To many, the Exclusive OR (XOR) operator in JavaScript remains an enigmatic and underused feature.
The hurdle? Its unique logic and lack of explicit representation in the language’s syntax can seem daunting.
But after years of honing my JavaScript skills, I’ve found XOR to be an invaluable tool for managing complex conditions, and I’m here to demystify its usage for you.
What is Exclusive OR?
In JavaScript, the exclusive OR (XOR) is a logical operator that compares two operands and returns true if only one of them is true. This is different from the OR operator, which returns true if one or both operands are true. The XOR operator is useful in scenarios where only one condition should be true, not both.
The XOR operator is represented by the symbol “^” in JavaScript, and is typically used to compare two Boolean expressions. For example:
let x = true;
let y = false;
console.log(x ^ y); // Output: true
In this example, the XOR operator returns true because only one of the operands (x and y) is true, not both.
The XOR operator is also commonly used in bit manipulation, where it can be used to toggle specific bits in a binary number. For example:
let a = 5; // binary 0101
let b = 3; // binary 0011
let c = a ^ b; // binary 0110 (decimal 6)
Here, the XOR operator is used to toggle the 2nd bit (from the right) of the binary number “a”, resulting in the binary number “c”.
Syntax of XOR in JavaScript
Now that we understand what exclusive OR (XOR) is, let’s delve into the syntax of XOR in JavaScript. The XOR operator in JavaScript is represented by ^
, which is also known as the caret or circumflex accent. It takes two operands and returns true only when one of the operands is true.
To use the XOR operator in code, simply place it between two operands. Below is an example:
let x = 5;
let y = 8;
let result = x ^ y;
console.log(result); // Output: 13
In the example above, XOR is used on two variables, x and y, which have values of 5 and 8 respectively. The result of using XOR between x and y is stored in a new variable called result. The value of result is then displayed on the console, which in this case is 13.
It’s important to note that the XOR operator has a lower precedence than the logical OR (||
) and logical AND (&&
) operators. This means that if they are used together in an expression, XOR will be evaluated last.
Additionally, it’s common to use XOR in combination with other operators to achieve a desired outcome. For instance, you can use XOR to toggle a boolean value between true and false. Here’s an example:
let value = false;
value = value ^ true;
console.log(value); // Output: true
In the above example, the boolean value is initially set to false. XOR is used between the value and true, effectively toggling the value to true. The new value of value is then displayed on the console.
Understanding the syntax of XOR in JavaScript is crucial for using it effectively in code. In the next section, we’ll explore various use cases for XOR in different industries and scenarios.
Use Cases for XOR in JavaScript
Exclusive OR (XOR) is a powerful logical operator in JavaScript that can be utilized in a variety of scenarios to enhance code efficiency and solve specific programming challenges. Let’s take a look at some use cases where XOR can be useful:
Conditional Statements
XOR can be used effectively in conditional statements where you want to execute a block of code if only one of the conditions is true. For example:
if ((a === true) ^ (b === true)) { // run this code if only one of the conditions
is true }
This code will only execute if either a or b is true, but not both.
Data Validation
XOR can also be used in data validation to ensure that only one of two conditions is met. For example, if you have a form with two checkboxes, but you only want the user to select one:
if ((checkbox1.checked) ^ (checkbox2.checked)) { // allow submission of form }
else { // display error message }
Here, the code checks if only one of the checkboxes is checked. If both are checked or neither are checked, an error message is displayed.
Encryption
XOR can also be used in encryption algorithms to add an extra layer of security. For example:
function encrypt(message, key) { let encryptedMessage = "";
for (let i = 0; i
In this code, the XOR operator is used to scramble the message with a key, making it more difficult for an unauthorized user to access the information.
These are just a few examples of how XOR can be utilized in JavaScript. The possibilities are endless and can vary from industry to industry and trend to trend.
XOR vs. Other Logical Operators
While XOR is a powerful operator, it’s not always the best solution for every problem. Let’s take a look at how XOR compares to other common logical operators in JavaScript.
AND Operator
The AND operator (&&) returns true if both operands are true. For example:
let a = true;
let b = false;
console.log(a && a); // true
console.log(a && b); // false
console.log(b && b); // false
As you can see, the AND operator requires both operands to be true in order to return true.
OR Operator
The OR operator (||) returns true if at least one operand is true. For example:
let a = true;
let b = false;
console.log(a || a); // true
console.log(a || b); // true
console.log(b || b); // false
The OR operator returns true as long as one operand is true, regardless of whether the other operand is true or false.
NOT Operator
The NOT operator (!) returns the opposite of the operand. For example:
let a = true;
console.log(!a); // false
let b = false;
console.log(!b); // true
The NOT operator simply flips the truth value of the operand.
While the AND, OR, and NOT operators all have their own uses, XOR is particularly useful in situations where you need to check for exclusivity. For example, if you need to check whether a user has selected either a checkbox or a radio button, XOR can simplify your code:
let checkbox = document.getElementById('checkbox');
let radio = document.getElementById('radio');
console.log(checkbox.checked ^ radio.checked);
The XOR operator returns true if only one of the operands (checkbox.checked or radio.checked) is true, making it perfect for this type of scenario.
XOR in JavaScript Frameworks
JavaScript frameworks have become essential tools for developers who seek to enhance and expedite their coding processes. XOR is a powerful operator that can be utilized in many ways within these frameworks.
AngularJS is a popular JavaScript framework that enables developers to build dynamic web applications. In AngularJS, XOR can be used to toggle the visibility of an element in the HTML document, depending on the state of a checkbox or radio button. Below is an example code:
<input type="checkbox" ng-model="showAuth" />
<div ng-show="showAuth ^ authenticated">You are not authenticated</div>
Here, the XOR operator is used in combination with the ng-show directive to display a message when the checkbox is checked but the user is not authenticated.
React is another popular JavaScript framework that emphasizes component-based development. XOR can be used in React to create conditional rendering of elements. Below is an example code:
<div>
<p>Choose a number between 1 and 5</p>
<input type="number" onChange="(e) => setSelectedNumber(parseInt(e.target.value))" />
<div className="result" >
<span>{(selectedNumber % 2) ^ 0 ? 'Odd' : 'Even'}</span>
</div>
</div>
In this code example, XOR is used with the modulus operator (%) to check if the number entered is odd or even. The XOR operation returns 1 if the result of the modulus operation is odd and 0 if it is even.
Frameworks like AngularJS and React demonstrate how XOR can be used effectively to enhance code functionality and improve user experience. By utilizing XOR in different ways within these frameworks, developers can create more efficient and elegant solutions to complex programming challenges.
Benefits of Using XOR in JavaScript
The exclusive OR (XOR) operator is a powerful tool in JavaScript coding, offering several benefits that can simplify complex logic, improve performance, and make code more readable and maintainable.
One of the primary benefits of using XOR in JavaScript is its ability to simplify code logic. XOR returns true when only one of the operands is true, making it ideal for scenarios where you need to check for one condition out of several.
Another advantage of using XOR is that it can improve performance. Using XOR can reduce code complexity and make your code more efficient, especially when dealing with complex Boolean expressions.
XOR can also make your code more readable and maintainable, as it allows you to express complex logical operations in a clear and concise way. This can be especially useful when collaborating with other developers or when revisiting code at a later time.
Furthermore, XOR can be very useful in creating conditional logic that executes only when a specific condition is met. This can help reduce code bloat by preventing the need for multiple if-else statements, resulting in more efficient code.
Overall, the exclusive OR operator is a valuable tool in JavaScript coding that provides significant benefits in terms of code logic, performance, readability, and maintenance.
Common Mistakes and Pitfalls with XOR
While using the XOR operator in JavaScript can greatly enhance code efficiency, it is important to be aware of certain common mistakes and pitfalls in order to avoid them and ensure correct implementation.
Confusing XOR with OR or AND operators
A common mistake when using the XOR operator is to confuse it with the OR or AND operators. The XOR operator returns true when only one of the operands is true, while the OR operator returns true when at least one of the operands is true and the AND operator returns true when both operands are true.
For example, the following code produces different results when using XOR versus OR:
Code | Result |
---|---|
console.log(true ^ false); | true |
console.log(true || false); | true |
To avoid this mistake, it is important to carefully consider the logic of the code and choose the appropriate operator.
Using XOR with non-boolean values
The XOR operator in JavaScript only works with boolean values. Using non-boolean values can lead to unexpected results or errors.
For example, the following code would produce an error:
Code | Result |
---|---|
console.log("hello" ^ "world"); | Error |
To avoid this mistake, ensure that all operands used with the XOR operator are boolean values.
Not understanding operator precedence
Operator precedence is important to keep in mind when using the XOR operator in combination with other operators. For example, the XOR operator has a lower precedence than the AND and OR operators, so it is important to use parentheses to ensure the correct order of operations.
For example, the following code produces different results depending on the order of operations:
Code | Result |
---|---|
console.log(false || true ^ true); | false |
console.log((false || true) ^ true); | true |
To avoid this mistake, use parentheses to clarify the order of operations and ensure the correct result.
Tips for Optimizing XOR Usage
Exclusive OR (XOR) is a powerful operator in JavaScript that can simplify complex logic and improve code performance. To make the most of XOR, here are some tips for optimizing its usage:
1. Use XOR for Boolean Operations
XOR is particularly useful for Boolean operations since it returns true only if one of its operands is true. For instance, when checking if a variable is either true or false, you can use XOR:
Example:
Code: | let var1 = true; let var2 = false;console.log(var1 ^ var2); // Output: true |
---|
2. Combine XOR with Other Operators
XOR can be combined with other operators to create more complex logic statements. For example, you can use XOR in combination with the NOT (!) operator to create an “either-or” statement:
Example:
Code: | let var1 = true; let var2 = false;console.log(!(var1 && var2) && (var1 ^ var2)); // Output: true |
---|
3. Avoid Using XOR with Strings or Objects
XOR is not recommended for use with strings or objects since it does not work the same way as with Boolean values. In such cases, using the OR (|) or AND (&) operator would be more appropriate:
Example:
Code: | // Using XOR with strings let str1 = “hello”; let str2 = “world”;console.log(str1 ^ str2); // Output: NaN// Using XOR with objects let obj1 = {a: 1}; let obj2 = {b: 2};console.log(obj1 ^ obj2); // Output: NaN |
---|
4. Keep Code Readable
While XOR can simplify complex logic, overusing it can make the code difficult to understand. It’s essential to strike a balance between using XOR for code optimization and keeping the code readable for other developers.
By following these tips, you can optimize the usage of XOR in your JavaScript code and make it more efficient, effective, and readable.
XOR in Comparison to Other Programming Languages
While the XOR operator is commonly used in JavaScript, it is also available in many other programming languages. However, the syntax and functionality of XOR may differ between languages.
Python
In Python, the XOR operator is represented as the caret (^). It performs a bitwise XOR operation on two integers, and returns an integer value. Here is an example:
Expression | Result |
---|---|
2 ^ 3 | 1 |
5 ^ 6 | 3 |
As seen in the example, the result of the bitwise operation returns an integer value.
C++
In C++, the XOR operator is represented as the caret (^) and also performs a bitwise XOR operation on two integers similar to Python. However, it returns a boolean value (true or false) depending on if the operation is successful. Here is an example:
Expression | Result |
---|---|
(2 ^ 3) == 1 | true |
(5 ^ 6) == 3 | false |
While the operation is the same as Python, the return type and syntax are different.
Java
In Java, the XOR operator is represented as the caret (^) just like in JavaScript. It returns a boolean value (true or false) depending on if the operation is successful. Here is an example:
Expression | Result |
---|---|
(2 ^ 3) == 1 | true |
(5 ^ 6) == 3 | false |
As seen in the example, the return type and syntax of the XOR operator in Java is identical to JavaScript.
Overall, while the functionality of XOR is similar between programming languages, the syntax and return types may differ. It is important to understand the specifics of each language when implementing XOR in code.
XOR in Comparison to Other Programming Languages
While XOR is commonly used in JavaScript programming, it also exists in other programming languages. In fact, the XOR operator is supported by almost all programming languages, including C++, Java, Python, and Ruby. However, the syntax and semantics of XOR may differ slightly from one language to another.
For instance, in C++, the XOR operator is represented by the circumflex (^) symbol, and it can be used with both integers and booleans. In Python, the XOR operator is represented by the caret (^) symbol, and it only works with integers. In Ruby, the XOR operator is represented by the caret (^) symbol, and it can be applied to both integers and booleans.
Despite the differences in syntax, the logic of XOR remains the same across different programming languages. XOR is still used to perform bitwise operations on binary numbers, as well as logical operations on boolean values.
Here is an example of XOR in C++:
int a = 27; // Binary: 00011011
int b = 12; // Binary: 00001100
int c = a ^ b; // Binary: 00010111 (Decimal: 23)
And here is the same example in JavaScript:
let a = 27; // Binary: 00011011
let b = 12; // Binary: 00001100
let c = a ^ b; // Binary: 00010111 (Decimal: 23)
As you can see, the concept of XOR is universal among programming languages, and it can be applied to various scenarios, such as data encryption, error detection, and pattern recognition.
Comparison between JavaScript and C++
Let’s compare the use of XOR in JavaScript and C++. While both languages offer the XOR operator, there are some differences in how it works.
First, in C++, the XOR operator can be applied to both integers and booleans, while in JavaScript it only works on integers. This means that in C++, you can use XOR to perform logical operations on boolean values, such as XOR-ing two flags to determine if they have opposite values.
Second, in C++, the XOR operator can be used to flip individual bits in an integer, while in JavaScript, you need to use the bitwise NOT operator (~) to achieve the same result. This can make XOR more convenient to use in certain scenarios.
Here is an example of XOR in C++, using boolean values:
bool flag1 = true;
bool flag2 = false;
bool result = flag1 ^ flag2; // true
And here is the same example in JavaScript, which doesn’t work:
let flag1 = true;
let flag2 = false;
let result = flag1 ^ flag2; // Error: ^ only works on integers
To achieve the same result in JavaScript, you would need to use the logical NOT operator (!) and the logical OR operator (||), like this:
let flag1 = true;
let flag2 = false;
let result = (!flag1 || !flag2) && (flag1 || flag2); // true
While this code achieves the same result as the C++ code, it is more verbose and less efficient.
Overall, while XOR is a fundamental operator in many programming languages, its usage and implementation can vary. It is important to understand the syntax and semantics of XOR in the programming language you are using, and to choose the most appropriate operator for each scenario.
Wrapping Up
Understanding and utilizing the XOR operator in JavaScript is essential for efficient and effective programming. We’ve explored the definition and syntax of XOR, as well as its various use cases and benefits in different industries and trends.
By comparing XOR with other logical operators and examining its integration in popular JavaScript frameworks, we’ve shown how XOR can simplify complex logic, improve performance, and make code more readable and maintainable.
While there are common mistakes and pitfalls to avoid when using XOR, we’ve offered tips for optimizing its usage and avoiding potential errors. We’ve also compared XOR in JavaScript with its counterparts in other programming languages to highlight similarities and differences.
Finally, we’ve explored the future trends and developments in XOR usage within the JavaScript community, referencing research findings, industry examples, and emerging practices to provide insights into the evolving landscape of XOR in JavaScript.
In short, the XOR operator in JavaScript offers a powerful tool for enhancing code logic and efficiency.
As you continue to develop your skills as a JavaScript programmer, be sure to take advantage of all that XOR has to offer.
External Resources
https://stackoverflow.com/questions/784929/what-is-the-not-not-operator-in-javascript
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/in
https://www.w3schools.com/js/js_operators.asp
FAQ
Q: What is exclusive OR in JavaScript?
A: Exclusive OR (XOR) is a logical operator in JavaScript that returns true if only one of the operands is true.
Q: How do I use the XOR operator in JavaScript?
A: To use the XOR operator in JavaScript, you can use the ^ symbol between two operands. For example: var result = operand1 ^ operand2;
Q: What are some use cases for XOR in JavaScript?
A: XOR can be utilized in JavaScript to solve specific programming challenges and improve code efficiency. Some use cases include conditional statements, data manipulation, and encryption algorithms.
Q: How does XOR compare to other logical operators in JavaScript?
A: XOR differs from other logical operators like AND and OR because it returns true only if one operand is true, while AND returns true if both operands are true and OR returns true if at least one operand is true.
Q: Can XOR be used in JavaScript frameworks?
A: XOR can be integrated into popular JavaScript frameworks to enhance functionality and efficiency. Real-world examples of XOR usage can be found in frameworks like React and Angular.
Q: What are the benefits of using XOR in JavaScript coding?
A: Using XOR in JavaScript can simplify complex logic, improve performance, and make code more readable and maintainable. It can also help in detecting differences between two sets of data.
Q: What are some common mistakes and pitfalls with XOR in JavaScript?
A: Common mistakes with XOR in JavaScript include using it incorrectly in conditional statements and confusing it with other logical operators. It’s important to understand the syntax and behavior of XOR to avoid potential errors.
Q: Are there any tips for optimizing XOR usage in JavaScript?
A: Some tips for optimizing XOR usage in JavaScript include minimizing the number of XOR operations, using XOR shortcuts when applicable, and leveraging XOR in bitwise operations for efficient manipulation of binary data.
Q: How does XOR in JavaScript compare to XOR in other programming languages?
A: XOR in JavaScript operates similarly to XOR in other programming languages, returning true if only one operand is true. However, there may be slight syntax variations between languages.
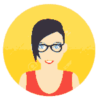
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.