Developing a website or web application requires selecting the right frontend framework to get the desired User Interface (UI) for your project. Two of the most popular options are Svelte and React.js, both of which have garnered significant attention and praise in the industry.
Comparing Svelte and React.js can help in making an informed decision when it comes to selecting the right frontend framework for your project.
Understanding Svelte and React.js
Frontend frameworks play a critical role in modern web development, and two of the most popular options are Svelte and React.js. Both frameworks are based on component-based architecture and offer features such as virtual DOM and reactive programming. Let’s take a closer look at each one:
Svelte
Svelte is a relatively new framework, launched in 2016, and has gained popularity due to its simplicity and performance. One of the key differences between Svelte and React.js is that Svelte compiles code at build time, while React compiles at runtime.
Here’s an example of a Svelte component:
// Counter.svelte
<script>
let count = 0;
</script>
<button on:click="{() => {count += 1;}}">
Clicked {count} times
</button>
This Svelte component creates a counter that increases by one each time it is clicked. As you can see, Svelte uses reactive statements to update the component.
React.js
React.js is a popular frontend framework based on JavaScript. It was developed by Facebook and is widely used in the industry. React uses a virtual DOM to improve performance and offers an extensive ecosystem of libraries and tools.
Here’s an example of a React component:
// Counter.js
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<button onClick={() => setCount(count + 1)}>
Clicked {count} times
</button>
);
}
export default Counter;
This React component also creates a counter that updates its value each time it is clicked. In React, we use the useState hook to manage state.
Both Svelte and React.js offer different approaches to web development, and the choice ultimately depends on specific project requirements. However, understanding the core programming concepts and features of each framework can help developers make informed decisions.
Performance Comparison
When choosing a frontend framework for web development projects, performance is a critical factor to consider.
Svelte’s approach to performance is unique, as it compiles components at build time rather than runtime. This means that Svelte generates highly optimized, simple JavaScript code that runs faster and with fewer resources. In comparison, React.js uses a virtual DOM that creates a JavaScript object representation of the actual DOM and updates it during run time. This approach, while flexible and powerful, can result in slower performance and larger bundle sizes.
To illustrate the performance differences between the two frameworks, let’s compare the rendering speed of a simple button component in Svelte and React.js:
Svelte Example
//Button.svelte
<script>
export let label;
</script>
<button>{label}</button>
The above example shows a Svelte button component that takes a label prop and renders a button element with the label text. When compiled, Svelte generates optimized code that results in faster rendering speed and smaller bundle size.
React.js Example
//Button.js
import React from 'react';
function Button(props) {
return <button>{props.label}</button>;
}
export default Button;
The above example shows a React.js button component that takes a label prop and renders a button element with the label text. Because React.js uses a virtual DOM and updates it during runtime, the resulting code is larger and slower than Svelte.
In addition to rendering speed, Svelte’s approach to reactive programming and optimized code also results in better programming efficiency. As the codebase grows, Svelte’s compiler generates highly optimized code that allows for faster development and easier maintenance than React.js.
Overall, while React.js is a powerful and flexible framework, Svelte’s approach to performance and programming efficiency make it a strong contender for frontend web development projects.
Developer Experience and Learning Curve
When it comes to Svelte versus React.js, developer experience and learning curve are important factors to consider. Both frameworks require knowledge of frontend programming, including HTML, CSS, and JavaScript. However, the overall approach to building user interfaces differs between the two frameworks.
Svelte has a reputation for being easy to learn, thanks to its simple syntax and intuitive component-based architecture.
In fact, the official Svelte website claims that developers can “build a complete app in less than an hour” using the framework. Svelte also provides excellent documentation, a supportive community, and a growing number of learning resources, including video tutorials and online courses.
Here is an example of Svelte’s syntax for creating a component:
// Button.svelte
<script>
export let text;
</script>
<button>{text}</button>
On the other hand, React.js has a steeper learning curve due to its use of JSX and virtual DOM. However, React.js also has a large and active community, extensive documentation, and many learning resources available, including free and paid courses.
Here is an example of React.js syntax for creating a component:
// Button.js
import React from 'react';
function Button(props) {
return <button>{props.text}</button>;
}
export default Button;
Ultimately, the framework you choose will depend on your level of experience and coding preferences. Svelte may be a more accessible option for beginners, while React.js may be a better fit for more experienced developers or those who prefer its JSX syntax.
Ecosystem and Tooling
When it comes to choosing a frontend framework, developers often consider the availability of libraries, frameworks, and community-driven resources.
Svelte Ecosystem
Svelte is a relatively new frontend framework, but it has quickly gained popularity among developers. The framework has a small but growing ecosystem of plugins, components, and tools.
The official Svelte website provides documentation, tutorials, and examples to help developers get started with the framework.
One of the advantages of Svelte is that it doesn’t require a big ecosystem of supporting libraries because it’s so flexible. Svelte is designed to allow users to write code in vanilla JavaScript, which makes it easy to integrate with other libraries, tools, and frameworks.
For example, Svelte can be easily used with Redux, GraphQL, and TypeScript, among others.
React.js Ecosystem
React.js has a large and well-established ecosystem of libraries, components, and tools. React.js is often the go-to choice for frontend developers because of the vast number of libraries and resources available. Popular React.js libraries include Redux for state management, React Router for routing, and Material UI for UI components.
React.js also has great community support with a wide range of blogs, tutorials, and videos that provide guidance for both beginners and experienced developers. The official React.js website provides documentation and examples that make it easy to get started with the framework.
Frontend Development Tools
Both Svelte and React.js have a range of tools available for frontend development. Svelte has a built-in compiler that can optimize and compress code for efficient rendering, making it a great choice for high-performance applications. Svelte also has an official extension for Visual Studio Code, providing a seamless development experience.
React.js, on the other hand, has a range of tools available for testing, debugging, and building applications. Popular tools for React.js development include Babel for transpiling, Jest for testing, and Webpack for bundling.
Framework | Ecosystem | Tools |
---|---|---|
Svelte | Small but growing | Built-in compiler, official VS Code extension |
React.js | Large and established | Babel, Jest, Webpack |
Overall, both Svelte and React.js have strong ecosystems and tools available for frontend development. The choice between the two will depend on specific project requirements, developer preferences, and the availability of resources in the development community.
State Management: Comparing Svelte and React.js
State management is a critical aspect of developing frontend applications, and both Svelte and React.js provide solutions for managing application state. In Svelte, state management revolves around the use of reactive variables, while React.js makes use of both props and state. Let’s explore the state management options in both frameworks:
Svelte
In Svelte, reactive variables are used to manage changes in the application state. These variables are automatically updated when their underlying data changes, and any components that rely on them are also updated accordingly.
Here’s an example of a reactive variable:
<script>
let count = 0;
$: double = count * 2; // reactive variable
function handleClick() {
count += 1;
}
</script>
<button on:click={handleClick}>Click Me</button>
<p>Count: {count}</p>
<p>Double: {double}</p>
Here, the reactive variable “double” is updated every time the count changes. This ensures that any components that rely on “double” are also updated, resulting in a seamless user experience.
React.js
React.js makes use of both props and state for managing application state. In this framework, props are used for passing data down from parent components to child components, while state is used for managing changes within a component.
Here’s an example of using state in a React.js component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // state
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Click Me</button>
</div>
);
}
export default Counter;
Here, the useState hook is used to manage the state within the Counter component. The count value is initialized to 0, and the setCount function is used to update the value whenever the button is clicked. This ensures that the count value is updated within the component, resulting in a re-render and an updated user interface.
Overall, both Svelte and React.js provide solutions for managing application state. While Svelte relies heavily on reactive variables, React.js makes use of both props and state. Developers should carefully consider their project requirements when selecting a framework for state management.
Component Reusability
One of the key features of modern frontend frameworks is the ability to create reusable components that can be easily shared across multiple parts of a project. Both Svelte and React.js offer robust component architectures that enable efficient development and maintenance of complex frontend applications.
In Svelte, components are defined as standalone files with an HTML layout, JavaScript logic, and CSS styling. These components can be easily imported and used within other Svelte files or projects.
Here’s an example of a reusable Svelte component:
<script> export let title; </script> <h3>{title}</h3>
In React.js, components are defined as classes or functions with JSX code that describes their layout and behavior. These components can be exported and imported into other React.js files or projects.
Here’s an example of a reusable React.js component:
import React from 'react' const MyComponent = ({ title }) => { return
( <h3>{title}</h3> ) } export default MyComponent
Both Svelte and React.js encourage the creation and usage of reusable components as a core element of frontend architecture. The availability of component libraries, such as Material UI for React.js and Svelte Material UI for Svelte, further enhances their reusability and accessibility across projects.
Community and Industry Adoption
When it comes to choosing a frontend framework, community and industry adoption can provide valuable insight into their strengths and weaknesses. The Svelte community is growing steadily, with many developers praising the framework’s simplicity and performance.
While it may not have the same level of adoption as React.js, Svelte is gaining traction and is being implemented in both personal and enterprise projects.
The React.js community, on the other hand, is one of the largest and most active in the web development industry. It has a vast ecosystem of libraries, resources, and tools, making it a go-to choice for many developers.
React.js is also widely used by tech giants such as Facebook, Reddit, and Netflix, demonstrating its suitability for enterprise-level projects.
However, it’s important to note that community size and industry adoption don’t always reflect the quality or suitability of a framework for a specific project. Depending on the project’s needs and requirements, a smaller community or lesser-known framework may be the best choice.
Therefore, it’s crucial to assess the framework’s strengths and weaknesses alongside community and industry adoption when making a decision.
Final Thoughts
When it comes to selecting a frontend framework for your web development projects, there are many factors to consider. In this article, we have compared two popular options in the industry – Svelte and React.js – and highlighted their strengths and weaknesses in various areas.
External Resources
FAQ
Q: Can I use both Svelte and React.js in the same project?
A: Yes, it is possible to use both Svelte and React.js in the same project. However, it may require additional configuration and integration work to ensure smooth interoperability between the two frameworks.
Q: Which framework is better for performance optimization?
A: Both Svelte and React.js have their own performance optimizations. Svelte is known for its compiled output, which allows for smaller bundle sizes and faster rendering. On the other hand, React.js has a virtual DOM that efficiently updates only the necessary components. The choice between the two would depend on the specific requirements of your project.
Q: Are there any differences in the learning curves for Svelte and React.js?
A: The learning curve for Svelte and React.js can vary depending on your previous experience with frontend development. Svelte’s syntax is closer to traditional HTML and JavaScript, making it easier for beginners to grasp. React.js, on the other hand, has a steeper learning curve due to its JSX syntax and the need to understand concepts such as components and JSX transformations.
Q: Which framework has a larger community and industry adoption?
A: React.js has a larger and more mature community compared to Svelte. React.js has been around longer and has gained widespread adoption in the web development industry. However, Svelte is steadily growing in popularity and has a dedicated community of developers who actively contribute to its ecosystem.
Q: How does state management differ in Svelte and React.js?
A: Svelte and React.js have different approaches to state management. React.js uses a centralized state management solution like Redux or the Context API. Svelte, on the other hand, has built-in reactive variables that make state management more intuitive and straightforward.
It eliminates the need for external libraries in many cases.
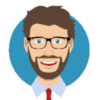
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.