ERP with Node.js revolutionizes business operations with its efficient real-time processing, scalable architecture, and seamless integration, ensuring agile and robust management solutions for startups and enterprises alike.
Enterprise Resource Planning (ERP) is a software system that enables businesses to streamline their operations, manage resources efficiently, and improve their bottom line.
People often dismiss Node.js as being unfit for complex applications such as Enterprise Resource Planning (ERP) systems.
However, this is largely because many developers lack comprehensive experience in using Node.js for such large-scale projects.
What is ERP?
Enterprise Resource Planning (ERP) is a type of software that organizations use to manage and integrate important parts of their businesses. For a CTO at an early startup in the USA, it’s crucial to understand that
ERP systems can help streamline and automate core business processes, such as finance, HR, manufacturing, supply chain, services, procurement, and others, by providing a centralized and integrated platform.
This integration allows for better data visibility, efficiency, and decision-making across the company. ERP systems can be critical in scaling operations, improving productivity, and enhancing the organization’s ability to respond to market changes.
They can be custom-built or purchased as off-the-shelf solutions, and are increasingly offered through cloud-based services, offering scalability and reduced need for in-house IT infrastructure. For a startup, selecting the right ERP system involves considering factors like cost, scalability, industry-specific features, and the ability to integrate with existing systems.
Why Choose Node.js for ERP Development?
Choosing Node.js for ERP development can be beneficial for several reasons:
- Asynchronous and Event-Driven: Node.js operates on a non-blocking, event-driven architecture, making it suitable for handling multiple operations simultaneously. This is particularly beneficial for ERP systems which often require high concurrency and real-time data processing.
- Scalability: Node.js is known for its scalability, which is essential for ERP systems as they need to adapt to the growing needs of a business.
- Performance: Node.js uses the V8 JavaScript engine, which compiles JavaScript directly to native machine code. This results in fast execution, a significant advantage for data-intensive ERP applications.
- Community and Ecosystem: Node.js has a large and active community, providing a vast array of libraries and tools that can speed up ERP development and offer solutions to common problems.
- Cross-Platform Development: Node.js supports cross-platform development, which can be a cost-effective approach for startups wanting to deploy their ERP system across different platforms.
- Unified JavaScript Development: Using JavaScript on both the frontend and backend (Node.js) simplifies development. This uniformity can streamline the development process, as the same team can work on both ends of the application.
Sample Code: Basic Node.js Server
Below is a simple Node.js code sample to create a basic server. This example is basic and would be just a small part of an entire ERP system, but it gives an idea of how Node.js can be used to set up server-side functionality.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, this is a basic Node.js server for an ERP system!');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
To run this code, you would need Node.js installed on your system and the Express.js framework, which can be installed using npm (Node Package Manager):
npm init -y
npm install express
This example is a starting point. Real-world ERP systems would involve more complex functionalities, including database interactions, authentication, real-time data processing, and integration with other business systems and services.
How Node.js Streamlines ERP Processes
Node.js can streamline ERP (Enterprise Resource Planning) processes in several key ways, enhancing the efficiency and performance of these critical business systems:
- Asynchronous I/O Operations: Node.js excels in handling asynchronous input/output operations. This non-blocking nature is particularly useful for ERP systems, which often deal with a large number of concurrent data requests and need to perform multiple operations simultaneously without slowing down.
- Real-Time Data Processing: Node.js is well-suited for real-time data processing and updates, a crucial feature for ERP systems that require immediate reflection of data changes, like inventory levels, financial transactions, and customer interactions.
- Microservices Architecture: Node.js supports a microservices architecture, allowing for the development of smaller, independent modules within the ERP system. This approach can lead to easier maintenance, better scalability, and quicker updates or enhancements to the system.
- Scalability: Node.js’s lightweight and efficient nature makes it easy to scale applications. This is essential for ERP systems as they need to handle growing amounts of data and users as the business expands.
- Unified JavaScript Stack: Using JavaScript for both the front-end and back-end (through Node.js) streamlines the development process. This uniformity can lead to faster development cycles for ERP systems, as developers can work across the entire stack more seamlessly.
- Community and Ecosystem: The extensive Node.js community contributes a vast range of tools and libraries that can accelerate ERP development. These resources can simplify the integration of various functionalities like database management, authentication, and more.
- Cross-Platform Compatibility: Node.js applications can run on various platforms (Windows, Linux, MacOS), ensuring that ERP systems are accessible across different environments without the need for extensive modifications.
- Integration Capabilities: Node.js can easily integrate with various databases, third-party services, and legacy systems, which is vital for ERP systems that often need to communicate with different technologies and platforms within a business.
- Performance Under Load: ERP systems, especially in larger organizations, can experience high traffic and data load. Node.js, known for its good performance under load, can handle these demands efficiently.
Code Sample
Let’s consider a scenario where we’re using Node.js to develop a part of an ERP system, specifically for handling real-time inventory updates. This example will demonstrate asynchronous I/O operations, real-time data processing, and integration with a database (using MongoDB for simplicity).
Prerequisites
- Node.js installed on your system
- MongoDB setup for database operations
- Install necessary Node.js packages:
express
for creating the server andmongoose
for interacting with MongoDB
Sample Code
First, install the necessary packages using npm:
By leveraging these capabilities, Node.js can help create ERP systems that are not only efficient and scalable but also adaptable to the evolving needs of a business.
npm init -y
npm install express mongoose
Here’s a basic Node.js script:
const express = require('express');
const mongoose = require('mongoose');
const app = express();
// MongoDB connection string (replace with your own URI)
const mongoURI = 'mongodb://localhost:27017/erpInventory';
// Connect to MongoDB
mongoose.connect(mongoURI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch(err => console.error(err));
// Schema for Inventory Item
const inventorySchema = new mongoose.Schema({
itemName: String,
quantity: Number,
lastUpdated: { type: Date, default: Date.now }
});
// Model for Inventory
const InventoryItem = mongoose.model('InventoryItem', inventorySchema);
// Middleware to parse JSON
app.use(express.json());
// Route to get all inventory items
app.get('/inventory', async (req, res) => {
try {
const items = await InventoryItem.find();
res.json(items);
} catch (err) {
res.status(500).send(err);
}
});
// Route to update an inventory item
app.put('/inventory/update', async (req, res) => {
try {
const { itemName, quantity } = req.body;
const updatedItem = await InventoryItem.findOneAndUpdate(
{ itemName },
{ quantity, lastUpdated: Date.now() },
{ new: true }
);
res.json(updatedItem);
} catch (err) {
res.status(500).send(err);
}
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation
- This script sets up a basic Express server and connects to a MongoDB database.
- It defines a schema and model for inventory items using Mongoose.
- There are two routes:
GET /inventory
: Retrieves all inventory items.PUT /inventory/update
: Updates the quantity of a specific inventory item.
- The operations are asynchronous, utilizing
async/await
for handling database operations.
How to Run
- Start your MongoDB server.
- Run this script with Node.js.
- Use a tool like Postman or a web browser to interact with the API.
This code is a basic example and would be part of a larger, more complex ERP system. It demonstrates real-time data handling, asynchronous operations, and database interactions, which are crucial in ERP systems.
Code Samples of Node.js vs. Other Languages for ERP Development
When comparing Node.js to other popular languages for ERP development, it’s crucial to consider aspects like performance, scalability, ease of development, and ecosystem. Here, I’ll provide concise examples comparing Node.js with Python and Java, two other commonly used languages in ERP development.
Node.js Example: Real-Time Inventory Update API
Node.js is excellent for handling asynchronous operations and real-time data processing.
// Node.js with Express and MongoDB
const express = require('express');
const app = express();
app.use(express.json());
app.put('/inventory/update', async (req, res) => {
const { itemName, quantity } = req.body;
// Update inventory logic (asynchronous)
res.send(`Inventory updated: ${itemName} - ${quantity}`);
});
app.listen(3000, () => console.log('Server running on port 3000'));
Python Example: Batch Data Processing
Python, with frameworks like Flask or Django, is often praised for its simplicity and readability, suitable for complex business logic.
# Python with Flask
from flask import Flask, request
app = Flask(__name__)
@app.route('/inventory/update', methods=['PUT'])
def update_inventory():
data = request.json
item_name = data['itemName']
quantity = data['quantity']
# Update inventory logic (synchronous)
return f'Inventory updated: {item_name} - {quantity}'
if __name__ == '__main__':
app.run(port=3000)
Java Example: Enterprise-Grade Solution
Java, often used in large-scale enterprise applications, excels in robustness and has a vast ecosystem of tools and libraries.
// Java with Spring Boot
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class ErpApplication {
public static void main(String[] args) {
SpringApplication.run(ErpApplication.class, args);
}
}
@RestController
class InventoryController {
@PutMapping("/inventory/update")
public String updateInventory(@RequestBody InventoryItem item) {
// Update inventory logic
return "Inventory updated: " + item.getItemName() + " - " + item.getQuantity();
}
}
class InventoryItem {
private String itemName;
private int quantity;
// Getters and setters...
}
Comparison:
- Node.js: Ideal for real-time applications and services. It’s lightweight and can handle multiple I/O operations concurrently, which is great for responsive, event-driven parts of an ERP, like real-time inventory updates or user interactions.
- Python: Known for its simplicity and readability. It’s excellent for writing clear, maintainable code, which is beneficial for complex business logic in ERP systems. Python may not match Node.js in handling concurrent operations but is well-suited for batch processing and complex calculations.
- Java: Offers robustness and is widely used in large-scale enterprise environments. Java’s ecosystem provides numerous tools and libraries for building scalable, secure, and maintainable ERP systems. It might be more resource-intensive and verbose compared to Node.js or Python but excels in long-term maintainability and performance optimization.
Your choice should align with your team’s expertise, the specific requirements of your ERP system, and the expected scale of operation.
Node.js could be a more agile and efficient choice for a startup due to its simplicity and speed, while Python and Java might be considered for their established presence in enterprise application development.
Industry Examples of Successful ERP Node.js Implementation
This table provides an overview of what a CTO should consider when deciding on Node.js for their ERP system. Balancing these factors against the startup’s specific needs and resources will guide towards making the best technological choice.
Best Practices for ERP Node.js Development
Implementing best practices in Node.js ERP development is crucial for building a robust, scalable, and maintainable system.
Here are key practices with concise examples:
1. Modular Architecture
Best Practice: Use modular architecture to improve maintainability and scalability. Break down the ERP system into smaller, manageable parts.
Code Sample:
// Example: Modularizing routes in Express
// inventoryRoutes.js
const express = require('express');
const router = express.Router();
router.get('/inventory', (req, res) => {
// Logic for getting inventory
});
router.post('/inventory', (req, res) => {
// Logic for adding to inventory
});
module.exports = router;
// app.js
const express = require('express');
const inventoryRoutes = require('./inventoryRoutes');
const app = express();
app.use('/api', inventoryRoutes);
app.listen(3000, () => console.log('Server running'));
2. Asynchronous Programming
Best Practice: Leverage asynchronous programming to handle I/O operations efficiently.
Code Sample:
// Example: Using async/await for database operations
const getUserData = async (userId) => {
try {
const user = await User.findById(userId);
return user;
} catch (error) {
console.error('Error fetching user:', error);
}
};
getUserData('12345');
3. Error Handling and Logging
Best Practice: Implement comprehensive error handling and logging for debugging and monitoring.
Code Sample:
// Example: Error handling middleware in Express
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
4. Use Environment Variables for Configuration
Best Practice: Store configuration in environment variables to enhance security and flexibility.
Code Sample:
// Example: Using environment variables for database connection
const mongoose = require('mongoose');
const dbURI = process.env.MONGO_URI;
mongoose.connect(dbURI, { useNewUrlParser: true, useUnifiedTopology: true });
5. Implementing Testing
Best Practice: Write tests for your code to ensure reliability and reduce bugs.
Code Sample:
// Example: Basic unit test with Mocha & Chai
const expect = require('chai').expect;
describe('Inventory Module', () => {
it('should add item correctly', () => {
const result = InventoryModule.addItem('newItem', 10);
expect(result).to.be.true;
});
});
6. Optimize for Performance
Best Practice: Profile and optimize your Node.js application for better performance.
Code Sample:
// Example: Using clustering to handle more load
const cluster = require('cluster');
const totalCPUs = require('os').cpus().length;
if (cluster.isMaster) {
for (let i = 0; i < totalCPUs; i++) {
cluster.fork();
}
} else {
const express = require('express');
const app = express();
// Server code...
}
7. Secure Your Application
Best Practice: Prioritize security to protect sensitive ERP data.
Code Sample:
// Example: Using helmet for security headers
const helmet = require('helmet');
app.use(helmet());
These practices strike a balance between development efficiency, application performance, and long-term maintainability, crucial for the success of an ERP implementation.
Conclusion
Node.js is a highly effective choice for ERP development in startups due to its asynchronous, event-driven nature, and scalability. It supports real-time data processing and efficient concurrency management, essential for ERP systems.
The examples and best practices highlight Node.js’s ability to create maintainable, secure, and performance-optimized applications.
Choosing Node.js for ERP development means leveraging a technology that offers development agility and operational efficiency, crucial for a growing business.
This makes Node.js a strategic choice for startups seeking a flexible and scalable ERP solution.
FAQ
1. How can Node.js handle real-time data processing in an ERP system?
Answer: Node.js is well-suited for real-time data processing due to its event-driven architecture. This is crucial in ERP systems for features like inventory management and order processing.
Code Sample:
// Using Socket.IO with Node.js for real-time communication
const app = require('express')();
const server = require('http').createServer(app);
const io = require('socket.io')(server);
io.on('connection', (socket) => {
socket.on('updateInventory', (data) => {
// Handle inventory update
io.emit('inventoryUpdated', data); // Broadcast update
});
});
server.listen(3000);
2. How does Node.js improve scalability in ERP systems?
Answer: Node.js enables horizontal scaling, which is essential for ERP systems as they grow. Its non-blocking I/O model allows handling more concurrent requests with less hardware.
Code Sample:
// Example of clustering in Node.js
const cluster = require('cluster');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
} else {
const express = require('express');
const app = express();
app.get('/', (req, res) => res.send('Hello World!'));
app.listen(3000);
}
3. How can we ensure secure data handling in a Node.js-based ERP system?
Answer: Security in a Node.js ERP system involves implementing best practices like HTTPS, using security middleware like Helmet, and managing dependencies securely.
Code Sample:
// Secure data handling in Express
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet()); // Security middleware
// ...rest of the code
app.listen(3000);
4. What is the best way to manage database operations in Node.js for ERP?
Answer: Using an ORM (Object-Relational Mapping) like Sequelize or Mongoose for MongoDB. This abstracts the database layer, making code more maintainable and scalable.
Code Sample:
// Using Mongoose with MongoDB
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const InventorySchema = new Schema({
itemName: String,
quantity: Number
});
const Inventory = mongoose.model('Inventory', InventorySchema);
// Connect to MongoDB
mongoose.connect('mongodb://localhost/erpSystem');
5. How do we handle asynchronous operations in Node.js for complex ERP workflows?
Answer: Use async/await to handle asynchronous operations. This makes the code easier to read and maintain, especially for complex workflows in an ERP system.
Code Sample:
// Async/Await for handling database operations
const getUser = async (userId) => {
try {
const user = await User.findById(userId);
// Handle user data
} catch (error) {
console.error(error);
}
};
These questions and code samples provide with insights into critical aspects of ERP development using Node.js, highlighting its capabilities in real-time processing, scalability, security, database management, and asynchronous operations.
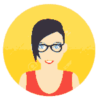
Nicole is a highly accomplished technical author specializing in scientific computer science. With a distinguished career as a developer and program manager at Accenture and Nike, she has showcased exceptional leadership skills and technical expertise in delivering successful projects.
For the past 22 years, Nicole’s unwavering interest has been in JavaScript web development. Since the early days of its release, she has immersed herself in the intricacies of the language, exploring its vast potential and harnessing its capabilities to create dynamic and interactive web solutions. Nicole’s expertise in JavaScript extends to various frameworks and libraries, positioning her as a trusted authority in the field.
As a technical author, Nicole remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. Her expertise in JavaScript web development, coupled with her experience as a developer and program manager, positions her as a valuable resource for professionals seeking guidance and best practices. With each publication, Nicole strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.