TypeScript vs JavaScript: Explore their differences in type checking, flexibility, and use cases. Perfect for developers deciding between TypeScript’s static typing and JavaScript’s dynamic nature for their projects.
JavaScript is an excellent starting point for newcomers to web development, but it has its limitations.
One of its main pitfalls is the lack of static typing, which often results in hard-to-trace bugs in larger applications.
Having transitioned to TypeScript three years ago, I have noticed a significant improvement in code reliability and predictability without losing the flexibility that JavaScript offers.
What is TypeScript?
TypeScript is a programming language developed and maintained by Microsoft. It’s a superset of JavaScript, which means it has all the features of JavaScript plus additional features unique to TypeScript.
The key feature of TypeScript is its static type system, which allows for checking type correctness at compile time.
This helps catch errors early in the development process, making the code more robust and maintainable. TypeScript is particularly favored in large-scale applications due to its enhanced code structuring and object-oriented programming capabilities.
TypeScript’s appeal lies in its ability to facilitate large-scale web application development with a team, ensuring higher code quality and maintainability, which are critical for scaling up.
Here’s a basic example of TypeScript code:
interface User {
name: string;
id: number;
}
const user: User = {
name: "John Doe",
id: 0,
}
function getUserInfo(user: User): string {
return `User Name: ${user.name}, User ID: ${user.id}`;
}
console.log(getUserInfo(user));
This code snippet defines an interface User
, ensuring that any user object conforms to the structure with a name
as a string and an id
as a number. The function getUserInfo
takes a User
type object and returns a formatted string. TypeScript’s type system ensures that the correct types are used throughout the code, reducing runtime errors.
What is JavaScript?
JavaScript is a high-level, dynamic programming language that is widely used for developing interactive and responsive web applications.
It’s an essential component of the web technology stack, alongside HTML and CSS, and runs in the web browser, enabling client-side script to interact with the user, control the browser, and alter the document content that is displayed.
JavaScript is versatile and supports imperative, object-oriented, and functional programming styles.
JavaScript’s significance lies in its universality across web platforms and its ability to create rich, engaging user experiences.
It’s a cornerstone in modern web development, essential for front-end development and increasingly popular for back-end development with the advent of Node.js.
Here’s a simple example of JavaScript code:
function greet(name) {
alert("Hello, " + name);
}
greet("Alice");
In this example, the greet
function takes a name as an argument and displays a greeting using the alert
function. This code represents the basic structure and syntax of JavaScript and demonstrates its use in creating dynamic interactions in web applications.
Key Differences between TypeScript and JavaScript
While TypeScript and JavaScript share many similarities, they also have some fundamental differences that set them apart.
Here’s a table outlining the key differences between TypeScript and JavaScript, along with code samples to illustrate these differences:
Aspect | TypeScript | JavaScript |
---|---|---|
Type System | Static type checking. Types are defined at compile time. | Dynamic type checking. Types are resolved at runtime. |
Superset | TypeScript is a superset of JavaScript. | JavaScript is the core language TypeScript builds upon. |
Compilation | Needs to be compiled into JavaScript before execution. | Can be run directly in browsers or Node.js environments. |
Type Annotation | Supports type annotations for variables, function parameters, etc. | No native support for type annotations. |
Interfaces and Generics | Supports interfaces and generics for complex type definitions. | Lacks native support for interfaces and generics. |
Error Checking | Provides early error detection at compile-time. | Errors are typically found at runtime. |
Adoption | Widely used in large-scale applications for better maintainability. | Universally used for web development, both client and server-side. |
Community and Ecosystem | Growing, with strong support for large-scale applications. | Vast and well-established, with a rich ecosystem of libraries. |
TypeScript Code Sample:
interface User {
name: string;
id: number;
}
const user: User = {
name: "John Doe",
id: 1
};
function getUserInfo(user: User): string {
return `Name: ${user.name}, ID: ${user.id}`;
}
JavaScript Code Sample:
function getUserInfo(user) {
return `Name: ${user.name}, ID: ${user.id}`;
}
const user = {
name: "John Doe",
id: 1
};
In the TypeScript example, you can see type annotations and an interface defining the structure of a User
object, which provides compile-time error checking.
In contrast, the JavaScript example lacks these features, making it more flexible but also more prone to runtime errors due to the dynamic nature of the types.
Advantages of Typescript over JavaScript
Here’s a table highlighting the advantages of TypeScript over JavaScript, along with code samples to illustrate these points:
Advantage | TypeScript | JavaScript |
---|---|---|
Static Typing | Offers static typing which helps in catching errors at compile time, leading to fewer runtime errors. | JavaScript is dynamically typed, which can lead to more runtime errors as type errors are only caught during execution. |
Better Tooling | Enhanced tooling with autocompletion, interface checking, and more due to static types. | Tooling is less advanced due to dynamic typing, resulting in less accurate autocompletion and interface checking. |
Advanced Features | Supports advanced features like generics, enums, and decorators, enhancing code quality and maintainability. | Lacks support for these advanced features natively. |
Improved Readability | Code is more readable and easier to understand, especially in large codebases, due to explicit types and interfaces. | Code can become harder to read and maintain, especially in large projects, due to lack of explicit types. |
Easier Refactoring | Safe and easy refactoring, as changes in types are detected across the entire codebase. | Refactoring can be error-prone as changes might not be consistently reflected across the codebase. |
Community and Ecosystem | Growing community focused on robust, large-scale application development. | A vast and diverse community, but with a broader focus not solely on application robustness. |
TypeScript Code Sample:
interface User {
name: string;
age: number;
}
function getUserDetails(user: User): string {
return `${user.name} is ${user.age} years old.`;
}
const user = {
name: "Alice",
age: 30
};
console.log(getUserDetails(user));
JavaScript Code Sample:
function getUserDetails(user) {
return `${user.name} is ${user.age} years old.`;
}
const user = {
name: "Alice",
age: 30
};
console.log(getUserDetails(user));
In the TypeScript example, the User
interface clearly specifies the structure of the user object, enabling the TypeScript compiler to validate types and catch errors.
In contrast, the JavaScript example offers more flexibility but lacks the compile-time checks that TypeScript provides, potentially leading to runtime errors if the object structure is inconsistent.
Advantages of JavaScript
Here’s a table highlighting the advantages of JavaScript over TypeScript, along with code samples to illustrate these points:
Advantage | JavaScript | TypeScript |
---|---|---|
Simplicity | JavaScript is simpler to set up and use, especially for smaller projects or beginners. | TypeScript requires understanding of types and additional syntax, which can be a barrier for beginners. |
Flexibility | Dynamic typing allows for more flexibility in coding and can be faster for prototyping. | Static typing, while reducing errors, can be seen as restrictive and verbose for simple tasks. |
Execution | Can be executed directly in browsers and Node.js without any compilation step. | Needs to be compiled into JavaScript before it can be executed, adding an extra step in the development process. |
Universality | JavaScript is universally understood by web browsers, making it a standard for web development. | TypeScript needs to be transpiled to JavaScript to run in browsers, adding a layer of complexity. |
Learning Curve | Easier learning curve due to its widespread use and abundance of resources and community support. | Has a steeper learning curve due to advanced concepts like types, interfaces, and decorators. |
Third-Party Libraries | Direct and straightforward integration with any JavaScript library without type definition concerns. | May require additional type definitions for seamless integration with third-party JavaScript libraries. |
JavaScript Code Sample:
function calculateArea(radius) {
return Math.PI * radius * radius;
}
console.log(calculateArea(5));
TypeScript Code Sample:
function calculateArea(radius: number): number {
return Math.PI * radius * radius;
}
console.log(calculateArea(5));
In the JavaScript example, the calculateArea
function is straightforward and flexible, allowing any type of argument to be passed. This can be beneficial for quick prototyping and smaller projects.
In contrast, the TypeScript example requires explicit type annotations, which adds robustness but also complexity. For larger projects, TypeScript’s strict typing is advantageous, but for small-scale or rapid development projects, JavaScript’s flexibility can be more practical.
Use Cases for TypeScript
Here’s a table outlining three specific use cases for TypeScript, highlighting where it excels:
Use Case | Description |
---|---|
Large-Scale Application Development | TypeScript’s static typing and advanced features like interfaces and generics make it ideal for developing and maintaining large-scale applications, improving readability and reducing bugs. |
Enterprise-Level Projects | For projects that require long-term maintainability, TypeScript offers robust tooling and early error detection, making it suitable for enterprise-level applications. |
Angular Development | TypeScript is the primary language for Angular, a popular front-end framework. Its features perfectly align with Angular’s architecture, making it ideal for robust and scalable web applications. |
TypeScript Code Samples for Each Use Case:
Large-Scale Application Development:
interface Employee {
id: number;
name: string;
department: string;
}
function getEmployeeDetails(employee: Employee): string {
return `ID: ${employee.id}, Name: ${employee.name}, Department: ${employee.department}`;
}
const emp = { id: 101, name: "John Doe", department: "Engineering" };
console.log(getEmployeeDetails(emp));
Enterprise-Level Projects:
class Product {
constructor(public id: number, public name: string, private price: number) {}
displayProductInfo(): string {
return `Product ID: ${this.id}, Name: ${this.name}, Price: ${this.price}`;
}
}
let product = new Product(123, "Widget", 29.99);
console.log(product.displayProductInfo());
Angular Development:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `<h1>{{ title }}</h1>`
})
export class AppComponent {
title = 'Angular TypeScript App';
}
In each of these examples, TypeScript’s capabilities like interfaces, classes, and decorators are used to illustrate its suitability for large-scale, enterprise-level, and Angular-based applications.
The strong typing system, coupled with OOP features, enhances the structure and maintainability of the code, which are key aspects in these scenarios.
Use Cases for JavaScript
Here’s a table outlining three specific use cases for JavaScript, highlighting where it excels:
Use Case | Description |
---|---|
Web Page Interactivity | JavaScript is extensively used to make web pages interactive, handling user inputs, manipulating DOM, and creating dynamic content changes. |
Server-Side Development | With Node.js, JavaScript can be used for server-side programming, allowing the same language to be used across the full stack of web development. |
Single Page Applications (SPAs) | JavaScript frameworks like React, Vue, and Angular enable the creation of efficient and interactive SPAs, enhancing user experience with smooth, dynamic content loading. |
JavaScript Code Samples for Each Use Case:
Web Page Interactivity:document.getElementById("demo").addEventListener
("click", function() {
alert("Hello World!");
});
Server-Side Development:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello from Node.js');
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
Single Page Applications (SPAs):
// Using React
import React from 'react';
import ReactDOM from 'react-dom';
function App() {
return <h1>Hello, React!</h1>;
}
ReactDOM.render(<App />, document.getElementById('root'));
In each of these examples, JavaScript’s capabilities are leveraged to demonstrate its versatility. The first example shows how JavaScript can be used to add interactivity to web pages. The second example illustrates server-side programming with Node.js.
The third example demonstrates how JavaScript, with the React framework, can be used to create dynamic and responsive single-page applications. These use cases underscore JavaScript’s role as a cornerstone of modern web development.
Which Language to Choose?
Here’s a table to help decide which language to choose, TypeScript or JavaScript, based on different criteria:
Criteria | TypeScript | JavaScript |
---|---|---|
Type Safety | Excellent for projects where type safety is a priority. Static typing helps catch errors early. | Better for projects where rapid prototyping and flexibility are more important than type safety. |
Project Scale | Ideal for large-scale applications due to better structuring and maintainability. | Suited for small to medium projects where the overhead of types may not be necessary. |
Learning Curve | Steeper learning curve due to advanced type system and additional syntax. | Easier to start with, especially for those new to programming. |
Development Speed | Slower initial development due to type definitions, but pays off in large projects with easier maintenance. | Faster initial development, beneficial for quick turnaround projects. |
Ecosystem and Community | Growing community, especially among enterprise-level applications. Strong integration with Angular. | Vast and well-established community. Universal language of the web with numerous resources and libraries. |
Runtime Environment | Compiled to JavaScript for execution. Requires a build process. | Directly executed in the browser or in Node.js, no compilation needed. |
TypeScript Code Sample:
function greet(person: { name: string; age: number }): string {
return `Hello ${person.name}, you are ${person.age} years old.`;
}
console.log(greet({ name: "Alice", age: 30 }));
JavaScript Code Sample:
function greet(person) {
return `Hello ${person.name}, you are ${person.age} years old.`;
}
console.log(greet({ name: "Alice", age: 30 }));
In deciding between TypeScript and JavaScript, consider the scale and complexity of your project, your team’s familiarity with types, and the development environment. TypeScript is more suitable for large, complex projects requiring robust type safety and maintainability, whereas JavaScript is ideal for smaller projects or those requiring quick development and flexibility.
Final Thoughts
Both TypeScript and JavaScript have their unique strengths and are suited to different scenarios:
TypeScript
Is ideal for large-scale or complex applications, particularly where type safety and code maintainability are crucial. Its static typing, advanced features like interfaces and generics, and compatibility with Angular make it a strong choice for enterprise-level projects and robust web applications.
However, it does have a steeper learning curve and requires a compilation step, which might be considered overhead in smaller or rapid development projects.
JavaScript
Shines in its simplicity, flexibility, and universality. It’s a perfect fit for small to medium-sized projects, rapid prototyping, and situations where the dynamic nature of the language can be an advantage.
Its ease of use, widespread support, and direct execution in browsers and Node.js environments make it highly accessible and versatile.
The choice between TypeScript and JavaScript largely depends on project requirements, team expertise, and long-term maintenance considerations.
TypeScript brings in an additional layer of structure and safety at the cost of some complexity, while JavaScript offers greater flexibility and ease of use, especially beneficial for quick development cycles.
External Resources
https://en.wikipedia.org/wiki/JavaScript
https://www.typescriptlang.org/
FAQ
Here are five frequently asked questions about TypeScript vs JavaScript, each with a relevant code sample to illustrate the point:
What are the differences in type checking between TypeScript and JavaScript?
FAQ Answer: TypeScript uses static type checking, where types are checked during compile time, preventing many runtime errors. JavaScript, on the other hand, uses dynamic typing, which means types are checked at runtime.
TypeScript Code Sample:
let age: number = 25; // TypeScript will throw an error if a
non-number is assigned later.
age = "thirty"; // Error: Type 'string' is not assignable to type 'number'.
JavaScript Code Sample:
let age = 25; // JavaScript allows changing the type of a variable at any time.
age = "thirty"; // No error, dynamic typing.
How do interfaces work in TypeScript compared to JavaScript?
FAQ Answer: TypeScript supports interfaces, allowing you to define contracts within your code. JavaScript does not have a native concept of interfaces.
TypeScript Code Sample:
interface User {
name: string;
id: number;
}
const user: User = { name: "John Doe", id: 101 };
Can TypeScript code be used in a JavaScript project?
FAQ Answer: TypeScript compiles down to JavaScript, so the compiled code can be used in JavaScript projects.
However, TypeScript’s specific features like static typing won’t be directly usable in plain JavaScript.
TypeScript to JavaScript Compilation Sample:
TypeScript:
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
}
Compiled JavaScript:
class Greeter {
constructor(message) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
}
What are generics in TypeScript and does JavaScript have an equivalent?
FAQ Answer: Generics in TypeScript allow you to write reusable, generalized code that works with different types. JavaScript does not have an equivalent feature; it relies on its dynamic nature to achieve similar functionality.
TypeScript Code Sample:
function identity<T>(arg: T): T {
return arg;
}
let output = identity<string>("myString");
How do error handling and debugging differ in TypeScript vs JavaScript?
FAQ Answer: TypeScript’s compile-time error checking can catch type errors and issues before the code is executed, leading to potentially fewer runtime errors. JavaScript’s dynamic typing means most errors are only caught when the code is run, which can make debugging more challenging.
TypeScript Code Sample:
function addNumbers(a: number, b: number): number {
return a + b;
}
addNumbers(5, "10"); // Error at compile time
JavaScript Code Sample:
function addNumbers(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new Error("Invalid arguments: expected numbers.");
}
return a + b;
}
addNumbers(5, "10"); // Runtime error caught by manual check
These FAQs provide insights into the functional differences and use cases of TypeScript and JavaScript, highlighting how TypeScript’s static typing and advanced features compare against JavaScript’s dynamic nature and flexibility.
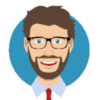
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.