Server Side Rendering with Chart.js: A Strategic Approach for Startups
In the fast-paced and innovation-driven environment of early-stage startups, efficiency and scalability are crucial. As a CTO, understanding the importance of presenting complex data in an accessible and visually appealing manner is key. This is where ‘Server Side Rendering with Chart.js’ comes into play.
This approach leverages Chart.js, a popular JavaScript library, for creating responsive, interactive charts and graphs, directly on the server side.
This technique not only enhances the performance and speed of your web applications but also ensures consistency in data visualization across different devices and platforms. By integrating server-side rendering with Chart.js, you’ll be able to present complex data sets in a more engaging and insightful way, providing a competitive edge in data-driven decision making.
What is Server Side Rendering?
Server Side Rendering (SSR) is a technique used in web development where the content of a webpage, including HTML, CSS, and JavaScript, is generated on the server rather than in the user’s browser. This approach delivers fully formed pages to the browser, making the content immediately visible and accessible to users and search engines.
For a CTO at an early startup, implementing SSR can lead to improved site performance, faster load times, and enhanced SEO, crucial for engaging users and scaling effectively in the competitive digital landscape.
Introducing Chart.js
Chart.js is a versatile and easy-to-use JavaScript library for creating interactive and visually appealing charts and graphs on web pages. It supports a wide range of chart types such as line, bar, pie, radar, and more. Here’s a basic introduction with a code sample to get you started:
First, ensure you include Chart.js in your project. You can do this by adding the following script tag to your HTML:
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
Next, add a canvas element to your HTML where the chart will be rendered:
<canvas id="myChart" width="400" height="400"></canvas>
Now, you can create a chart using JavaScript. Here’s an example of a simple line chart:
var ctx = document.getElementById('myChart').getContext('2d');
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [{
label: '# of Votes',
data: [12, 19, 3, 5, 2, 3, 7],
backgroundColor: 'rgba(255, 99, 132, 0.2)',
borderColor: 'rgba(255, 99, 132, 1)',
borderWidth: 1
}]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
});
In this example:
- We first get the context of the canvas (
ctx
) where the chart will be drawn. - Then, we create a new
Chart
object, specifying the type (line
in this case). - The
data
object containslabels
for the X-axis and adatasets
array for the chart data, styling, and other properties. - The
options
object is used to configure various aspects of the chart, like starting the Y-axis at zero.
Remember, Chart.js is highly customizable, so you can tweak a wide array of settings to suit your needs.
The Need for Server Side Rendering
Server Side Rendering (SSR) is a crucial technique in modern web development, particularly important for startups looking to enhance user experience and optimize for search engines. SSR involves rendering web pages on the server instead of the client-side.
This process results in faster page load times, improved SEO as search engine crawlers can easily index the content, and a more consistent user experience across different devices and network conditions.
Here’s a basic example of implementing SSR using Node.js and Express:
First, set up your Node.js environment and install Express:
npm init -y
npm install express
Create an index.js
file with the following code:
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/', (req, res) => {
const html = `
<!DOCTYPE html>
<html>
<head>
<title>My Server Side Rendered Page</title>
</head>
<body>
<h1>Hello from the server side!</h1>
</body>
</html>
`;
res.send(html);
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
In this simple example:
- We set up an Express server.
- When the root URL (
'/'
) is accessed, the server renders an HTML page. - The
html
constant contains the HTML string that will be sent back to the client. - The server listens on a specified port (3000 in this case).
This example demonstrates the essence of SSR: rendering HTML content on the server before sending it to the client. For startups, applying SSR can significantly improve initial load times and overall performance, crucial for retaining users and improving search rankings.
Implementing Server Side Rendering with Chart.js
Server side rendering with Chart.js is a powerful technique for generating data visualizations that load quickly and efficiently. To implement server side rendering with Chart.js, follow these steps:
Install Chart.js: Start by installing the Chart.js library on your server using npm or another package manager. For example:
$ npm install chart.js --save
Create a Chart: Next, create a new Chart object in your server-side code. You can use the same Chart.js API and configuration options that you would use on the client side. For example:
// import Chart.js
const Chart = require('chart.js');
// create new Chart object
const chart = new Chart(ctx, {
type: 'line',
data: {
labels: ['Jan', 'Feb', 'Mar', 'Apr', 'May'],
datasets: [{
label: 'Sales',
backgroundColor: 'rgba(255, 99, 132, 0.2)',
borderColor: 'rgba(255, 99, 132, 1)',
borderWidth: 1,
data: [12, 19, 3, 5, 2],
}]
});
Render the Chart: Finally, use the canvas renderer provided by Chart.js to render the chart as an image. For example:
// render the chart as a PNG image
const image = chart.canvas.toBuffer('image/png');
res.writeHead(200, {'Content-Type': 'image/png' });
res.end(image);
With these simple steps, you can generate Chart.js visualizations on the server side. This approach can help improve performance, SEO, and accessibility for your web applications.
Comparing Client Side Rendering and Server Side Rendering
In web development, choosing between Client Side Rendering (CSR) and Server Side Rendering (SSR) is crucial, each with distinct advantages and use cases. CSR, where the browser executes JavaScript to render pages, is ideal for dynamic, interactive web applications.
It offers a rich user experience and reduces server load, but can lead to slower initial load times. SSR, on the other hand, involves rendering pages on the server before sending them to the client. This approach provides faster initial load times and is SEO-friendly, but can increase server load.
Here’s a basic comparison with code samples for both CSR and SSR using a simple webpage as an example:
Client Side Rendering:
HTML File (index.html):
<!DOCTYPE html>
<html>
<head>
<title>CSR Example</title>
</head>
<body>
<div id="app"></div>
<script src="app.js"></script>
</body>
</html>
JavaScript File (app.js):
document.getElementById(‘app’).innerHTML = ‘<h1>Hello, this is rendered on the client side!</h1>’;
In CSR, the JavaScript (app.js) manipulates the DOM after the initial page load, adding content to the div
with id="app"
.
Server Side Rendering:
Node.js Server (server.js):
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/', (req, res) => {
res.send(`
<!DOCTYPE html>
<html>
<head>
<title>SSR Example</title>
</head>
<body>
<h1>Hello, this is rendered on the server side!</h1>
</body>
</html>
`);
});
app.listen(PORT, () => {
console.log(`Server running on http://localhost:${PORT}`);
});
In SSR, the content is rendered directly into the HTML on the server (within server.js
), and a complete HTML page is sent to the client.
In summary, CSR offers a dynamic, app-like experience at the cost of initial load time, while SSR provides faster initial loads and SEO benefits, which can be critical for content-heavy sites and initial user impressions.
Best Practices for Server Side Rendering with Chart.js
Server side rendering with Chart.js provides many benefits in terms of performance, SEO, and user experience. However, to ensure smooth rendering of charts on the server side, it is important to follow some best practices and optimization techniques. Here are some tips to help you get started:
- Preprocess Data: Before rendering the chart on the server side, it is important to preprocess the data to ensure that it is in the correct format and that it is optimized for rendering. This includes filtering and formatting data as well as performing any necessary data calculations.
- Cache Results: Caching is a powerful tool that can significantly improve performance by reducing the load on the server. You can use caching to store the result of the chart rendering process so that it can be quickly retrieved and displayed to the user when needed.
- Optimize Performance: There are several ways to optimize performance when rendering charts on the server side. For example, you can use a lightweight server-side language like NodeJS, or you can use a load balancer to distribute the load across multiple servers.
Example 1: Preprocessing Data
Here is an example of how to preprocess data before rendering the chart:
// Sample Data var data = [ { label: 'January', value: 10 }, { label: 'February', value: 20 }, { label: 'March', value: 30 }, { label: 'April', value: 40 }, { label: 'May', value: 50 } ]; // Preprocess Data var chartData = []; for (var i = 0; i < data.length; i++) { chartData.push({ label: data[i].label, data: data[i].value }); }
Example 2: Caching Results
Here is an example of how to cache the result of chart rendering:
// Set Cache function setCache(key, data) { // Store data in cache } // Get Cache function getCache(key) { // Retrieve data from cache } // Render Chart with Cache function renderChartWithCache(chartData) { var cacheKey = JSON.stringify(chartData); var cachedResult = getCache(cacheKey); if (cachedResult) { // Use cached result } else { // Render chart and store result in cache var result = renderChart(chartData); setCache(cacheKey, result); } }
Example 3: Optimizing Performance
Here is an example of how to optimize performance when rendering charts on the server side:
// Use Load Balancer var loadBalancer = require('load-balancer'); loadBalancer.start({ servers: [ { host: 'server1', port: 8080 }, { host: 'server2', port: 8080 }, { host: 'server3', port: 8080 } ] }); // Use Lightweight Server-Side Language var http = require('http'); http.createServer(function (req, res) { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('Hello World\n'); }).listen(8080);
By following these best practices and optimization techniques, you can ensure that your server side rendering with Chart.js is fast, efficient, and effective.
Conclusion: Embrace the Power of Server Side Rendering with Chart.js
Server side rendering with Chart.js is a game changer for web data visualizations. By generating charts on the server side, you can improve performance, ensure smooth rendering across devices, optimize for SEO, and enable faster loading times for end users.
With Chart.js, the process of implementing server side rendering is easy and straightforward. Simply configure your server-side environment with Node.js, install the necessary dependencies, and start generating charts with your preferred data source. This flexibility and ease of use has made Chart.js a popular choice among developers for creating interactive and responsive data visualizations.
External Resources
https://www.chartjs.org/docs/latest/
https://reactjs.org/docs/react-dom-server.html
FAQ
Here are five frequently asked questions about Server Side Rendering (SSR) with Chart.js, tailored for a CTO at an early startup in the USA, along with code samples for each:
1. How do I set up a basic server-side rendered chart using Chart.js in Node.js?
Answer: To set up a basic SSR chart, you’ll need to use a Node.js server environment. Here’s a simple example using Express and Chart.js:
// Node.js server setup
const express = require('express');
const { ChartJSNodeCanvas } = require('chartjs-node-canvas');
const app = express();
const port = 3000;
const width = 400; // chart width
const height = 400; // chart height
const chartJSNodeCanvas = new ChartJSNodeCanvas({ width, height });
app.get('/chart', async (req, res) => {
const configuration = {
type: 'bar', // chart type
data: {
labels: ['Red', 'Blue', 'Green'],
datasets: [{
label: 'Colors',
data: [12, 19, 3],
backgroundColor: ['red', 'blue', 'green']
}]
}
};
const image = await chartJSNodeCanvas.renderToBuffer(configuration);
res.type('image/png');
res.send(image);
});
app.listen(port, () => console.log(`Server running at http://localhost:${port}`));
2. How can I customize the appearance of charts in SSR?
Answer: Chart.js offers extensive customization options. You can modify the chart’s appearance by adjusting the configuration
object in the server-side script. This includes changing chart types, colors, labels, and adding interactions.
const configuration = {
type: 'line',
data: { /* data settings */ },
options: {
scales: { /* scale settings */ },
title: {
display: true,
text: 'Custom Chart Title'
}
// Additional customization here
}
};
3. Can SSR with Chart.js improve the performance of my web application?
Answer: Yes, using SSR with Chart.js can enhance performance. Since the charts are rendered on the server, it reduces the initial load time and processing on the client-side, making the application faster and more efficient.
4. How can I ensure my SSR charts are responsive on different devices?
Answer: Responsiveness in SSR is managed by setting the chart dimensions dynamically based on the request parameters or using CSS for the image tag in the HTML.
app.get('/chart', async (req, res) => {
const width = req.query.width || 400; // Dynamic width
const height = req.query.height || 400; // Dynamic height
// Rest of the chart rendering code
});
5. Is it possible to cache the rendered charts on the server for faster delivery?
Answer: You can implement caching mechanisms on your server to store previously rendered charts and serve them quickly for repeated requests.
const cache = {};
app.get('/chart', async (req, res) => {
const chartKey = 'some_unique_key'; // Define a unique key for the chart
if (cache[chartKey]) {
res.type('image/png');
return res.send(cache[chartKey]);
}
// Chart rendering logic
const image = await chartJSNodeCanvas.renderToBuffer(configuration);
cache[chartKey] = image; // Cache the rendered image
res.type('image/png');
res.send(image);
});
These FAQs and code samples provide a foundational understanding of how SSR with Chart.js can be implemented and leveraged in a startup environment, emphasizing performance optimization and user experience enhancement.
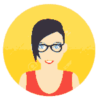
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.