops and conditionals.
Counterargument: But what about the need for robust error handling and defensive coding?
While it’s true that error handling and defensive coding are essential, it’s also possible to write code that is both concise and robust.
By embracing a minimalist approach to coding and focusing on the core functionality of our code, we can often write code that is not only more efficient but also more reliable and easier to maintain in the long term.
Functional Programming Over Object-Oriented Programming
Object-oriented programming has been the dominant paradigm in software development for many years. However, in recent years, functional programming has gained popularity as a more efficient and elegant approach to writing code.
Functional programming emphasizes using pure functions, immutability, and higher-order functions to write more declarative and expressive code than traditional imperative code.
By avoiding side effects and shared mutable states, functional code is often more predictable, more accessible to reason about, and more resistant to bugs and errors.
In contrast, object-oriented programming can often lead to complex, convoluted code that is difficult to understand and maintain. By relying on inheritance, mutable states, and complex class hierarchies, object-oriented code can quickly become unwieldy and hard to maintain.
Counterargument: Don’t object-oriented principles like encapsulation and polymorphism make code more modular and reusable?
While it’s true that object-oriented principles can make code more modular and reusable, functional programming techniques like higher-order functions and composition can achieve the same goals more elegantly and efficiently.
By embracing functional programming, developers can write more concise, expressive, and reliable code while maintaining modularity and reusability.
Avoid Frameworks and Libraries Whenever Possible
One of the most common JavaScript best practices is to use frameworks and libraries to simplify and speed up the development process. However, this can often lead to a culture of over-dependence on third-party tools and a lack of understanding of the underlying technology.
Frameworks and libraries can also introduce unnecessary complexity and bloat to our code, slowing performance and making it harder to debug and maintain. In some cases, they limit the flexibility and customization of our code.
Instead, it’s essential to deeply understand the underlying language and its features and write code that is as lean and optimized as possible. By avoiding frameworks and libraries whenever possible, we can write code that is more efficient, more customizable, and more maintainable in the long term.
For example, consider using jQuery, a popular JavaScript library that simplifies DOM manipulation. While jQuery can be useful for streamlining specific tasks, it can also introduce unnecessary overhead and limit the flexibility of our code.
Using native JavaScript methods and features to manipulate the DOM, we can write more efficient, flexible, and customizable code.
Counterargument: But what about the need for cross-browser compatibility and consistency?
While cross-browser compatibility is essential, achieving this without relying on external frameworks and libraries is often possible.
By understanding the underlying technologies and using feature detection and progressive enhancement techniques, we can write code that works well across different browsers and devices without introducing unnecessary dependencies.
Don’t Be Afraid to Break the Rules
Many JavaScript best practices are based on well-established conventions and standards developed over time. However, blindly following these rules can sometimes stifle creativity and innovation.
While it’s essential to understand and follow best practices when writing code, it’s also important to recognize when these rules may be holding us back. Sometimes, breaking the rules can lead to new and innovative solutions that would not be possible within the constraints of conventional wisdom.
For example, consider the use of unusual variable names or unconventional syntax. While these may violate established coding conventions, they can make our code more readable and expressive in certain situations.
Similarly, experimenting with new and unconventional techniques or frameworks can lead to breakthroughs and innovations that are impossible within the confines of established best practices.
Counterargument: But isn’t it risky to break the rules? Won’t it lead to unpredictable behavior and hard-to-maintain code?
While it’s true that breaking the rules can be risky, it’s also important to recognize that all coding involves some degree of risk.
By taking calculated risks and experimenting with new techniques and approaches, we can gain valuable experience and knowledge that can help us write better code in the long term. Moreover, we can better respond to changing requirements and new challenges by staying flexible and adaptable.
Code Comments Can Be Harmful
One of the most widely recommended best practices in JavaScript development is to include comments in our code to help explain its purpose and functionality. However, too many comments can harm our code, leading to confusion, redundancy, and unnecessary clutter.
When we rely too heavily on comments to explain our code, it can indicate a need for more clarity and organization in our code itself. In many cases, it’s better to focus on writing clear, well-organized, self-explanatory code rather than relying on comments to do the work for us.
Moreover, comments can become outdated or misleading over time, especially if they need to be kept up-to-date with changes to the code. This can lead to confusion and errors, making our code more challenging to maintain and debug.
Instead, writing clear, concise, and self-explanatory code is essential. Comments are used sparingly and only when necessary to clarify particularly complex or obscure functionality.
For example, consider using descriptive variable names and function names to help explain their purpose and functionality. By choosing clear, descriptive names, we can make our code more readable and self-explanatory, reducing the need for excessive comments.
Counterargument: What about when working with large, complex codebases or collaborating with other developers? Don’t comments become more necessary in these situations?
While comments can be helpful in certain situations, it’s essential to recognize that they should not be a substitute for clear, well-organized code.
In large, complex codebases or collaborative projects, it’s often better to focus on writing modular, well-organized, and self-explanatory rather than relying on excessive comments to explain its purpose and functionality.
By writing code that is easy to read and understand, we can reduce the need for excessive comments while making our code more maintainable and extensible in the long term.
Wrapping up about JavaScript Best Practices
Following JavaScript best practices and tips is a safe and reliable approach to development. However, by blindly adhering to these guidelines, developers may limit their creativity, innovation, and efficiency potential.
JavaScript developers can push the boundaries of what is possible by challenging the status quo and embracing experimentation and exploration. Remember, the best practices of today may be the constraints of tomorrow.
So feel free to break the rules and explore new approaches to writing powerful and efficient code.
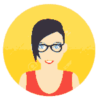
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.