Things I wish I knew about: How to refresh page with JavaScript: Use location.reload()
for a full refresh or history.go(0)
for a soft reload. For timed refreshes, utilize setTimeout()
or setInterval()
.
To refresh from another page, employ the Window.postMessage()
method. Remember, wisely consider user experience when implementing refreshes.
Step 1: Understanding the Basics
Everything starts somewhere. For us, that journey commences in understanding JavaScript. This programming language forms a core part of web development, often used to create interactive effects within browsers. Think about a time when you clicked a button, and a form popped up—that’s JavaScript in action.
Step 2: Identifying the Need to Refresh
Secondly, we must identify why we want to refresh a page. Let’s paint a picture here. Imagine you’re browsing an online store. You add items to your cart, but the cart doesn’t update.
Annoying, right? That’s where JavaScript comes in handy, refreshing parts of the page without requiring a complete reload. A key tool in any developer’s toolkit.
Step 3: Diving into the Code
Then we delve into the actual code. Not too deep, though—there’s no need to worry. JavaScript has a built-in method called location.reload()
, which allows us to refresh a web page. That’s it. Really! But why stop there?
Let’s explore some of the cool options we have.
Step 4: Playing with Options
Did you know you can force a hard refresh with JavaScript? It’s like hitting the F5 key on your keyboard. You can achieve this with location.reload(true)
. What’s happening behind the scenes, you ask? Well, this function forces the browser to bypass the cache and download the entire web page again.
It’s like giving your page a fresh start.
Step 5: Implementing the Code
Now, time to put our knowledge into practice. You can trigger the location.reload()
function in multiple ways—perhaps when a button gets clicked or after a certain time period. So, how would you use it? A common scenario would be to refresh a page after a user action.
Let’s say, updating a shopping cart. So, you could use a code snippet like this:
document.getElementById("update-cart").addEventListener("click", function() {
location. Reload();
});
In this example, we’ve attached a click event to a button with the id “update-cart.” Once that button gets clicked, voila! The page refreshes.
Step 6: Keeping the User in Mind
Remember, with great power comes great responsibility. Yes, I just quoted Spiderman. But it’s true. Refreshing a page might be a useful tool, but it can also disrupt the user’s experience if used excessively. Let’s not forget who we’re coding for—the user. Striking a balance is essential.
Step 7: Keeping Up with Change
Every day, web technology evolves. New methods come into play; old methods retire. The world of JavaScript is no different. As you continue your development journey, remember to stay updated with changes and adapt accordingly. Remember our friend location.reload()
?
While immensely helpful, it might not be the only way to refresh a page in the future. So, keep exploring, keep learning.
That’s the beauty of this field.
Step 8: Exploring Alternatives
Speaking of change, you might wonder if there are alternatives to location.reload()
. And rightly so! Here’s where AJAX (Asynchronous JavaScript and XML) enters the scene. This technique allows you to update parts of a webpage without reloading the entire page.
It’s like having your cake and eating it too. But that’s a topic for another day.
Step 7: Wrapping Up
Finally, time to reflect. We started with understanding the basics of JavaScript, moved on to identifying the need to refresh a page, then dove into the code, played around with options, implemented it, and considered the user’s perspective. Look how far you’ve come!
Learning how to refresh a page with JavaScript isn’t just about writing a few lines of code. It’s about understanding the why, the how, and the when. With this knowledge in hand, you’re ready to create web pages that are more dynamic and user-friendly. Remember, each line of code you write isn’t just a command—it’s a step towards building a better web experience. Go forth, and code!
A word of caution, though. Refreshing a page can be helpful but can also disrupt the user’s browsing experience if overused. As developers, we need to ensure that we use tools like location.reload()
responsibly, with the user’s experience in mind.
We’ve also kept ourselves open to learning about new techniques and considered potential alternatives.
By applying the knowledge in this article, you can create a better, more dynamic, and ultimately more satisfying web experience. In every line of code you write, keep your users at the heart of your decisions.
Mastering how to refresh a page with JavaScript is not just about understanding a command. It’s about knowing when and where to use it, how it impacts the user, and how it fits into the ever-evolving landscape of web development.
As a developer, the world of web technology is your playground. Every new command you learn, every technique you master, is a new toy to play with.
Developer Frequently Asked Questions: How to refresh page with JavaScript using JavaScript
What is the primary function used in JavaScript to refresh a page?
The most common function for page refresh in JavaScript is location.reload()
.
How does location.reload()
function work?
This built-in JavaScript method refreshes the current document, similar to clicking the refresh button in your browser.
What’s the difference between location.reload()
and location.reload(true)
?
Using location.reload(true)
forces a hard refresh, bypassing the browser cache and re-fetching the entire webpage from the server.
Can we refresh a page after a certain event with JavaScript?
Absolutely! By attaching an event listener, you can refresh a page after a specific event, such as a button click or form submission.
Is it possible to refresh part of a page with JavaScript?
Yes, by using AJAX (Asynchronous JavaScript and XML), you can refresh or update parts of a webpage without reloading the entire page.
What should be considered before refreshing a page using JavaScript?
Think about the user experience. Page refresh can be disruptive if overused. Always keep the balance between functionality and user comfort.
Is JavaScript the only way to refresh a page?
No, not at all. Various server-side languages like PHP or frameworks like ASP.NET can trigger page refreshes. But JavaScript provides an easy, client-side method for this task.
Will location.reload()
always be the way to refresh a page in JavaScript?
Technology evolves constantly, and JavaScript is no different. While location.reload()
is currently a reliable way to refresh a page, other methods may arise in the future. Stay updated, stay flexible.
With these FAQs, you should have a comprehensive understanding of how to refresh a page with JavaScript. Keep exploring, keep coding, and never stop learning.
Developer FAQs: How to refresh page with JavaScript from another page
How to refresh page with JavaScript from another page: You can utilize Window.postMessage()
method to communicate between pages and trigger a refresh.
Set up a message listener on the page to be refreshed and send a message from another page when a refresh is needed.
1. Can JavaScript refresh a page from a different one?
JavaScript primarily operates within the context of a single webpage. Directly refreshing a different webpage using just JavaScript isn’t typically feasible.
2. How can indirect page refreshes be accomplished using JavaScript?
Indirect refreshes can be achieved using a WebSocket or Server-Sent Events. You can send a message from one webpage to a server, which then communicates with the other webpage, telling it to refresh.
Code Sample using WebSocket:
// Page A: Emit an event
socket.emit('refreshPage');
// Server: Listen for the event and broadcast it to other clients
socket.on('refreshPage', () => {
socket.broadcast.emit('refreshPage');
});
// Page B: Listen for the event and refresh when it's received
socket.on('refreshPage', () => {
location. Reload();
});
3. Can localStorage or sessionStorage be used to trigger a page refresh?
Yes, sessionStorage and localStorage can store flags which can be checked to determine if a page should refresh. These can be accessed across different pages within the same domain.
Code Sample using localStorage:
// Page A: Set flag
localStorage.setItem('shouldRefresh', true);
// Page B: Check flag and refresh if needed
window.onload = function() {
if(localStorage.getItem('shouldRefresh')) {
localStorage.removeItem('shouldRefresh');
location. Reload();
}
};
4. Is it possible to refresh an iframe from the parent page?
Yes, if an iframe is present on your page, JavaScript can refresh this iframe, effectively refreshing another page from the parent page.
Code Sample:
// Parent Page: Refresh iframe
document.getElementById('myIframe').contentWindow.location.reload();
5. Can JavaScript on one device refresh a page on another device?
Not directly. But with backend server technologies combined with JavaScript, you can set up real-time communication (like using WebSockets) to trigger a refresh on another device.
6. Can AJAX be used to refresh content on another page?
AJAX can refresh content on the current page without a full page reload. However, it doesn’t directly refresh a different page. For this, indirect methods, such as server communication, would need to be employed.
7. What is the best practice when trying to refresh a page from another page?
User experience is crucial to consider. While technically possible through certain workarounds, such behaviour can potentially confuse users. Make sure it’s necessary for your application, and implement it responsibly.
8. Are there alternatives to JavaScript for refreshing a page from another page?
Server-side languages and frameworks often have methods for these actions, such as PHP sessions or .NET SignalR for real-time page updates.
In development, the direct path isn’t always possible. However, with creative problem-solving and a thorough understanding of your tools, like JavaScript, you can achieve your goals.
Developer FAQs: How to refresh page with JavaScript examples
1. How can I refresh a webpage using JavaScript?
The location.reload()
method is a simple way to refresh a webpage.
Code Sample:
// Refresh the current webpage
location. Reload();
2. How can I force a hard refresh with JavaScript?
To bypass the cache and force a hard refresh, you can use location.reload(true)
.
Code Sample:
// Force a hard refresh
location. Reload(true);
3. Can I refresh a webpage after a delay using JavaScript?
Yes, using setTimeout()
, you can delay the execution of location. Reload()
.
Code Sample:
// Refresh the page after 5 seconds
setTimeout(() => {
location.reload();
}, 5000);
4. How can I refresh a webpage in response to a user event?
By using an event listener, you can trigger a page refresh based on a user action, such as clicking a button.
Code Sample:
// Refresh the page when a button is clicked
document.getElementById('myButton').addEventListener('click', () => {
location.reload();
});
5. Can I cancel a page refresh?
Yes, you can cancel a delayed page refresh by clearing the timeout.
Code Sample:
// Set a page refresh to occur after 5 seconds
let refreshTimeout = setTimeout(() => {
location.reload();
}, 5000);
// Cancel the page refresh
clearTimeout(refreshTimeout);
Remember, page refreshes should be used judiciously to avoid disrupting the user’s experience. As a developer, always consider the implications of each action and prioritize user experience in your designs.
Developer FAQs about: JavaScript refresh page on button click
1. Can a button click in an iframe trigger a refresh of the parent page?
Yes, a button inside an iframe can trigger a refresh of the parent page. You would need to use parent.location.reload()
from within the iframe.
Code Sample:
// Inside the iframe, add an event listener to a button
document.getElementById('iframeButton').addEventListener('click', () => {
parent.location.reload();
});
2. Can I confirm with the user before refreshing the page on button click?
Certainly! You can use JavaScript’s confirm()
function to get a user’s confirmation before refreshing the page.
Code Sample:
// Ask for user confirmation before refreshing the page
document.getElementById('myButton').addEventListener('click', () => {
let confirmed = confirm("Are you sure you want to refresh the page?");
if (confirmed) {
location. Reload();
}
});
3. Can I use jQuery to refresh the page on button click?
Yes, jQuery also supports event listeners and can be used to refresh a page when a button is clicked.
Code Sample:
// Using jQuery to refresh the page on button click
$('#myButton').click(function() {
location. Reload();
});
4. Can a button click trigger a refresh of a different part of the page instead of a full page refresh?
Indeed! A button click can be used to trigger AJAX calls that refresh part of a webpage without a full page reload.
Code Sample:
// Refresh part of the page using AJAX on button click
document.getElementById('myButton').addEventListener('click', () => {
fetch('/api/data')
.then(response => response.text())
.then(data => {
document.getElementById('partToRefresh').innerHTML = data;
});
});
With JavaScript, the opportunities for interactivity and user engagement are vast. Always remember to consider the user experience when deciding when and how to refresh your webpage.
Developer FAQs: How do you refresh a web page in JavaScript?
1. Can I refresh a webpage at regular intervals using JavaScript?
Yes, using setInterval()
, you can refresh a webpage at regular intervals.
Code Sample:
// Refresh the page every 60 seconds
setInterval(() => {
location. Reload();
}, 60000);
2. How can I refresh the webpage to a specific part or section?
You can use location.hash
to refresh and navigate to a specific part of your webpage.
Code Sample:
// Refresh and navigate to a specific part of the webpage
location.reload();
location.hash = '#sectionID';
3. Is there a way to refresh the webpage without scrolling back to the top?
Yes, you can combine scrollX
and scrollY
with scrollTo()
to maintain the current scroll position after refreshing.
Code Sample:
// Get current scroll position before refreshing
let scrollX = window.scrollX;
let scrollY = window.scrollY;
// Refresh the page
location.reload();
// Scroll back to the original position after refreshing
window.scrollTo(scroll, scrollY);
4. Can I use JavaScript to refresh another webpage in a different tab or window?
Directly, no. But you can use a combination of JavaScript with some backend server technology and cookies or localStorage to send a signal that can cause a refresh in another tab.
5. How to use a keyboard shortcut to refresh a webpage using JavaScript?
You can create a keyboard shortcut for refreshing the webpage using addEventListener()
for keydown
event.
Code Sample:
// Refresh the page when 'r' key is pressed
window.addEventListener('keydown', (event) => {
if (event.key === 'r') {
location. Reload();
}
});
With JavaScript, the capabilities are wide-ranging. It’s not just about writing code, it’s about creating an optimal, user-friendly experience. As you further your knowledge, remember to implement these techniques responsibly and keep exploring!
Developer FAQs on: How to refresh page in Chrome JavaScript?
1. Are there specific considerations for refreshing a webpage in Chrome using JavaScript?
While JavaScript is generally browser-agnostic, certain browser-specific issues may arise. Nonetheless, the location.reload()
method typically works across all browsers, including Chrome.
Code Sample:
// Refresh the current webpage location. Reload();
2. Is there a way to handle potential cache issues in Chrome when refreshing the page?
To avoid cache issues, you may opt to perform a hard refresh using location.reload(true)
in Chrome.
Code Sample:
// Force a hard refresh, bypassing the cache location. Reload(true);
3. Can I use JavaScript to automate the process of clearing cache before refreshing a page in Chrome?
Unfortunately, JavaScript does not have the capability to clear a browser’s cache. This action is typically handled by the browser itself. However, using location.reload(true)
, can force a hard refresh, which bypasses the cache.
4. How can I trigger Chrome’s “Pull-to-refresh” feature using JavaScript?
Chrome’s “Pull-to-refresh” feature is a user-initiated action and cannot be programmatically triggered by JavaScript. However, a refresh can be simulated using location.reload()
.
5. Can I disable the Chrome alert “Confirm Form Resubmission” on page refresh with JavaScript?
The “Confirm Form Resubmission” alert in Chrome cannot be directly disabled via JavaScript. This alert is a built-in browser feature that appears when a form submission is about to be repeated due to a page refresh.
To avoid this alert, you can consider using AJAX for form submissions or the PRG (Post/Redirect/Get) pattern.
While there are some browser-specific considerations, most JavaScript operations, including page refresh, function consistently across all modern browsers.
It’s crucial, though, to keep up-to-date with browser changes as they may occasionally impact JavaScript functionality.
Developer FAQs: How to auto refresh page every 30 seconds in JavaScript?
1. How can I auto-refresh a webpage every 30 seconds using JavaScript?
You can use the setInterval()
method in JavaScript to refresh a webpage every 30 seconds.
Code Sample:
// Refresh the page every 30 seconds setInterval(() => { location.reload(); }, 30000);
2. Is there a way to stop the auto-refresh after a certain period?
Yes, you can use clearInterval()
to stop the auto-refresh after a certain period.
Code Sample:
// Start refreshing the page every 30 seconds
let refreshIntervalId = setInterval(() => {
location.reload();
}, 30000);
// After 5 minutes, stop the auto-refresh
setTimeout(() => {
clearInterval(refreshIntervalId);
}, 300000);
3. Can I auto-refresh a page every 30 seconds but only during certain hours?
Yes, you can include a check for the current hour before executing the refresh.
Code Sample:
// Refresh the page every 30 seconds, but only between 9am and 5pm setInterval(() => { let currentHour = new Date().getHours(); if (currentHour >= 9 && currentHour <= 17) { location.reload(); } }, 30000);
4. Is it possible to auto-refresh a page every 30 seconds, but pause if the user is actively interacting with the page?
Yes, you can detect user activity and pause the auto-refresh accordingly.
Code Sample:
let lastActivity = new Date().getTime(); // Detect user activity window.onmousemove = window.onkeypress = () => { lastActivity = new Date().getTime(); }; // Refresh the page every 30 seconds, but pause if user is active setInterval(() => { if (new Date().getTime() - lastActivity > 30000) { location.reload(); } }, 30000);
Although auto-refreshing a page every 30 seconds can be useful in certain scenarios, it’s important to consider the user experience. Frequent refreshes could potentially disrupt user activity, so use this when necessary.
Developer FAQs: How to refresh a page in JavaScript without reloading
1. Can I refresh part of a webpage without reloading the entire page in JavaScript?
Indeed, by utilizing AJAX, you can refresh a portion of your webpage without a complete page reload.
Code Sample:
// Refresh a part of the webpage using AJAX fetch('/api/data') .then(response => response.text()) .then(data => { document.getElementById('partToRefresh').innerHTML = data; });
/* Your code... */
2. Can I dynamically update the DOM without a page refresh?
Yes, the Document Object Model (DOM) allows you to interactively change webpage content without needing a refresh.
Code Sample:
// Update DOM element content without refreshing
document.getElementById('elementToChange').textContent = 'New Content';
3. How can I use Vue.js or React.js to refresh a page without reloading?
With modern JavaScript frameworks like Vue.js or React.js, components are automatically updated when their state changes, without a full page refresh.
For instance, in Vue.js:
// Vue.js: Refresh a component by changing its data new Vue({ el: '#app', data: { message: 'Hello Vue.js!' }, methods: { updateMessage: function () { this.message = 'Updated message'; } } });
In React.js:
// React.js: Refresh a component by changing its state class App extends React.Component { constructor(props) { super(props); this.state = { message: 'Hello React!' }; } render() { return ( <div> {this.state.message} <button onClick={() => this.setState({ message: 'Updated message' })}>Update</button> </div> ); } } ReactDOM.render(<App />, document.getElementById('root'));
Refreshing a page without reloading is a common practice in modern web development, enhancing user experience with fluid and seamless interactions. As you work on your projects, remember that every detail, down to how you refresh content, contributes to the overall user experience.
Developer FAQs: How to reload page in JavaScript once
1. How can I ensure a webpage reloads only once using JavaScript?
You can use localStorage
to ensure a webpage reloads only once.
Code Sample:
// Reload the page once if (!localStorage.getItem('hasReloaded')) { location.reload(); localStorage.setItem('hasReloaded', 'true'); }
2. How can I reset the condition so the page can reload once again?
You can reset the condition by removing the item from localStorage
.
Code Sample:
// Reset the reload condition localStorage.removeItem('hasReloaded');
3. Can I reload the page once only after a specific event?
Yes, you can tie the reload to a specific event, like a button click.
Code Sample:
// Reload the page once after a button click document.getElementById('reloadButton').addEventListener('click', () => { if (!localStorage.getItem('hasReloaded')) { location.reload(); localStorage.setItem('hasReloaded', 'true'); } });
4. Is there a way to reload the page only once for a specific user?
By setting a unique key in localStorage
for each user, you can ensure the page reloads once per user.
Code Sample:
// Reload the page once per user if (!localStorage.getItem('hasReloaded' + userID)) { location.reload(); localStorage.setItem('hasReloaded' + userID, 'true'); }
The ability to control page reloads offers developers greater flexibility in enhancing user experience. Remember to use this tool judiciously, ensuring it contributes positively to your application’s functionality and user satisfaction.
Developer FAQs: How to reload page with URL in JavaScript
1. How can I reload a webpage to a specific URL using JavaScript?
You can use location.href
to reload a webpage to a specific URL.
Code Sample:
// Reload the webpage to a specific URL location.href = 'https://www.example.com';
2. Can I pass parameters to the URL when reloading the page?
Yes, you can include parameters in the URL when reloading the page.
Code Sample:
// Reload the webpage to a specific URL with parameters location.href = 'https://www.example.com?param1=value1¶m2=value2';
3. Can I use JavaScript to reload to a relative URL?
Yes, location.href
can handle both absolute and relative URLs.
Code Sample:
// Reload the webpage to a relative URL location.href = '/path/to/page';
/* Your code... */
4. Is it possible to change the URL without reloading the page?
Yes, you can change the URL without reloading the page using history.pushState()
.
Code Sample:
// Change the URL without reloading the page history.pushState({}, '', '/new-url');
In modern web development, being able to manipulate the URL programmatically offers great flexibility. Whether you’re implementing navigation, tracking page states, or handling URL parameters, JavaScript provides robust tools to achieve your goals. Remember, each tool used correctly enhances the overall user experience.
Developer FAQs: How to auto reload page in JavaScript
1. How can I make a webpage auto-reload using JavaScript?
Auto-reloading a webpage in JavaScript is straightforward with the setInterval()
function.
Code Sample:
// Auto-reload the page every minute (60000 milliseconds) setInterval(() => { location.reload(); }, 60000);
2. Can I auto-reload a webpage at specific times using JavaScript?
Yes, you can set specific times for auto-reload by using the Date
object together with setInterval()
.
Code Sample:
// Auto-reload the page every hour, on the hour setInterval(() => { let now = new Date(); if (now.getMinutes() === 0 && now.getSeconds() === 0) { location.reload(); } }, 1000);
3. Can I stop the webpage from auto-reloading after a certain period?
Yes, the clearInterval()
function can be used to stop auto-reloading after a certain period.
Code Sample:
// Start auto-reloading the page every minute let autoReload = setInterval(() => { location.reload(); }, 60000); // Stop auto-reloading after 30 minutes setTimeout(() => { clearInterval(autoReload); }, 1800000);
4. Can I make a webpage auto-reload based on user activity?
Yes, you can detect user activity and set the webpage to auto-reload when there is no activity.
Code Sample:
let lastActivity = new Date().getTime(); window.onmousemove = window.onkeypress = () => { lastActivity = new Date().getTime(); }; // Auto-reload the page if no user activity for 2 minutes setInterval(() => { if (new Date().getTime() - lastActivity > 120000) { location.reload(); } }, 1000);
Auto-reloading a webpage can be useful, but it’s crucial to ensure that it enhances the user experience rather than detracting from it. Carefully consider the impact of auto-reloads on the user interface and functionality.
Developer FAQs: How to refresh page in node JS
1. How can I trigger a page refresh from the server-side using Node.js?
While Node.js primarily handles server-side operations, it can notify a client-side script (e.g., via WebSockets) to reload the page.
Code Sample:
// Server-side: Node.js with socket.io io.on('connection', (socket) => { socket.emit('reload'); }); // Client-side: JavaScript with socket.io let socket = io(); socket.on('reload', () => { location. Reload(); });
2. Can I use Node.js to set automatic server-side redirection?
Yes, you can use res.redirect()
in Express, a Node.js framework, to redirect to a different page, which effectively refreshes the page.
Code Sample:
// Node.js with Express app.get('/refresh', (req, res) => { res.redirect('/newPage'); });
3. Can Node.js monitor file changes and refresh the page?
Yes, you can use tools like nodemon
or browser-sync
during development to refresh the page whenever a file changes.
Code Sample:
// Start server with nodemon // Install nodemon globally: npm install -g nodemon // Run your script with nodemon instead of node: nodemon server.js
4. Can I implement server-side polling with Node.js to refresh data?
Yes, server-side polling can be implemented with Node.js. When data changes, you can notify client-side JavaScript to update without a full page refresh.
Code Sample:
// Server-side: Node.js with Express app.get('/data', (req, res) => { res.json({ data: 'New data' }); }); // Client-side: JavaScript using Fetch API for polling setInterval(() => { fetch('/data') .then(response => response.json()) .then(data => { document.getElementById('data').textContent = data.data; }); }, 60000);
In web development, it’s crucial to separate concerns: Node.js manages server-side operations, while client-side JavaScript manipulates the webpage. Working together, these tools can deliver a dynamic, interactive user experience.
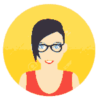
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.