If you are working with AngularJS, you have likely come across the angular.extend method. This method is an essential tool for developers who want to extend objects in their AngularJS applications.
The angular.extend method allows you to combine two or more objects into a single object. This feature is especially useful when you want to add new properties or methods to an existing object without overwriting any of its existing properties. With the angular.extend method, you can quickly and easily extend objects in your AngularJS application.
Using angular.extend in your AngularJS application can save you time and improve your code’s reusability..
What is the Angular.extend Method?
The Angular.extend method is a powerful tool used in AngularJS development for extending objects. It allows developers to combine properties from two or more objects into a single object, simplifying the process of creating and managing complex objects. This method is part of the AngularJS core and is widely used by developers around the world.
Using the Angular.extend method, it is possible to add new properties and values to an existing object while preserving the original properties and values. This can be incredibly useful when working with large and complex objects, as it allows developers to modify and extend these objects without having to rewrite the entire code.
The syntax of the Angular.extend method is straightforward, with two or more objects being passed as arguments. Once invoked, the method will combine the properties of the objects and return a new object with the updated values.
Here is an example of how the Angular.extend method can be used in AngularJS:
// Define two objects var object1 = { name: 'John', age: 28 }; var object2 =
{ gender: 'Male' };
// Extend the first object with the properties of the second object
var extendedObject = angular.extend(object1, object2);
// Output the extended object console.log(extendedObject);
In this example, the Angular.extend method is used to extend the object1 with the properties of the object2. The resulting extendedObject contains all the properties of both objects:
{ name: 'John', age: 28, gender: 'Male' }
As you can see, the Angular.extend method is a versatile and powerful tool that can be used in a wide variety of scenarios. Whether you are working with simple or complex objects, this method can simplify the process of creating and managing them, making AngularJS development faster and more efficient.
Extending Objects with Angular.extend
Now that we understand what the Angular.extend method is and how it works, let’s explore how we can use it to extend objects in AngularJS.
The basic syntax for using Angular.extend to extend an object is as follows:
angular.extend(destination, source);
The destination parameter is the object to be extended, and the source parameter is the object from which the properties will be copied.
Let’s take a look at a simple example:
var obj1 = {name: "John"}; var obj2 = {age: 30}; angular.extend(obj1, obj2);
console.log(obj1); // Output: {name: "John", age: 30}
In this example, we created two objects, obj1 and obj2. We then used the angular.extend() method to copy the properties of obj2 into obj1, effectively extending obj1.
We can also use the Angular.extend method to extend objects with multiple sources:
var obj1 = {name: "John"}; var obj2 = {age: 30}; var obj3 = {city: "New York"};
angular.extend(obj1, obj2, obj3); console.log(obj1); // Output: {name: "John",
age: 30, city: "New York"}
In this example, we used the angular.extend() method to copy the properties of obj2 and obj3 into obj1, effectively extending obj1 with multiple sources.
The Angular.extend method can also be used to create a deep copy of an object. When used in this way, the method ensures that all nested objects are copied by value, rather than by reference:
var obj1 = {name: "John", address: {street: "123 Main St", city: "New York"}};
var obj2 = angular.extend({}, obj1);
console.log(obj2); // Output: {name: "John", address:
{street: "123 Main St", city: "New York"}}
In this example, we created an object obj1 with a nested object address. We then used the angular.extend() method to create a deep copy of obj1 and stored the result in obj2. Notice that the nested object address is copied by value, ensuring that changes to obj2.address do not affect obj1.address.
These are just a few examples of how the Angular.extend method can be used to extend objects in AngularJS. By leveraging this powerful tool, developers can greatly simplify their code and improve code reusability.
Advantages of Using Angular.extend
There are many advantages to utilizing the Angular.extend method in your AngularJS development.
Here are some of the key benefits:
- Code Reusability: One of the main advantages of using Angular.extend is that it allows you to reuse objects that you have already defined in your code. This can save a lot of time and effort, especially when working on larger projects.
- Easy to Use: Angular.extend is a very straightforward method to use, with a simple syntax that is easy to understand and implement in your code. This makes it a great tool for developers of all skill levels.
- Enhanced Flexibility: By using Angular.extend to extend objects, you can add or modify properties on the fly, without having to define a new object. This gives you much more flexibility and control over your code.
- Improved Performance: Angular.extend is a very lightweight method, which means that it can be used in your code without adding unnecessary overhead. This can help to improve the performance of your application.
- Increases Maintainability: By using Angular.extend, you can keep your code more organized and easier to maintain, as it allows you to group related properties together in a single object.
By taking advantage of these benefits, you can significantly improve the quality and efficiency of your AngularJS development projects.
Comparison with Other Object Extension Methods
While the Angular.extend method is a powerful tool for extending objects in AngularJS, it is not the only option available. Other object extension methods exist in AngularJS and other frameworks, and each has its own strengths and weaknesses.
One notable alternative is the jQuery.extend method, which can also be used in AngularJS applications. While jQuery.extend operates similarly to Angular.extend, it does not support deep merging like Angular.extend does.
In addition, jQuery.extend is dependent on the jQuery library, whereas Angular.extend is built into AngularJS.
Another option for object extension is the Object.assign method, which is a part of the ECMAScript 2015 (ES6) standard. Object.assign is designed to copy the values of all enumerable properties from one or more source objects to a target object.
While Object.assign can accomplish similar tasks to Angular.extend, it is not as versatile in terms of deep merging and is not supported in older browsers.
When comparing Angular.extend to other object extension methods, it is important to consider the specific needs of your project and choose the method that best aligns with those needs. In some cases, using a combination of methods may be necessary to achieve the desired outcome.
Method | Pros | Cons |
---|---|---|
Angular.extend | Supports deep merging, built into AngularJS | Not as widely supported in other frameworks, may require additional syntax for certain tasks |
jQuery.extend | Extensive library support, can be used in AngularJS applications | Does not support deep merging, dependent on jQuery library |
Object.assign | Part of ES6 standard, can be used in modern browsers | Not as versatile for deep merging, not supported in older browsers |
In any case, it is best to be familiar with multiple object extension methods in order to choose the one that best fits the task at hand.
Best Practices for Using Angular.extend
While the Angular.extend method can simplify the process of object extension in AngularJS, it’s important to use it correctly to avoid common mistakes and optimize performance.
Here are some best practices for using Angular.extend:
- Avoid excessive nesting: While nesting can make your code more organized, too much nesting can make it difficult to read and understand. Keep your code clean and readable by limiting nesting to no more than two levels.
- Use caution with deep copies: When using Angular.extend to create a deep copy of an object, be aware that it can be memory-intensive and slow. Whenever possible, try to use shallow copying instead.
- Optimize performance: Use the Angular.extend method sparingly and only when needed. When working with large objects or arrays, consider using other techniques like concatenation or pushing to achieve the same result.
By following these best practices, you can make the most of the Angular.extend method and improve the quality and performance of your AngularJS applications.
Common Mistakes to Avoid
While using the Angular.extend method can simplify the task of extending objects in AngularJS, there are some common mistakes that developers can make while using it.
1. Incorrect Usage
One of the most common mistakes is incorrect usage of the method. This can happen when developers try to extend an object that does not exist or when they try to extend multiple objects at once.
Incorrect usage can result in errors that are difficult to debug, so it’s important to validate the objects being extended before using the method.
2. Potential Pitfalls
Another common mistake is failing to consider potential pitfalls that may arise during object extension.
For example, if two objects have properties with the same name, the properties in the second object will overwrite the properties in the first object. This can lead to unexpected behavior and should be avoided by carefully checking for property conflicts before extending an object.
3. Performance Considerations
Finally, it’s important to consider performance when using the Angular.extend method. Extending large objects or nesting extensions can result in slow performance and increased memory usage.
To avoid these issues, it’s recommended to limit the depth of object nesting and to use the method sparingly when working with large objects or arrays.
By avoiding these common mistakes, developers can use the Angular.extend method effectively and improve their AngularJS development workflow.
Advanced Techniques with Angular.extend
While the Angular.extend method is a powerful tool for extending objects in AngularJS development, there are several advanced techniques that can take this functionality to the next level. Let’s explore some in-depth below:
Deep Merging
Deep merging refers to the process of merging two or more objects deeply, including nested properties. With Angular.extend, deep merging can be achieved by passing an additional object as a parameter. The resulting object will contain a combination of all the properties of the objects passed in, with any conflicts resolved in favor of the last object.
Here’s an example:
// Create the initial object
var parentObj = {
name: "John",
age: 30,
address: {
street: "123 Main St",
city: "Anytown",
state: "CA"
}
};
// Create the object to merge
var childObj = {
name: "Jane",
address: {
city: "Othertown"
}
};
// Merge the objects
var mergedObj = angular.extend({}, parentObj, childObj);
// Resulting object:
//{
// name: "Jane",
// age: 30,
// address: {
// street: "123 Main St",
// city: "Othertown",
// state: "CA"
// }
//}
Combining Multiple Objects
Angular.extend can also be used to combine multiple objects into a single object. This can be achieved by passing all the objects to be combined as parameters in a single call to the method.
Here’s an example:
// Create the objects to combine
var obj1 = {
a: 1,
b: 2
};
var obj2 = {
c: 3,
d: 4
};
var obj3 = {
e: 5,
f: 6
};
// Combine the objects
var combinedObj = angular.extend({}, obj1, obj2, obj3);
// Resulting object:
//{
// a: 1,
// b: 2,
// c: 3,
// d: 4,
// e: 5,
// f: 6
//}
Handling Edge Cases
Finally, it’s important to consider edge cases when using Angular.extend. For example, when extending an object with a null or undefined value, the method will throw an error.
To prevent this, you can check for the existence of the object before attempting to extend it. Similarly, when extending objects that contain circular references, you may need to use a custom cloning function to avoid infinite recursion.
These edge cases should be handled with care to ensure proper functioning of your code.
By mastering these advanced techniques, you can take full advantage of the power and flexibility of the Angular.extend method in your AngularJS development projects.
Final Thoughts
Overall, the Angular.extend method is a crucial tool for developers working with AngularJS. It simplifies the process of extending objects and improves code reusability, making it an essential tool for any AngularJS project.
By following best practices and avoiding common mistakes, developers can make full use of the method’s capabilities and optimize performance. It’s important to keep an eye on future trends and updates to AngularJS to ensure the method remains relevant and effective in future development.
If you’re working with AngularJS, it’s highly recommended that you start using the Angular.extend method in your projects. With its user-friendly syntax and powerful functionality, it’s sure to enhance your development experience and improve the quality of your code.
External Resources
https://docs.angularjs.org/api/ng/function/angular.extend
FAQ
Q: What is the Angular.extend method?
A: The Angular.extend method is a utility function provided by the AngularJS framework. It allows you to extend an object by adding properties from one or more other objects. This can be useful when you want to combine the properties of multiple objects into a single object.
Q: How do I use the Angular.extend method to extend objects?
A: To use the Angular.extend method, you simply provide the object you want to extend as the first argument, followed by one or more objects that contain the properties you want to add. The method will then add the properties from the other objects to the original object.
Q: What are the advantages of using Angular.extend?
A: Using the Angular.extend method can simplify the process of extending objects in AngularJS. It allows you to easily combine the properties of multiple objects into a single object, improving code reusability and reducing the need for manual property copying.
Q: How does the Angular.extend method compare to other object extension methods?
A: The Angular.extend method is similar to other object extension methods available in AngularJS and other frameworks. However, it has its own syntax and behavior. It’s important to understand the differences between the methods and choose the one that best fits your specific needs.
Q: What are some best practices for using Angular.extend?
A: When using the Angular.extend method, it’s important to avoid excessive nesting and handle deep copies properly. You should also optimize performance by only extending objects when necessary. Following these best practices can help you use the method effectively in your AngularJS development.
Q: What are some common mistakes to avoid when using Angular.extend?
A: Common mistakes when using the Angular.extend method include incorrect usage, potential pitfalls, and performance considerations. It’s important to understand the method’s behavior and potential issues to avoid making these mistakes.
Q: What are some advanced techniques with Angular.extend?
A: Advanced techniques with Angular.extend include deep merging, combining multiple objects, and handling edge cases. By utilizing these techniques, you can take full advantage of the method’s capabilities and solve more complex problems in your AngularJS development.
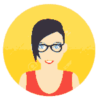
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.