As a developer, you’re always looking for ways to optimize and improve the functionality of your web applications. By leveraging Request.js with Rails, you can make HTTP requests more efficiently and effectively.
Before we dive into the technical aspects of integrating Request.js with Rails, let’s take a moment to understand what Request.js is and why it is beneficial to use it with Rails.
Understanding Request.js
Before we delve into using Request.js with Rails, it’s crucial to have a clear understanding of what Request.js is and what it can do. Request.js is a powerful and lightweight JavaScript library that provides an easy and efficient way to make HTTP requests directly from the client-side JavaScript code.
With Request.js, you can use a single API to make various types of requests like GET, POST, PUT, and DELETE requests with ease. Additionally, it offers numerous features such as interceptors, request and response transformations, and automatic JSON parsing.
One of the main advantages of Request.js is its simplicity: It provides an intuitive interface that requires minimal setup and can be used with any JavaScript framework or library. Moreover, Request.js is compatible with all modern browsers and Node.js, making it an ideal tool for building web applications.
If you’re familiar with jQuery’s AJAX function, Request.js follows a similar syntax, but with additional features and improved performance.
Why Use Request.js with Rails?
Integrating Request.js with Rails can provide several advantages that enhance the functionality and performance of your web applications. Here are a few reasons why:
- Efficient HTTP requests: Request.js simplifies the process of making HTTP requests from client-side JavaScript code. By leveraging Request.js, you can make requests more efficiently and handle responses seamlessly within your Rails application.
- Flexibility: Request.js works with several different data formats, including JSON, XML, and FormData. This flexibility allows developers to handle a range of data formats according to the specific requirements of their application.
- Asynchronous requests: Request.js allows you to make asynchronous requests, which means that your application can continue running while waiting for the response from the server. This capability improves the overall performance of your application.
- Compatibility with other libraries: Request.js integrates smoothly with other popular JavaScript libraries such as Angular, React, and Vue.js. This compatibility allows developers to leverage the power of multiple libraries in their application seamlessly.
By using Request.js with Rails, you can achieve a robust and efficient workflow that streamlines the communication between your client-side and server-side code. In the following section, we will explore the technical aspects of integrating Request.js with Rails.
Integrating Request.js with Rails
Now that we understand the benefits of using Request.js with Rails, let’s dive into the technical aspects of integrating this powerful library into your Rails application. The first step is to install Request.js either via a CDN or by downloading it and adding it to your Rails application’s asset pipeline.
Next, we need to make sure that Request.js is loaded before any of our JavaScript code. You can do this by adding the following code to your application.js file:
//= require request
This ensures that Request.js is loaded and available for use.
Now that we have Request.js loaded, we can start making HTTP requests. To do this, we create a new instance of the Request class and set the necessary options, such as the URL and HTTP method:
// Making a GET request
var request = new Request('/api/data');
// Making a POST request
var request = new Request('/api/data', {
method: 'POST',
body: JSON.stringify({data: 'example'})
});
You can also add additional options such as headers or authentication tokens. Once the options are set, we can use the fetch() method to send the HTTP request:
// Sending the request
fetch(request)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the fetch() method to send the request and then parsing the response as JSON data. You can also handle other types of responses such as text or binary data.
And that’s it! With Request.js properly integrated into your Rails application, you can now make HTTP requests more efficiently and effectively.
Making HTTP Requests in Rails with Request.js
With Request.js properly integrated into your Rails application, you can now start making HTTP requests. The library supports GET, POST, PUT, and DELETE requests.
Here’s an example of making a GET request using Request.js:
Example 1: Making a GET request
Rails Code | Request.js Code |
---|---|
require 'net/http' uri = URI('https://jsonplaceholder.typicode.com/posts/1') response = Net::HTTP.get(uri) puts response | const request = require('request'); request.get('https://jsonplaceholder.typicode.com/posts/1', function (error, response, body) { console.error('error:', error); console.log('statusCode:', response && response.statusCode); console.log('body:', body); }); |
The Rails code above uses the built-in Net::HTTP library to make a GET request to the specified URL and output the response. The Request.js code, on the other hand, uses the request library to achieve the same result.
Here’s an example of making a POST request:
Example 2: Making a POST request
Rails Code | Request.js Code |
---|---|
require 'net/http' require 'json' uri = URI('https://jsonplaceholder.typicode.com/posts') http = Net::HTTP.new(uri.host, uri.port) http.use_ssl = true request = Net::HTTP::Post.new(uri.path, 'Content-Type' => 'application/json') request.body = { title: 'foo', body: 'bar', userId: 1 }.to_json response = http.request(request) puts response.body |
|
Both the Rails and Request.js code above make a POST request to the specified URL with a JSON payload containing a title, body, and userId field.
PUT and DELETE requests can be made in a similar fashion using Request.js. Simply replace request.post with request.put or request.delete, respectively.
Handling Responses in Request.js
When making HTTP requests using Request.js in Rails, it’s crucial to handle the various types of responses that may be returned. Here, we’ll cover some common response handling techniques in Request.js.
Handling Errors
Errors can occur for a variety of reasons, such as network issues or server-side errors. In Request.js, we can use the on("error")
method to handle errors:
Example:
request
.get('/api/data')
.on("error", function(err){
console.log("Error:", err);
});
In this example, we’re making a GET request to the ‘/api/data’ endpoint. If an error occurs, the on("error")
method will be called and the error will be logged to the console.
Handling Status Codes
In addition to handling errors, we may also want to handle specific HTTP status codes that may be returned. For example, we may want to redirect the user if the response status code is 401 (Unauthorized). We can use the on("response")
method to handle response codes:
Example:
request .get('/api/data') .on("response", function(response)
{ if (response.statusCode === 401) { window.location.replace("/login"); } });
In this example, we’re making a GET request to the ‘/api/data’ endpoint. If the response status code is 401, we’ll redirect the user to the login page using the window.location.replace()
method.
Parsing JSON Responses
JSON is a common format for data returned by HTTP requests. In Request.js, we can use the json()
method to parse JSON responses:
Example:
request .get('/api/data') .on("response", function(response)
{ response.json().then(function(data){ console.log(data); }); });
In this example, we’re making a GET request to the ‘/api/data’ endpoint. After the response is received, we’re parsing the JSON data using the json()
method and logging it to the console.
Handling responses in Request.js is an essential part of making HTTP requests in Rails. By properly handling errors, status codes, and parsing JSON responses, we can create robust and reliable applications.
Comparing Request.js with Other HTTP Request Libraries
Request.js is not the only library available for making HTTP requests in web development. In this section, we will compare Request.js with other popular HTTP request libraries to help you determine which one best suits your coding needs.
Axios
Axios is a popular HTTP request library that boasts an easy-to-use API and support for both Node.js and web browsers. Like Request.js, Axios provides methods for making GET, POST, PUT, and DELETE requests and includes features such as cancellation and response interceptors. Axios also automatically converts response data to JSON and has built-in support for handling cross-site scripting (XSS) attacks.
Here’s an example of making a GET request with Axios:
axios.get('/api/users')
.then(response => console.log(response))
.catch(error => console.log(error));
Fetch
Fetch is a newer API for making HTTP requests built into most modern web browsers, although it’s not supported in Internet Explorer. Fetch has a simpler syntax than Axios and Request.js but provides less flexibility in terms of configuration options. Fetch also returns a Promise by default instead of a more traditional callback function, making it well-suited for modern web development with asynchronous JavaScript.
Here’s an example of making a GET request with Fetch:
fetch('/api/users')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
Superagent
Superagent is another popular HTTP request library that offers support for both Node.js and web browsers. Superagent includes features such as file uploads and streaming responses and has a simple syntax for making requests with customizable options. Superagent also includes built-in support for promises and can work with JSON or XML data.
Here’s an example of making a GET request with Superagent:
superagent.get('/api/users')
.query({ page: 2, limit: 10 })
.end((error, response) => console.log(response));
When comparing these libraries, it’s important to consider your specific needs and preferences as a developer. Request.js and Axios offer similar features and syntax but differ in their community support and popularity. Fetch provides a simpler and more modern API but lacks some of the configuration options of other libraries.
Superagent offers unique features but may be less well-known in the development community.
Ultimately, the choice of which HTTP request library to use depends on your project’s requirements, your development team’s familiarity with the library, and your overall coding style and preferences.
Tips and Best Practices
As you integrate Request.js with Rails, there are several tips and best practices that can help optimize your code and enhance your application’s performance.
1. Use Asynchronous Requests
One of the most significant benefits of using Request.js is its ability to make asynchronous requests. By leveraging the async/await syntax in your code, you can avoid blocking the main thread and improve the overall responsiveness of your application. For example:
// Using async/await
async function getUsers() {
const response = await request.get('/users');
return response.data;
}
// Without async/await
function getUsers() {
request.get('/users').then((response) => {
return response.data;
});
}
2. Limit the Number of Requests
Excessive requests can significantly slow down your application and even cause performance issues. To avoid this, it’s essential to limit the number of requests you make, especially when working with large datasets. Use pagination or lazy loading techniques to load data incrementally and avoid overwhelming your application with unnecessary requests.
3. Safeguard Against Security Vulnerabilities
When making requests, it’s crucial to guard against security vulnerabilities such as Cross-Site Request Forgery (CSRF) attacks. Ensure that your Rails application is configured to use CSRF tokens and that Request.js automatically includes these tokens with each request. Use HTTPS to ensure secure communication between the client and server.
4. Debug Your Code
Debugging is an integral part of the development process, and it’s essential to test your code thoroughly when working with a new library like Request.js. Use the browser’s console or a dedicated debugging tool to identify errors and troubleshoot issues with your code. Take advantage of Request.js’s error handling capabilities to identify and address issues with your HTTP requests.
5. Optimize Responses
When making HTTP requests, it’s essential to optimize the responses to ensure that you’re only receiving the necessary data. Use optional query parameters in your requests to filter data and reduce the response payload. You can also use Request.js’s data transformation capabilities to modify response data and extract the required information.
By following these tips and best practices, you can optimize your Request.js code and enhance the performance of your Rails application.
Troubleshooting Common Issues
Despite the benefits of integrating Request.js with Rails, issues may arise during the process. In this section, we will address some common problems that developers face, along with potential solutions and troubleshooting tips.
Problem: CORS
One of the most common issues when using Request.js with Rails is dealing with CORS errors. This occurs when the client-side JavaScript code tries to make requests to a domain different from the domain that served the initial page.
To solve this issue, you need to enable CORS on your Rails server. One way to do this is by adding the ‘rack-cors’ gem to your Gemfile and configuring it in your application.rb file.
Example:
#Gemfile
gem 'rack-cors'
#config/application.rb
config.middleware.insert_before 0, Rack::Cors do
allow do
origins '*'
resource '*', headers: :any, methods: [:get, :post, :options, :delete, :put]
end
end
Problem: Unexpected Results
Sometimes, when making requests with Request.js in Rails, you may receive unexpected results, such as incorrect responses or no responses at all.
One possible cause of this issue is incorrect URL formatting. Make sure you are using the correct URL format in your requests.
Another possible cause is server-side issues. Check your server logs to see if there are any errors or exceptions being thrown.
Problem: Slow Response Times
Slow response times can significantly impact the performance of your web application.
One possible cause of slow response times is server load. Check if your server is experiencing high traffic or if there are any processes running that are consuming a lot of resources.
Another possible cause is the size of your data payloads. If you are sending large amounts of data in your requests, it may take longer to process and return a response. Consider optimizing your data payloads to reduce their size or breaking them up into smaller requests.
Problem: Security
Security is a crucial aspect of any web application, and integrating Request.js with Rails requires some additional security considerations.
One potential security issue is cross-site scripting (XSS) attacks. Make sure that all data sent from the client-side code is properly sanitized to prevent any XSS vulnerabilities.
Another potential security issue is cross-site request forgery (CSRF) attacks. To prevent CSRF attacks, include a CSRF token in all requests sent from client-side code.
By addressing these common issues, you can ensure that your integration of Request.js with Rails is smooth and efficient.
Final Thoughts
Request.js is a powerful and versatile library that can greatly enhance the functionality and performance of your Rails applications. By integrating Request.js with Rails, you can make HTTP requests more efficiently and effectively, improving the user experience and streamlining your development workflow.
With the step-by-step guide provided in this article, you can seamlessly integrate Request.js into your Rails application and start taking advantage of its many features and benefits. Remember to follow best practices for optimizing your code and enhancing security, and refer to the troubleshooting tips if you encounter any issues.
Take inspiration from industry examples and trends to see how Request.js can be applied in real-world scenarios, and explore the provided references and resources to further deepen your knowledge. With Request.js and Rails, the possibilities are endless.
External Resources
https://developer.mozilla.org/en-US/docs/Web/API/Request
FAQ
Q: How can I integrate Request.js with Rails?
A: To integrate Request.js with Rails, you need to follow a step-by-step process. First, ensure that you have Request.js installed in your Rails application. Then, configure Request.js to work seamlessly with Rails by setting up the appropriate routes and controllers. Finally, you can start making HTTP requests using the Request.js library within your Rails codebase.
Q: What are the benefits of using Request.js with Rails?
A: There are several advantages to integrating Request.js with Rails. Firstly, it simplifies the process of making HTTP requests from client-side JavaScript code. Additionally, Request.js enhances the functionality and performance of your web applications by providing efficient and effective ways to handle responses and parse JSON data.
Q: Can I use other HTTP request libraries instead of Request.js?
A: Yes, while Request.js is a popular choice for making HTTP requests in Rails, there are other libraries available as well. In this article, we compare Request.js with other popular HTTP request libraries, providing code examples and discussing the advantages and disadvantages of each.
Q: How can I handle responses in Request.js?
A: Handling responses in Request.js is crucial when making HTTP requests. In this article, we cover various aspects of response handling, including error handling, status codes, and parsing JSON responses to extract necessary data. We provide insights and examples to help you handle responses effectively in your Rails application.
Q: What are some tips and best practices for integrating Request.js with Rails?
A: To ensure a smooth integration of Request.js with Rails, it’s important to follow certain best practices. In this article, we provide tips and recommendations for optimizing your code, enhancing security, and improving the overall performance of your Rails application when using Request.js. Following these best practices will help you maximize the benefits of Request.js in your projects.
Q: What are some common issues when using Request.js with Rails?
A: Despite our best efforts, issues may arise during the integration and usage of Request.js with Rails. In this article, we address common problems that developers may face and provide potential solutions and troubleshooting tips. If you encounter any issues, refer to this section for guidance on resolving them.
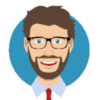
Christopher is a distinguished technical author renowned for his expertise in scientific computer science. With an illustrious career as a development manager at Cisco, Sun, and Twitter, he has consistently demonstrated exceptional leadership and technical acumen in delivering successful projects.
Christopher’s technical writing reflects his extensive knowledge and practical experience in scientific computer science. His ability to effectively communicate complex concepts to diverse audiences has earned him recognition within the scientific community. Through his publications, Christopher aims to share his insights, foster collaboration, and contribute to the advancement of the field.
As a technical author, Christopher remains committed to staying at the forefront of emerging technologies and driving innovation in scientific computer science. His expertise in Javascript development, coupled with his experience as a development manager, positions him as a valuable resource for professionals seeking guidance and best practices. With each publication, Christopher strives to empower readers, inspire creativity, and push the boundaries of scientific computer science.