JavaScript and jQuery are two of the most popular technologies used for front-end web development. They enable developers to create highly interactive and dynamic web applications that provide a seamless user experience. In this article, we will explore the fundamentals of JavaScript and jQuery, their features, and how they can be used to create powerful web applications.
Key Takeaways
- JavaScript and jQuery are essential for creating interactive front-end web applications.
- JavaScript is a programming language that enables developers to add interactivity and responsiveness to web pages.
- jQuery is a JavaScript library that simplifies coding and provides a set of powerful tools for front-end development.
Understanding JavaScript and its Role in Web Development
JavaScript is a programming language used primarily for creating dynamic and interactive web pages. By adding interactivity to web pages, JavaScript has become an essential part of modern web development.
JavaScript is executed by web browsers and can be used to manipulate the Document Object Model (DOM), the internal representation of a web page. This manipulation allows developers to create dynamic effects, user interfaces, and other interactive features.
JavaScript Features | Description |
---|---|
Interactivity | JavaScript allows for interactive web elements, such as animation and user input validation. |
DOM Manipulation | JavaScript enables developers to dynamically manipulate the DOM to create responsive and user-friendly web pages. |
Asynchronous Execution | JavaScript’s asynchronous execution allows web pages to perform non-blocking operations, improving performance and user experience. |
Using JavaScript, web developers can also perform asynchronous operations, such as loading content from a server without reloading the entire web page. This technique is known as Asynchronous JavaScript and XML (AJAX).
Understanding JavaScript is essential for any front-end web developer. In the next section, we will explore jQuery, a powerful JavaScript library that simplifies web development and makes it easier to create rich and interactive web applications.
Exploring jQuery and its Features
jQuery is a fast, small, and feature-rich JavaScript library designed to simplify front-end web development. It provides a robust set of tools and libraries that allows developers to manipulate HTML documents, handle events, create animations, and facilitate the development of AJAX applications with ease.
DOM Manipulation
One of the most powerful features of jQuery is its ability to manipulate the Document Object Model (DOM), which is a programming interface for web documents. jQuery allows developers to select and manipulate any element in the DOM tree, including HTML tags, classes, attributes, and more. With jQuery, you can dynamically add, remove, and modify content on a web page.
Task | Code |
---|---|
Select an element by its ID | $(“#myElement”) |
Select all elements with a class name | $(“.myClass”) |
Select all elements of a particular type | $(“p”) |
Event Handling
jQuery simplifies event handling by providing a unified API to handle different types of events, such as click, hover, and keypress. With jQuery, you can easily bind event handlers to DOM elements and trigger custom events as well.
“The event handling in jQuery is really simple and clean. It’s reduced a lot of our code.” – John Resig, Creator of jQuery
AJAX
jQuery’s AJAX API makes it easy to perform asynchronous HTTP requests and update web page content without requiring a full page reload. With jQuery, you can fetch data from a server, send data to a server, and handle HTTP responses with a few lines of code.
- Make an AJAX request:
$.ajax({ url: "data.php", success: function(data){ /* handle the response */ } });
- Load data from a server:
$("#result").load("data.html");
- Send data to a server:
$.post("save.php", { name: "John", age: 30 });
Getting Started with JavaScript and jQuery
Before diving into the world of JavaScript and jQuery, it’s important to set up your development environment and understand the basic syntax and concepts of these technologies. Here’s a step-by-step guide to help you get started:
Setting up your Development Environment
The first step to start creating web applications with JavaScript and jQuery is to set up your development environment. You will need a text editor and a web browser to write and test your code. Here are some popular text editors that work well with JavaScript and jQuery:
Text Editor | Description |
---|---|
Visual Studio Code | A lightweight and powerful editor with built-in debugging |
Sublime Text | A fast and flexible editor with a simple user interface |
Atom | A customizable editor with a large library of plugins |
You can also use a web browser like Google Chrome or Mozilla Firefox to test your code. Both of these browsers have built-in developer tools that allow you to inspect and debug your code.
Understanding Basic Syntax and Concepts
JavaScript and jQuery are both built on the same basic programming concepts, so it is essential to have a good understanding of these concepts before writing any code. Here are some of the key concepts to keep in mind:
- Variables: Variables are used to store data and can be declared using the
var
keyword. - Functions: Functions are reusable blocks of code that perform a specific task.
- Conditional Statements: Conditional statements allow you to control the flow of your code based on different conditions.
- Loops: Loops allow you to repeat a set of instructions multiple times.
- Events: Events are actions that happen on a web page, such as clicking a button or submitting a form.
Having a solid understanding of these concepts will make it easier to write and understand JavaScript and jQuery code.
DOM Manipulation with JavaScript and jQuery
The Document Object Model (DOM) is a hierarchical tree-like structure that defines how HTML elements are structured and organized on a web page. Manipulating the DOM with JavaScript and jQuery is essential for creating dynamic and interactive web pages.
One of the main advantages of jQuery is its ability to simplify DOM manipulation. With jQuery, you can select HTML elements using a wide range of selectors and then modify them using a variety of methods.
Selectors | Methods |
---|---|
$(“selector”) | .html() |
$(“#id”) | .text() |
$(“.class”) | .attr() |
Using these selectors and methods, you can dynamically modify the content, attributes, and styles of HTML elements on your web page. For example, you can change the text of a heading, add a new list item, or animate a button when a user clicks on it.
DOM manipulation is also essential for creating responsive web pages. With JavaScript and jQuery, you can detect changes in the size and orientation of a web page and then adjust the layout accordingly. For example, you can hide certain elements on a small screen or move them to a different location.
Overall, DOM manipulation is a crucial skill for any web developer, and JavaScript and jQuery provide powerful tools for making it easier and more efficient.
Event Handling and AJAX with JavaScript and jQuery
One of the key benefits of using JavaScript and jQuery in web development is their ability to handle user events and make asynchronous requests using AJAX. With these tools, developers can create interactive web applications that respond to user input and update content without requiring page refreshes.
Event Handling
JavaScript and jQuery provide a powerful set of tools for handling user events, such as mouse clicks, keyboard inputs, and touch gestures. These events can trigger custom functions that modify the behavior or appearance of web pages in response to user actions.
A common technique for event handling is to use the addEventListener()
method in JavaScript, or the on()
method in jQuery, to bind functions to specific events on HTML elements. For example, you can use the following code to change the background color of a button when it is clicked:
<button id="myButton">Click Me</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
document.body.style.backgroundColor = "red";
});
</script>
In this example, the addEventListener()
method is used to bind an anonymous function to the “click” event on the button with the ID “myButton”. When the button is clicked, the function changes the background color of the body element to red.
AJAX
AJAX (Asynchronous JavaScript and XML) is a technique for making HTTP requests from web pages without requiring full page refreshes. This allows web applications to update content dynamically without interrupting the user experience.
jQuery provides a number of methods for making AJAX requests, such as $.ajax()
, $.get()
, and $.post()
. These methods allow developers to send and receive data to and from web servers without reloading the entire page.
For example, you can use the following code to make a GET request to a web server and display the response in a div element:
<div id="myDiv"></div>
<script>
$.get("https://example.com/data.json", function(data) {
$("#myDiv").text(data);
});
</script>
In this example, the $.get()
method is used to make a GET request to the URL “https://example.com/data.json”. When the response is received, the anonymous function passed as the second argument is called, and the data is displayed in the div element with the ID “myDiv” using the text()
method.
With event handling and AJAX, JavaScript and jQuery provide a powerful set of tools for creating interactive and responsive web applications. By responding to user actions and updating content dynamically, developers can create engaging user experiences that rival those of native desktop or mobile applications.
Animations and Effects with jQuery
In today’s web development landscape, creating engaging and interactive user experiences has become essential. One of the best ways to achieve this is by incorporating animations and effects into your web applications. jQuery provides an extensive set of tools and libraries that make it easy to add these features to your website.
What is jQuery Animation?
jQuery animation is the process of changing the styles of an element over time to create a sense of motion and interactivity. This can include moving, fading, and resizing elements on the page. By adding these dynamic effects to your web application, you can make it more engaging and intuitive.
jQuery Effects
jQuery offers a wide range of effects that can be applied to elements on your web page. These effects include:
Effect | Description |
---|---|
fadeIn() | Fades in an element |
fadeOut() | Fades out an element |
slideUp() | Slides up an element |
slideDown() | Slides down an element |
animate() | Animates an element’s styles |
These are just a few examples of the many effects that jQuery offers. By using these effects, you can create a more engaging and interactive user experience on your website.
Form Validation and User Input with JavaScript and jQuery
Validating user input and ensuring data integrity is critical in developing effective web applications. With JavaScript and jQuery, you can easily perform client-side form validation to provide users with real-time feedback on their input.
The process of validating user input involves checking user-entered data against a set of predefined rules. These rules can include checking for the correct data type, minimum and maximum character limits, and required fields. With JavaScript and jQuery, you can create custom validation rules and messages to provide users with informative feedback on their input.
Validation Type | Description |
---|---|
Required Field | Ensures that a field is not left empty or blank |
Minimum Length | Checks that the entered data meets a minimum length requirement |
Maximum Length | Checks that the entered data does not exceed a maximum length limit |
Regular Expression | Uses a pattern to match input against a specific format |
JavaScript and jQuery provide a range of built-in validation methods and plugins to simplify the process of validating user input. These built-in tools can be combined with custom validation rules to create robust and effective validation systems.
By providing real-time validation feedback to users, you can improve the user experience and prevent errors before they occur. With JavaScript and jQuery, it’s easy to create dynamic and responsive forms that enhance the overall functionality of your web applications.
Responsive Web Design with JavaScript and jQuery
Responsive web design is essential for creating web applications that are flexible and optimized for a variety of devices. JavaScript and jQuery play an important role in achieving this goal by adapting layouts and improving user interactions.
One common technique for responsive web design is using CSS media queries to detect and adjust to different screen sizes. JavaScript and jQuery can enhance this approach by adding dynamic effects, such as image sliders, that respond to user interactions. Additionally, jQuery offers powerful animation capabilities that can be used to create subtle effects that improve the user experience.
Another way to ensure responsive web design is by optimizing user input and interactions. JavaScript and jQuery can improve the handling of user input by providing real-time feedback and validation. For example, jQuery’s validation plugin can be used to ensure that passwords are strong enough, or that email addresses are formatted correctly.
Finally, JavaScript and jQuery can help optimize the performance of web applications by reducing the amount of data sent over the network. AJAX (Asynchronous JavaScript and XML) is a technique that allows web pages to update content without requiring a page reload. This results in faster and more engaging interactions. Additionally, JavaScript’s ability to manipulate the Document Object Model (DOM) in real-time allows for efficient and targeted updates to specific areas of the page.
Accessibility and Performance Optimization with JavaScript and jQuery
Developers must ensure that their web applications are accessible to all users, including those with disabilities. JavaScript and jQuery can help in achieving the necessary accessibility compliance by providing rich user interfaces and enhanced functionality while maintaining accessibility standards.
Performance optimization is another essential aspect of web development. By reducing page load times, web applications can provide a better user experience and higher search engine rankings. JavaScript and jQuery can aid in optimizing the performance of web applications by reducing the size of web pages, combining external files, and minimizing network requests.
Developers should prioritize website accessibility and performance optimization during the development phase to ensure that users have a positive experience, regardless of their abilities or device. By utilizing JavaScript and jQuery, developers can create web applications that are both accessible and performant.
Integrating JavaScript and jQuery with Other Web Technologies
JavaScript and jQuery are versatile technologies that can be smoothly integrated with other web technologies to create powerful and dynamic web applications. One such technology is HTML5, the latest version of the HTML standard that offers several new features for web development. By utilizing HTML5’s capabilities, web developers can create advanced web applications that work seamlessly across multiple devices.
CSS3 is another web technology that can be paired with JavaScript and jQuery to enhance the visual appeal of web applications. CSS3 offers numerous new features such as animations and transitions that can be used in conjunction with jQuery to create visually stunning web applications.
Server-side languages like PHP, Ruby, and Python can be used to interact with databases and perform complex operations on the server. By integrating JavaScript and jQuery with these languages, developers can create web applications that provide fast and responsive user experiences.
Lastly, JavaScript and jQuery can be used in conjunction with content management systems (CMS) like WordPress, Drupal, and Joomla. By leveraging JavaScript and jQuery’s capabilities, developers can create custom modules and themes for CMS platforms that provide unique functionality and improved user experiences.
Conclusion
JavaScript and jQuery are essential tools for creating interactive and dynamic web applications. With JavaScript, developers can create animations, manipulate the DOM, handle events, and validate user input. jQuery simplifies the coding process by providing a powerful set of tools and libraries that can enhance the functionality and user experience of web applications.
By following the step-by-step guidance provided in this article, you can get started with JavaScript and jQuery and learn how to use them to create robust and dynamic web applications. JavaScript and jQuery can contribute to responsive web design, accessibility, and performance optimization, making them valuable tools for web developers in today’s fast-paced digital world.
Integrating JavaScript and jQuery with other web technologies such as HTML5, CSS3, and server-side languages can also help developers create more advanced and complex web applications. With its wide range of features and capabilities, JavaScript and jQuery continue to be essential tools for creating the modern web.
FAQ
Q: What is the purpose of this guide?
A: This guide aims to provide an introduction to interactive front-end web development using JavaScript and jQuery, covering various topics and techniques.
Q: Why is JavaScript important in web development?
A: JavaScript plays a crucial role in modern web development as it allows for creating interactivity and enhancing the overall user experience.
Q: What is jQuery and why is it relevant?
A: jQuery is a powerful JavaScript library that simplifies coding by providing a wide range of features and tools, making JavaScript development more efficient.
Q: How can I get started with JavaScript and jQuery?
A: This guide provides step-by-step guidance on setting up a development environment and understanding the basic syntax and concepts of JavaScript and jQuery.
Q: What is DOM manipulation and how can I use it?
A: DOM manipulation involves dynamically changing the structure and content of web pages using JavaScript and jQuery to create interactive and responsive experiences.
Q: How can I handle events and make AJAX requests?
A: This guide explores event handling techniques and teaches how to make asynchronous requests using AJAX with JavaScript and jQuery to create interactive web applications.
Q: Can jQuery be used to create animations and effects?
A: Yes, jQuery offers a wide range of animation and visual effects capabilities that can be used to add flair and interactivity to web applications.
Q: How can I validate user input in forms?
A: JavaScript and jQuery can be used to validate user input in forms, ensuring data integrity and providing a seamless user experience.
Q: How can JavaScript and jQuery contribute to responsive web design?
A: JavaScript and jQuery can adapt layouts and optimize user interactions for different devices, contributing to the creation of responsive web designs.
Q: What techniques can be used to improve accessibility and performance?
A: This guide explores techniques for improving the accessibility and performance of web applications using JavaScript and jQuery.
Q: How can I integrate JavaScript and jQuery with other web technologies?
A: This guide provides insights into integrating JavaScript and jQuery with other web technologies, such as HTML5, CSS3, and server-side languages, to create robust and dynamic web applications.
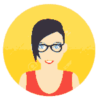
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.