Talk to a data analyst and they’ll likely roll their eyes when you mention JavaScript as a tool for data analysis.
The problem? JavaScript wasn’t originally designed with data analysis in mind, and traditionalists argue there are other, more suited languages for this task.
But after years of wrangling data, I’ve found JavaScript can be a surprisingly adept tool for data analysis with the right approach. Let’s dive into:
Understanding JavaScript Data Analysis
JavaScript has emerged as a powerful language for data analysis, offering a range of capabilities for processing and manipulating data. With its popularity among web developers and the availability of numerous libraries and frameworks, JavaScript has become a go-to language for working with data in businesses of all sizes.
When analyzing data using JS, it is essential to have a firm grasp of the language’s fundamentals. This includes working with variables, loops, functions, and objects, which form the building blocks of data analysis in JavaScript.
For instance, to analyze data, you must be able to access and manipulate arrays, objects, and strings. Here’s an example of a simple code snippet that loops through an array and sums its values:
//array of numbers
let numbers = [5, 8, 12, 16];
//sum variable initialized to zero
let sum = 0;
//loop through array and add each number to sum
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
//print the sum to console
console.log(sum);
Another critical aspect of JavaScript data analysis is data visualization. JS offers various libraries for creating interactive and informative visualizations, such as D3.js and Highcharts. These libraries help users to generate engaging graphics from datasets and convey insights quickly.
Popular JavaScript Data Analysis Frameworks
JavaScript has several popular frameworks and libraries for data analysis, each with unique features and functionalities. Let’s compare and contrast some of the most popular frameworks:
Framework | Description | Code Example |
---|---|---|
D3.js | D3.js is a powerful JavaScript library for data visualization. It allows developers to create highly customized and interactive data visualizations using SVG and HTML. D3.js is widely used in data journalism and business intelligence applications. | //create SVG element var svg = d3.select(“body”) .append(“svg”) .attr(“width”, width + margin.left + margin.right) .attr(“height”, height + margin.top + margin.bottom) .append(“g”) .attr(“transform”, “translate(” + margin.left + “,” + margin.top + “)”); |
Chart.js | Chart.js is a simple and flexible JavaScript charting library. It supports a wide range of chart types, including line, bar, pie, and radar charts. Chart.js is great for creating quick and attractive data visualizations for web applications. | //create a bar chart var myChart = new Chart(ctx, { type: ‘bar’, data: { labels: [‘Red’, ‘Blue’, ‘Yellow’, ‘Green’, ‘Purple’, ‘Orange’], datasets: [{ label: ‘# of Votes’, data: [12, 19, 3, 5, 2, 3], backgroundColor: [ ‘rgba(255, 99, 132, 0.2)’, ‘rgba(54, 162, 235, 0.2)’, ‘rgba(255, 206, 86, 0.2)’, ‘rgba(75, 192, 192, 0.2)’, ‘rgba(153, 102, 255, 0.2)’, ‘rgba(255, 159, 64, 0.2)’ ], borderColor: [ ‘rgba(255, 99, 132, 1)’, ‘rgba(54, 162, 235, 1)’, ‘rgba(255, 206, 86, 1)’, ‘rgba(75, 192, 192, 1)’, ‘rgba(153, 102, 255, 1)’, ‘rgba(255, 159, 64, 1)’ ], borderWidth: 1 }] }, options: { scales: { yAxes: [{ ticks: { beginAtZero: true } }] } } }); |
Both D3.js and Chart.js are great frameworks for data analysis, but they have different strengths and use cases. D3.js is more powerful and flexible, while Chart.js is simpler and more user-friendly.
In addition to these frameworks, there are many other JavaScript libraries for data analysis, such as Highcharts, Google Charts, and FusionCharts. It is important to choose the right framework for your specific project based on its requirements and your expertise.
Data Processing Techniques in JavaScript
The foundation of effective data analysis in JavaScript lies in the ability to control and manipulate data through various data processing techniques. JavaScript provides a wide range of techniques to clean, filter, aggregate, and transform data.
These techniques can be implemented through built-in functions or third-party libraries such as Lodash or Underscore.js.
One common data processing technique is data cleaning, which involves removing or correcting inaccurate, duplicated, or incomplete data. JavaScript provides methods such as trim() and replace() to clean strings and regular expressions to remove or replace specific characters or patterns.
Another technique is data filtering, which allows you to extract specific subsets of data based on certain criteria. JavaScript provides methods such as filter() and find() to accomplish this.
These methods can be used to retrieve data that meets specific conditions, such as values that are greater than a certain threshold or that match a certain pattern.
Data | Filtered Data |
---|---|
15 | 25 |
25 | 30 |
10 | 40 |
40 | 45 |
Aggregation is another technique used to group and summarize data. JavaScript provides methods such as reduce() and groupBy() to perform aggregation operations. These methods allow you to calculate key metrics, such as averages or sums, for groups of data based on a specific attribute.
Name | Age |
---|---|
John | 25 |
Mary | 30 |
John | 35 |
Alex | 40 |
Transforming data is yet another technique that allows you to modify or reshape data in order to prepare it for analysis or visualization. JavaScript provides methods such as map() and flatten() to achieve this.
These methods are used to restructure data into a format that is more suitable for further processing or analysis.
By mastering these data processing techniques, you can extract valuable insights from your data and make informed business decisions.
Visualizing Data with JavaScript
Effective data analysis is not complete without proper data visualization. Visualization is important for gaining insights and identifying patterns in data. In JavaScript, there are various libraries and tools available for creating interactive and informative visualizations. Let’s explore some of the popular ones:
D3.js
D3.js is a powerful JavaScript library for data visualization. Its flexibility and versatility make it ideal for complex, custom visualizations. Below is an example of a D3.js scatterplot created by binding data to a set of SVG circles:
var svg = d3.select("body")
.append("svg")
.attr("width", 500)
.attr("height", 500);
var data = [
{ x: 10, y: 20 },
{ x: 30, y: 40 },
{ x: 50, y: 60 }
];
svg.selectAll("circle")
.data(data)
.enter()
.append("circle")
.attr("cx", function(d) { return d.x; })
.attr("cy", function(d) { return d.y; })
.attr("r", 10);
The code above creates an SVG canvas and binds data to a set of circles using D3.js. The “cx” and “cy” attributes define the circle’s position based on the data, while the “r” attribute sets its radius.
Highcharts
Highcharts is another popular JavaScript library for data visualization. It offers a wide range of chart types, including bar, line, area, and pie charts. Below is an example of a Highcharts bar chart created by defining data and chart options:
Highcharts.chart('container', {
chart: { type: 'bar' },
title: { text: 'Fruit Consumption' },
xAxis: { categories: ['Apples', 'Bananas', 'Oranges'] },
yAxis: { title: { text: 'Fruit eaten' } },
series: [{
name: 'Jane',
data: [1, 0, 4]
}, {
name: 'John',
data: [5, 7, 3]
}]
});
The code above creates a Highcharts bar chart by defining chart options and series data. The “xAxis” and “yAxis” properties set the chart axes, while the “series” property defines the chart data.
JavaScript data visualization libraries like D3.js and Highcharts offer a wide range of options for creating interactive and informative visualizations. Choose the one that best suits your needs and integrates well with your data and application.
Javascript Data Analysis Best Practices
As with any programming task, there are certain best practices that should be followed when performing data analysis using JavaScript. These practices can help ensure that your analysis is efficient, accurate, and secure.
1. Ensure Data Quality: Before conducting any analysis, it is important to ensure that your data is of high quality. Clean, consistent data is vital for accurate analysis results. Be sure to handle missing or corrupted data appropriately, and validate your data before analyzing it.
2. Prioritize Data Security: When handling sensitive data, security should always be a top priority. Use encryption and other security measures to protect your data from unauthorized access or theft. Be sure to also follow industry-standard security protocols when storing data.
3. Optimize for Performance: Large datasets can be slow to analyze, so optimizing your code for performance is important. Use efficient data structures and algorithms, and consider using frameworks or libraries that are optimized for performance.
4. Use Libraries and Frameworks: There are many libraries and frameworks available for data analysis in JavaScript. Using these tools can save time and effort, and can offer additional features and functionality that may not be available in vanilla JavaScript.
5. Document Your Code: As with any programming project, it is important to document your code. This can help you and others understand how your code works, and can make it easier to troubleshoot issues or make updates in the future.
6. Test Your Code: Before deploying your analysis, be sure to thoroughly test your code. This can help catch any errors or bugs that may impact your results.
By following these best practices, you can ensure that your JavaScript data analysis is accurate, secure, and efficient. Keep in mind that these practices may vary depending on the specific project or industry, so it is important to stay up-to-date on industry standards and trends.
JavaScript vs Other Languages for Data Analysis
JavaScript has emerged as a popular language for data analysis in recent years, but how does it compare to other programming languages commonly used for this purpose?
Python and R have traditionally been the go-to languages for data analysis due to their powerful libraries and extensive toolkits. However, JavaScript’s flexibility and versatility have made it a strong contender in the field.
While Python and R may have deeper and more diverse libraries of data analysis tools, JavaScript provides a robust set of tools for data visualization and manipulation. With the rise of web applications and online data storage, JavaScript’s compatibility with these technologies provides an advantage over languages like Python or R.
Language | Strengths | Weaknesses |
---|---|---|
JavaScript | Flexibility, versatility, compatibility with web technologies | Less diverse collection of data analysis libraries |
Python | Extensive libraries for data analysis, diverse toolkit | Not as compatible with web technologies as JavaScript |
R | Powerful statistical analysis capabilities, extensive data visualization libraries | Not as versatile or flexible as JavaScript |
For instance, while Python provides Pandas for data manipulation, JavaScript provides D3.js for data visualization. Both libraries are powerful and popular, but each language has a different emphasis on what types of tools should be made available. Similarly, JavaScript’s user-friendly syntax is easier to learn for people with a background in web development, while Python’s syntax may be challenging for them.
JavaScript also has the advantage of a large and active community of developers constantly creating and improving frameworks and libraries for data analysis. This allows for a more dynamic and evolving ecosystem that can adapt to changing industry needs and emerging trends.
Overall, the choice of language for data analysis depends on the specific needs and requirements of the project. JavaScript’s compatibility with web technologies and its growing set of data analysis tools make it a strong contender, but Python and R have deeply entrenched and more diverse libraries for data analysis. Nonetheless, JavaScript’s ease-of-use and active community of developers make it a language to watch in the field of data analysis.
Advanced JavaScript Data Analysis Techniques
JavaScript has evolved into a powerful language for data analysis, and advanced techniques can be implemented to derive insights from large and complex datasets. Here are some of the advanced data analysis techniques that can be performed using JavaScript:
Machine Learning
Machine learning is a popular technique for data analysis, and JavaScript provides various libraries and tools for implementing machine learning algorithms. TensorFlow.js is one such library that enables developers to create and train machine learning models using JavaScript. With TensorFlow.js, developers can perform various tasks such as image recognition, natural language processing, and predictive modeling.
Here’s an example of TensorFlow.js code for predicting the price of a house based on its features:
// Define the model
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Compile the model
model.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
// Train the model
model.fit(xs, ys, {epochs: 10}).then(() => {
// Predict the price of a house
const prediction = model.predict(tf.tensor2d([7], [1, 1]));
prediction.print();
});
Natural Language Processing
Natural language processing (NLP) is another area where JavaScript can be used for data analysis. NLP involves analyzing and understanding human language, and JavaScript provides various libraries for performing NLP tasks such as sentiment analysis, text classification, and language translation. Natural provides an easy-to-use API for performing NLP tasks using JavaScript.
Here’s an example of Natural code for sentiment analysis:
const natural = require('natural');
const classifier = new natural.BayesClassifier();
// Train the classifier
classifier.addDocument('I love this product', 'positive');
classifier.addDocument('This product is terrible', 'negative');
classifier.train();
// Classify new text
console.log(classifier.classify('I hate this product'));
// Output: 'negative'
Big Data Analysis
JavaScript can also be used for analyzing big data, which involves processing and analyzing large datasets that cannot be handled by traditional data analysis tools. Apache Spark is a popular big data processing engine that provides a JavaScript API for processing and analyzing large datasets.
With Apache Spark, developers can perform various big data analysis tasks such as data cleansing, data aggregation, and data modeling.
Here’s an example of Apache Spark code for counting the number of words in a large dataset:
// Create a SparkContext
const SparkContext = require('eclairjs/SparkContext');
const sc = new SparkContext();
// Load the dataset
const textFile = sc.textFile('hdfs://...');
// Count the number of words
const words = textFile.flatMap(line => line.split(' '));
const wordCounts = words.countByValue();
// Output the results
console.log(wordCounts);
JavaScript provides various capabilities for advanced data analysis techniques such as machine learning, natural language processing, and big data analysis. With JavaScript, developers can perform complex data analysis tasks efficiently and effectively.
JavaScript Data Analysis Examples
By examining these examples, we can gain insights into the different techniques and frameworks used to tackle various data analysis challenges.
Example 1: Company A
Company A, a leading e-commerce platform, used JavaScript for data analysis to optimize their product recommendations algorithm. The company collected massive amounts of data on customer purchases and browsing behavior. However, they faced challenges in processing and interpreting this data efficiently.
Using D3.js, they were able to create interactive visualizations that helped them identify which products were most frequently viewed together and which products were frequently purchased together. By analyzing these patterns, they were able to improve their recommendation algorithm and boost customer engagement.
The flexibility and scalability of JavaScript allowed Company A to handle large datasets and create custom visualizations that fit their specific data analysis needs.
Example 2: Company B
Company B, a social media platform, leveraged JavaScript for data analysis to improve user engagement and retention. The company realized that they needed a better understanding of the content that users engaged with the most.
Using Chart.js, Company B was able to create a dashboard that displayed the most popular types of content (text, images, videos, etc.) and the engagement metrics for each type. This helped them identify which types of content were most successful in keeping users engaged on the platform.
The interactive nature of the dashboard allowed Company B to quickly iterate and test different content strategies based on the data they collected.
Example 3: Company C
Company C, a healthcare provider, utilized JavaScript for data analysis to improve patient outcomes. They collected data on patient medical history, symptoms, and treatments and needed to extract insights to support clinical decision-making.
Using JavaScript for data aggregation and transformation, Company C was able to create predictive models that helped identify patients at risk of certain conditions. By analyzing this data, they were able to develop targeted treatment plans and improve overall patient outcomes.
The versatility of JavaScript allowed Company C to integrate their data analysis into their existing healthcare systems and workflows, making it easier for medical professionals to access and utilize the insights gained from data analysis.
These examples demonstrate the potential of JavaScript for data analysis across various industries and use cases. By leveraging the power and versatility of JavaScript, these companies were able to gain valuable insights that helped them optimize their operations and improve outcomes.
Final thoughts
JavaScript is a versatile and powerful language that has become increasingly popular for data analysis in recent years. From data manipulation and processing to visualization and advanced techniques such as machine learning, JavaScript offers a wide range of tools and frameworks that can help businesses make informed decisions and gain a competitive edge.
External Resources
https://en.wikipedia.org/wiki/JavaScript
https://developer.mozilla.org/en-US/docs/Web/JavaScript
FAQ
Data analysis in JavaScript involves using JavaScript and its libraries to manipulate, analyze, and visualize data.
Here are five frequently asked questions about data analysis in JavaScript, each with a brief explanation and a code sample:
1. How do I read and parse a CSV file in JavaScript?
Reading and parsing CSV files in JavaScript can be done using the Papa Parse
library, which is a powerful and easy-to-use CSV parser.
// Using Papa Parse to read a CSV file
Papa.parse("http://example.com/file.csv", {
download: true,
complete: function(results) {
console.log(results);
}
});
2. How can I perform data manipulation and analysis in JavaScript?
For data manipulation and analysis, the Lodash
library provides many utility functions that are very handy.
// Using Lodash to manipulate data
const _ = require('lodash');
let users = [
{ 'user': 'fred', 'age': 48 },
{ 'user': 'barney', 'age': 36 },
{ 'user': 'fred', 'age': 40 },
{ 'user': 'barney', 'age': 34 }
];
let youngest = _.chain(users)
.sortBy('age')
.map(function(o) {
return o.user + ' is ' + o.age;
})
.head()
.value();
console.log(youngest); // 'barney is 34'
3. How do I create charts and visualizations in JavaScript?
To create charts and visualizations, the Chart.js
library is a popular choice. It’s simple and flexible.
// Using Chart.js to create a bar chart
var ctx = document.getElementById('myChart').getContext('2d');
var myChart = new Chart(ctx, {
type: 'bar',
data: {
labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'],
datasets: [{
label: '# of Votes',
data: [12, 19, 3, 5, 2, 3],
backgroundColor: [
'rgba(255, 99, 132, 0.2)',
'rgba(54, 162, 235, 0.2)',
'rgba(255, 206, 86, 0.2)',
'rgba(75, 192, 192, 0.2)',
'rgba(153, 102, 255, 0.2)',
'rgba(255, 159, 64, 0.2)'
],
borderColor: [
'rgba(255, 99, 132, 1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(75, 192, 192, 1)',
'rgba(153, 102, 255, 1)',
'rgba(255, 159, 64, 1)'
],
borderWidth: 1
}]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
});
4. How can I handle large datasets efficiently in JavaScript?
Handling large datasets efficiently in JavaScript can be achieved using d3.js
for data-driven manipulations and crossfilter.js
for fast filtering and grouping of multi-dimensional data.
// Example using Crossfilter.js
var ndx = crossfilter(data); // 'data' is your dataset
var dim = ndx.dimension(d => d.date);
var group = dim.group().reduceSum(d => d.value);
// Now you can perform various operations on this dimension and group
5. How do I perform statistical analysis in JavaScript?
For statistical analysis, you can use the Simple Statistics
library, which provides basic statistical functions.
// Using Simple Statistics for statistical analysis
const ss = require('simple-statistics');
let numbers = [1, 2, 3, 4, 5, 6];
let mean = ss.mean(numbers);
let variance = ss.variance(numbers);
console.log(`Mean: ${mean}, Variance: ${variance}`);
Remember to include the respective libraries in your project to use these code samples effectively.
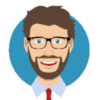
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.