Angular is a popular web development framework that has rapidly gained traction in recent years. However, like any other technology, it comes with its own set of challenges.
From complex data binding to testing, there is a range of issues that developers must navigate in their Angular projects. Even with its many advantages, such as its versatility and scalability, Angular presents unique challenges that can affect the performance and functionality of your applications.
Join us as we dive into the common challenges of Angular development, compare it to other popular frameworks, and provide best practices and tips for overcoming these challenges.
Keep reading to discover how to overcome these difficulties in Angular development.
Angular vs. Other Frameworks
Developers have a plethora of options when it comes to choosing a JavaScript framework. However, comparing the unique features and challenges faced by each framework can help developers make informed decisions.
Angular vs. React
React is a popular JavaScript library created by Facebook. It is a lightweight library designed for building user interfaces. Angular, on the other hand, is a full-fledged framework with a steep learning curve but more comprehensive out-of-the-box features. Here’s a code example showcasing the differences in syntax:
React example:
React Code |
---|
|
Angular example:
Angular Code |
---|
|
As seen in the code examples, React uses a more concise syntax, while Angular’s syntax is more verbose. Developers should consider the complexity of their application and the team’s skill level before choosing a framework.
Angular vs. Vue
Vue is another popular JavaScript framework designed for building user interfaces. It is known for its simplicity and ease-of-use. Here’s a code example showcasing the differences in syntax:
Vue example:
Vue Code |
---|
|
Angular example:
Angular Code |
---|
|
As seen in the code examples, Vue uses a template-based approach to display data, while Angular uses a component-based approach. Vue’s syntax is more similar to HTML, which may be more familiar to front-end developers.
When selecting a framework, developers should consider the project’s requirements, the team’s skill level, and the framework’s ecosystem support.
Performance Optimization
Angular is known for its robustness and flexibility, but this can come at a cost in terms of performance. As Angular applications grow in complexity and size, performance can become a significant challenge for developers. Here are some performance optimization techniques that can help overcome these challenges:
1. Lazy Loading
Lazy loading is a technique that allows modules to be loaded on demand, rather than all at once when the application starts. This can significantly reduce the initial load time and improve the overall performance of the application. To implement lazy loading in Angular, simply use the loadChildren property in the routing module.
2. Change Detection Strategies
Angular’s default change detection strategy is quite powerful, but it can also be time-consuming. This is especially true for large applications with complex data models. To optimize performance, consider using a different change detection strategy, such as OnPush. This strategy only triggers change detection when there is a change to the @Input() properties of a component. This can lead to a significant improvement in performance.
3. Minification and Ahead-of-Time Compilation
Minification is the process of removing all unnecessary characters and whitespace from code to reduce its size. This can have a big impact on the initial load time of an application. Additionally, ahead-of-time (AOT) compilation can further improve performance by pre-compiling templates and removing the need for just-in-time (JIT) compilation at runtime. This can lead to much faster load times and overall better performance.
4. Avoiding Unnecessary DOM Manipulation
DOM manipulation can be a costly operation, especially for large applications. To improve performance, try to minimize the number of DOM manipulations your application makes. Consider using the ngIf directive instead of ngShow or ngHide, as it removes elements from the DOM instead of just hiding them. Additionally, consider using trackBy to minimize the number of changes that need to be made to the DOM.
5. Code Optimization
Finally, it’s important to optimize your code as much as possible. This includes things like reducing the number of HTTP requests made by the application, caching data where possible, and using efficient algorithms and data structures. Additionally, it’s important to keep up-to-date with industry trends and best practices to ensure your code is as optimized as possible.
Complex Data Binding Challenges in Angular Development
One of the most significant challenges developers often face during Angular development is managing complex data binding. While Angular’s two-way data binding is a powerful feature, it can be challenging to handle complex data models and large data sets efficiently.
Developers must understand the intricacies of data binding in Angular and how it works under the hood. By doing so, they can identify and address the challenges that arise when working with complex data models.
Common Complex Data Binding Issues
Developers may encounter several issues when working with complex data models in Angular, including:
- Performance degradation due to excessive data binding
- Unintended side effects from chained bindings
- Problems with shared state between components
Addressing these challenges requires a deep understanding of the intricacies of data binding. Here’s a look at how to handle each issue:
Performance Optimization Techniques for Complex Data Binding
When working with complex data models in Angular, it’s crucial to optimize performance to ensure the application remains fast and responsive. Here are some best practices for optimizing performance:
- Minimize the number of bindings by creating smaller components that focus on specific functionality.
- Use one-time binding to avoid excessive updates.
- Defer initialization of complex components until they are needed, and then tear them down when they are no longer required.
By applying these techniques, developers can overcome performance issues associated with complex data binding.
Handling Chained Bindings
Chained bindings can lead to unintended consequences when updating the data model. Each binding triggers a cycle of updates, which can become unwieldy when chaining many of them together.
To overcome this challenge, developers can:
- Minimize the number of chained bindings, where possible.
- Use object references instead of primitive data types when binding objects to components.
By using these techniques to minimize chained bindings, developers can maintain control over their data model and avoid any unintended side effects.
Managing Shared State between Components
Sharing data between components can lead to complex data binding issues, as changes made in one component may not propagate correctly across the application. Developers should employ the following techniques to manage shared state:
- Use a service to manage shared data between components.
- Employ change detection strategies to ensure the data model updates correctly when shared data changes.
By using these techniques, developers can manage shared state and prevent complex data binding issues from arising.
Overall, managing complex data binding in Angular can be challenging, but by understanding the common issues and employing appropriate techniques, developers can overcome these challenges and build robust, efficient applications.
Routing and Navigation – Overcoming Challenges in Angular Development
Routing and navigation are essential components of any web application, and Angular provides powerful features for implementing them. However, developers often face several challenges while working on routing and navigation in Angular.
One of the common difficulties is handling complex routing scenarios, such as nested or lazy-loaded routes, and passing parameters between routes. To address this, Angular provides various routing techniques such as child routes, query parameters, and route resolvers. For example, child routes allow developers to define nested routes within a parent route, while route resolvers enable loading data before navigating to a route.
Another challenge is optimizing application performance while using routing. Angular’s routing module generates a separate bundle for each route, which can impact overall application performance. Developers can overcome this by using lazy-loading, which loads specific modules only when needed, reducing initial load time and improving performance.
Developers also face issues with navigation, such as navigating between tabs and ensuring that the proper route is displayed on page refresh. To handle this, Angular provides location strategies such as PathLocationStrategy and HashLocationStrategy. The former uses the actual URL path, while the latter uses a hash symbol in the URL to handle client-side routing.
Practical Tips for Overcoming Routing and Navigation Challenges in Angular
- Use route resolvers to load data prior to navigating to a route, improving user experience.
- Lazy-load modules to optimize application performance, reducing initial load time.
- Use location strategies such as HashLocationStrategy to handle navigation and page refresh issues.
- Implement guards to prevent unauthorized access to specific routes or modules.
- Consider using third-party libraries such as Angular Router Extensions to extend routing functionality.
By following these tips and best practices, developers can overcome routing and navigation challenges in Angular and deliver high-quality web applications that provide an optimal user experience.
Testing Angular Applications
Testing is an essential aspect of Angular development. However, it can be challenging to test Angular applications due to the complexity of the framework and the various testing frameworks available.
Types of Angular Tests
Angular supports various types of tests, including unit tests, integration tests, and end-to-end tests. Each type of test has its unique challenges, such as setting up dependencies for unit testing and handling asynchronous operations in end-to-end testing.
Unit tests are performed on individual units of code to ensure that they function as expected. These tests are generally quicker to execute and provide instant feedback. Integration tests, on the other hand, are performed on multiple units of code to verify that they work together as expected.
End-to-end tests cover the entire application’s functionality and test the user’s interaction with the application. These tests are slower to execute, and issues can be harder to detect and diagnose.
Testing Frameworks
Angular supports several testing frameworks, including Jasmine, Karma, and Protractor. Jasmine is a popular behavior-driven development (BDD) testing framework that provides an expressive syntax for writing tests. Karma is a test runner that executes tests in real browsers, providing an accurate representation of how the application will function in production. Protractor is an end-to-end testing framework built specifically for Angular applications.
Choosing the right testing framework depends on the type of test being performed and the developer’s preferences. It is essential to stay up-to-date with the latest testing frameworks and industry trends to ensure that your tests are effective.
Best Practices for Angular Testing
Effective testing requires a structured approach and adherence to best practices. Some best practices for testing Angular applications include:
- Organizing tests into meaningful categories
- Mocking or stubbing dependencies to isolate tests and improve speed
- Using beforeEach and afterEach hooks to set up and tear down tests
- Using async and fakeAsync functions to handle asynchronous operations in tests
- Writing tests early in the development process to catch issues early
By following best practices and staying up-to-date with the latest testing frameworks and trends, Angular developers can overcome the challenges associated with testing and ensure that their applications are reliable and bug-free.
Dependency Injection Challenges in Angular Development
Dependency injection is a powerful design pattern used in Angular to achieve loose coupling and better code organization. However, it can also be a challenging aspect of Angular development, especially for beginners.
Understanding Dependency Injection in Angular
Dependency injection (DI) is a design pattern used to achieve separation of concerns between components in an application. It allows components to be loosely coupled, resulting in better code organization, easier testing, and improved modularity. In Angular, DI is facilitated through the use of providers, which are registered with an injector, and then injected into components or services as needed.
Common Challenges with Dependency Injection in Angular
One of the most common challenges of DI in Angular is understanding the hierarchy of injectors. Angular uses a hierarchical injection system, where each component has its own injector, and child components inherit injectors from their parent components. This can lead to unexpected behavior when providers are registered at different levels of the injector hierarchy.
Another challenge is managing the scope of injected services. By default, Angular will create a new instance of a service for each component that injects it, which can lead to unintended consequences if not managed properly. Additionally, circular dependencies between components or services can cause issues and make it difficult to manage the dependency graph.
Best Practices for Overcoming DI Challenges
To overcome these challenges, it is important to follow best practices when using dependency injection in Angular. Firstly, it is recommended to register providers at the highest level possible in the component hierarchy, to avoid unexpected behavior due to conflicting providers.
Secondly, it is important to understand the scope of injected services and use the appropriate DI pattern (such as singleton or factory) to manage their lifecycle. Circular dependencies can be avoided by refactoring code to eliminate them, or by using the @Optional decorator to allow for undefined dependencies.
In conclusion, while dependency injection can be a challenging aspect of Angular development, following best practices and understanding the underlying concepts can help overcome these difficulties and lead to more robust and maintainable code.
Mobile Development with Angular
Developing mobile applications with Angular can be challenging due to the unique considerations involved with mobile devices. Responsive design is crucial, as mobile devices come in various screen sizes and resolutions. Additionally, performance considerations are important due to limited resources on mobile devices.
One way to address these challenges is by using Angular’s built-in mobile-specific features, such as Ionic and NativeScript. Ionic is a popular framework for building mobile applications using web technologies, while NativeScript allows you to build native mobile apps using Angular. Both frameworks provide a set of pre-built UI components optimized for mobile devices that help streamline the development process.
Another way to improve mobile development with Angular is by optimizing performance. This can be achieved through techniques such as lazy loading, which delays loading non-critical resources until needed, and tree shaking, which eliminates unused code to reduce bundle sizes.
It is important to keep up with industry trends and best practices when developing mobile applications with Angular. The Angular team regularly updates the framework with performance and mobile-specific enhancements, making it important to stay up-to-date with the latest improvements.
Overcoming Angular Challenges
As we have discussed throughout this article, Angular development poses several challenges for developers. However, with the right strategies and best practices, these challenges can be overcome. Here are some practical solutions to help you overcome Angular development challenges:
Make Use of Industry Best Practices
The Angular community is vibrant and constantly growing. As a result, there are many best practices and industry trends to follow when developing Angular applications. By staying up-to-date with these trends, you can ensure that your application is optimized for performance, security, and maintainability.
For example, one industry trend is to use lazy loading to improve application performance. By loading only the necessary components, your application can load faster and function more smoothly. Another trend is to use server-side rendering for faster initial loading times and better search engine optimization (SEO).
Optimize for Performance
Performance optimization is a critical aspect of Angular development. By paying attention to factors such as file size and API calls, you can improve your application’s speed and responsiveness. Here are some tips:
- Minimize the size of your JavaScript and CSS files.
- Avoid using too many API calls, and make use of caching when possible.
- Use lazy loading to load only the necessary components.
- Optimize images and videos to reduce file size.
Use Effective Testing Strategies
Testing is an essential part of Angular development. By using effective testing strategies, you can ensure that your application is bug-free and functions as intended. Here are some tips:
- Use unit testing to test individual components.
- Use end-to-end (E2E) testing to test the entire application workflow.
- Use continuous integration and continuous delivery (CI/CD) to automate testing and deployment processes.
Take Advantage of Dependency Injection
Dependency injection is a powerful tool in Angular development. By allowing components to share services and data, your application can be more modular and easier to maintain. Here are some tips:
- Use services to share data between components.
- Use providers to configure your application’s dependencies.
- Make use of Angular’s built-in dependency injection.
By following these tips and best practices, you can overcome the common challenges of Angular development and create high-quality applications.
Remember, the Angular community is always growing and evolving, so stay up-to-date with new trends and best practices to ensure that your applications are always optimized and up-to-date.
External Resources
https://angular.io/tutorial/first-app
https://angular.io/start/start-data
FAQ
Q: What are the challenges in Angular development?
A: Angular development can present various difficulties, including performance optimization, complex data binding, routing and navigation, testing, dependency injection, and mobile development.
Q: How does Angular compare to other frameworks?
A: Angular has its unique challenges and advantages compared to other popular frameworks. Code examples in both Angular and other frameworks will be provided to showcase the differences.
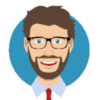
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.